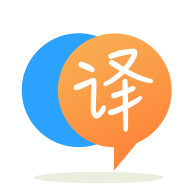
[英]Anagram of 2 string : I don't understand what is the problem with my code
[英]I don't understand what is wrong with my code (pointers and template)
#include <iostream>
#include <stdio.h>
#include <float.h>
using namespace std;
template<class T>
void getMinMax(T tab[], int nbPers, float *min, float *max){
*min = FLT_MAX;
*max = 0;
for (int i = 0; i < nbPers; i++){
if (tab[i] < *min){
*min = tab[i];
}
if (tab[i] > *max){
*max = tab[i];
}
}
printf("min : %.2f , max : %.2f", *min, *max);
}
int main()
{
int age[] = { 27, 35, 41, 18, 25, 30, 54, 50 };
float taille[] = { 1.72 , 1.65, 1.58, 1.76, 1.69, 1.54, 1.83, 1.71 },
poids[] = { 65.3, 67.1, 74.8, 60.7, 72.3, 56.4, 66.9, 72.1};
int nbPers = sizeof(age) / sizeof(int);
float *min;
float *max;
printf ("3. valeurs min et max avec pointeurs\n");
getMinMax(age, nbPers, &min, &max);
return 0;
}
我的目標是使用指針從 3 個 arrays 中獲取最小值和最大值,總共 6 個值。 當我嘗試編譯時,出現此錯誤:
error: no matching function for call to 'getMinMax(int [8], int&, float**, float**)
和這些注釋:
note: candidate: 'template<class T> void getMinMax(T*, int, float*, float*)
note: template argument deduction/substitution failed:
note: cannot convert '& min' (type 'float**') to type 'float*'
我找不到這些是什么意思,我相信我的模板會導致問題
這里有兩個問題:
#include <iostream>
#include <stdio.h>
#include <float.h>
using namespace std;
template<class T>
void getMinMax(T tab[], int nbPers, float *min, float *max){
*min = FLT_MAX;
*max = 0;
for (int i = 0; i < nbPers; i++){
if (tab[i] < *min){
*min = tab[i];
}
if (tab[i] > *max){
*max = tab[i];
}
}
printf("min : %.2f , max : %.2f", *min, *max);
}
int main()
{
int age[] = { 27, 35, 41, 18, 25, 30, 54, 50 };
float taille[] = { 1.72 , 1.65, 1.58, 1.76, 1.69, 1.54, 1.83, 1.71 },
poids[] = { 65.3, 67.1, 74.8, 60.7, 72.3, 56.4, 66.9, 72.1};
int nbPers = sizeof(age) / sizeof(int);
float *min; //PROBLEM # 1
float *max; //PROBLEM # 1
printf ("3. valeurs min et max avec pointeurs\n");
getMinMax(age, nbPers, &min, &max); //PROBLEM # 2
return 0;
}
問題#1:您使用的是未初始化的float
指針min
和max
。 您需要像這樣初始化它們,然后delete
它們(以免 memory 泄漏)它們:
int main()
{
int age[] = { 27, 35, 41, 18, 25, 30, 54, 50 };
float taille[] = { 1.72 , 1.65, 1.58, 1.76, 1.69, 1.54, 1.83, 1.71 },
poids[] = { 65.3, 67.1, 74.8, 60.7, 72.3, 56.4, 66.9, 72.1 };
int nbPers = sizeof(age) / sizeof(int);
float* min = new float; //initialized!
float* max = new float; //initialized!
printf("3. valeurs min et max avec pointeurs\n");
getMinMax(age, nbPers, &min, &max); //still a problem here
delete min; //no memory leak!
delete max; //no memory leak!
return 0;
}
問題 #2: min
和max
已經是float*
。 在它們上使用&
將給出一個float**
。 要解決此問題,只需執行以下操作:
int main()
{
int age[] = { 27, 35, 41, 18, 25, 30, 54, 50 };
float taille[] = { 1.72 , 1.65, 1.58, 1.76, 1.69, 1.54, 1.83, 1.71 },
poids[] = { 65.3, 67.1, 74.8, 60.7, 72.3, 56.4, 66.9, 72.1 };
int nbPers = sizeof(age) / sizeof(int);
float* min = new float;
float* max = new float;
printf("3. valeurs min et max avec pointeurs\n");
getMinMax(age, nbPers, min, max); // note I am not using &
delete min;
delete max;
return 0;
}
此外,要調試模板,最好刪除模板,並將所有泛型類型替換為固定類型,例如int
。
錯誤轉錄:
error: no matching function for call to 'getMinMax(int [8], int&, float**, float**) //See Problem # 2.
note: candidate: 'template void getMinMax(T*, int, float*, float*) //getMinMax is a possible function to call, but because of the float**s instead of float*s, as said in Problem # 2, it is not called
note: template argument deduction/substitution failed:
note: cannot convert '& min' (type 'float**') to type 'float*' //see Problem # 2
調試模板內容時的一個有用提示:擺脫模板,在這種情況下。 將 getMinMax 重寫為普通的舊 function,如果它有效。 那么您知道問題是模板特有的問題,如果不是。 那么你犯了一個與模板無關的錯誤,事實上,除非你正在編寫的模板非常簡單和/或你對這些東西非常有經驗,否則你應該先把它寫成一個普通的 function。 並且只有在它已經工作時才轉換為模板。
使這變得容易的標准“技巧”是使用 typedef。 也就是說,不是從template<class T>
開始,然后是 function 的 rest,而是從typedef float T;
然后是 function 的 rest。 然后編譯器會將 T 視為“float”的另一個名稱。 或者你可以使用比 T 更具體的名稱,例如typedef float Number;
如果您打算將 function 用於數字。
我建議您在 getMinMax 上嘗試這個技巧:注釋掉模板行並放入 ttpedef 行。 您的代碼仍然無法編譯,但編譯器錯誤消息通常更容易理解。
我已經在你的代碼中看到了至少一個錯誤,但我沒有指出它,而是決定分享這種技術,我認為它可以讓你很容易地找到問題。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.