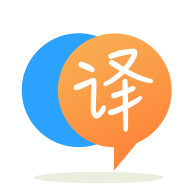
[英]Rubocop: Assignment Branch Condition size for method is too high. How can I reduce the method?
[英]Metrics/AbcSize: Assignment Branch Condition size for fill_arrays is too high. [<9, 21, 0> 22.85/17]
我需要用 ffaker 生成隨機數據並且不知道如何在這里修復 abcsize
這是我所擁有的
class Library
attr_accessor :authors, :books, :orders, :readers
def initialize(authors = [], books = [], orders = [], readers = [])
@books = books
@orders = orders
@readers = readers
@authors = authors
end
def create_data
@authors = []
@books = []
@readers = []
@orders = []
100.times do
authorname = FFaker::Book.author
biography = FFaker::Book.description
title = FFaker::Book.title
name = FFaker::Name.name
email = FFaker::Internet.email
city = FFaker::Address.city
street = FFaker::Address.street_name
house = rand(1 - 10_000)
date = Time.now.utc.strftime('%d.%m.%Y')
@authors.push(Author.new(authorname, biography))
@books.push(Book.new(title, authorname))
@readers.push(Reader.new(name, email, city, street, house))
@orders.push(Order.new(title, name, date))
end
end
end
所以我決定創建一些私有方法但仍然有很大的 abcsize
class Library
attr_accessor :authors, :books, :orders, :readers
def initialize(authors = [], books = [], orders = [], readers = [])
@books = books
@orders = orders
@readers = readers
@authors = authors
end
def create_data
create_arrays
fill_arrays
end
private
def create_arrays
@authors = []
@books = []
@readers = []
@orders = []
end
def fill_arrays
100.times do
authorname = FFaker::Book.author
biography = FFaker::Book.description
title = FFaker::Book.title
name = FFaker::Name.name
email = FFaker::Internet.email
city = FFaker::Address.city
street = FFaker::Address.street_name
house = rand(1 - 10_000)
date = Time.now.utc.strftime('%d.%m.%Y')
@authors.push(Author.new(authorname, biography))
@books.push(Book.new(title, authorname))
@readers.push(Reader.new(name, email, city, street, house))
@orders.push(Order.new(title, name, date))
end
end
end
我無法真正將那個 ffaker 部分分開,因為:
如果有人可以幫助我,我將不勝感激,在重構方面沒有太多經驗
方法分離部分按照@Sergii-k 的建議工作,但現在我在關聯方面遇到了一些麻煩
class Library
attr_accessor :authors, :books, :orders, :readers
def initialize(authors: [], books: [], orders: [], readers: [])
@books = books
@orders = orders
@readers = readers
@authors = authors
end
def create_arrays
@authors = []
@books = []
@readers = []
@orders = []
end
def create_data
create_arrays
fill_arrays
end
def show
create_data
puts @authors
puts @books
puts @orders
puts @readers
end
def build_author
name = FFaker::Book.author
biography = FFaker::Book.description
Author.new(name, biography)
end
def build_book(author)
title = FFaker::Book.title
Book.new(title, author.name)
end
def build_reader
name = FFaker::Name.name
email = FFaker::Internet.email
city = FFaker::Address.city
street = FFaker::Address.street_name
house = rand(1 - 10_000)
Reader.new(name, email, city, street, house)
end
def build_order(reader)
date = Time.now.utc.strftime('%d.%m.%Y')
title = FFaker::Book.title
Order.new(title, reader.name, date)
end
def fill_arrays
1.times do
author = build_author
reader = build_reader
@authors.push(author)
@books.push(build_book(author))
@readers.push(reader)
@orders.push(build_order(reader))
end
end
end
這是現在的問題部分
def build_book(author)
title = FFaker::Book.title
Book.new(title, author.name)
end
def build_order(reader)
date = Time.now.utc.strftime('%d.%m.%Y')
title = FFaker::Book.title
Order.new(title, reader.name, date)
end
我現在得到了 2 個不同的標題,因為我在這兩種方法中都生成了它們。 試圖通過 arguments 但它沒有用
def build_order(book, reader)
date = Time.now.utc.strftime('%d.%m.%Y')
Order.new(book.title, reader.name, date)
end
完成,效果很好!
class Library
attr_accessor :authors, :books, :orders, :readers
def initialize(authors: [], books: [], orders: [], readers: [])
@books = books
@orders = orders
@readers = readers
@authors = authors
end
def create_arrays
@authors = []
@books = []
@readers = []
@orders = []
end
def create_data
create_arrays
fill_arrays
end
def show
create_data
puts @authors
puts @books
puts @orders
puts @readers
end
def build_author
name = FFaker::Book.author
biography = FFaker::Book.description
Author.new(name, biography)
end
def build_book(author)
title = FFaker::Book.title
Book.new(title, author.name)
end
def build_reader
name = FFaker::Name.name
email = FFaker::Internet.email
city = FFaker::Address.city
street = FFaker::Address.street_name
house = rand(1 - 10_000)
Reader.new(name, email, city, street, house)
end
def build_order(book, reader)
date = Time.now.utc.strftime('%d.%m.%Y')
Order.new(book.title, reader.name, date)
end
def fill_arrays
1.times do
author = build_author
reader = build_reader
book = build_book(author)
@authors.push(author)
@books.push(book)
@readers.push(reader)
@orders.push(build_order(book, reader))
end
end
end
這是來自 yml 文件的日志
--- !ruby/object:Library
books:
- !ruby/object:Book
title: Case of the Missing Hungry Imp
author: Twila Mante
orders:
- !ruby/object:Order
book: Case of the Missing Hungry Imp
reader: Monty Feeney
date: 19.03.2021
readers:
- !ruby/object:Reader
name: Monty Feeney
email: lamonica.friesen@williamson.com
city: Croninport
street: Pfeffer Neck
house: 3002
authors:
- !ruby/object:Author
name: Twila Mante
biography: Ut porro deserunt voluptatem velit. Atque dicta labore ratione minima
sapiente. Dolor doloremque dolorem harum sint. At voluptatum molestias adipisci
vero. Perspiciatis rerum nesciunt maiores vitae.
您可以通過方法分離進一步 go :
def build_author
# ...
Author.new(authorname, biography)
end
def build_book(author)
# ...
Book.new(title, author.authorname)
end
def build_reader
# ...
Reader.new(name, email, city, street, house)
end
def build_order(book, reader)
# ...
Order.new(book.title, reader.name, date)
end
def fill_arrays
100.times do
author = build_author
reader = build_reader
book = build_book(author)
@authors.push(build_author)
@books.push(book)
@readers.push(reader)
@orders.push(build_order(book, reader))
end
end
更新:我注意到,一些參數是跨方法共享的。 您可以將它們作為 arguments 傳遞。
更新:固定書名。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.