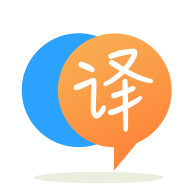
[英]How to move the pictures in all subfolders to another new same folder in Python?
[英]Powershell move all download to another folder function
我想創建一個 function 將文件移動到一個文件夾,具體取決於文件“C:\Users\dgoud\Desktop\TP4\Telechargement”到特定文件夾的類型
我想為 PowerShell 提供等價物:
for root, dirs, files in os.walk("C:\Users\dgoud\Desktop\TP4\Telechargement"):
我的 PowerShell function:
function DeplacerDansBonDossier {
param (
$extension
)
foreach ($file in $files) {
$extn = [IO.Path]::GetExtension($line)
if ($extn -eq ".txt" )
{
Move-Item -Path "C:\Users\dgoud\Desktop\TP4\Documents"
}
elseif ($extn -eq ".mp3" )
{
Move-Item -Path "C:\Users\dgoud\Desktop\TP4\Musique"
}
elseif ($extn -eq ".wav" )
{
Move-Item -Path "C:\Users\dgoud\Desktop\TP4\Musique"
elseif ($extn -eq ".mp4" )
{
Move-Item -Path "C:\Users\dgoud\Desktop\TP4\VidBo"
}
elseif ($extn -eq ".mkv" )
{
Move-Item -Path "C:\Users\dgoud\Desktop\TP4\VidBo"
}
}
}
}
我想這就是你要找的。 這個 function 將只接受文件作為輸入,請注意我在下面使用Get-ChildItem -File
。 要考慮的另一點是,如果目標文件夾Move-Item
上有同名File
,則會拋出以下錯誤:
Move-Item: Cannot create a file when that file already exists.
如果要替換現有文件,則可以使用-Force
,如果存在同名的現有文件,則可以在其中添加新條件。
function DeplacerDansBonDossier {
[cmdletbinding()]
param(
[parameter(mandatory,valuefrompipeline)]
[System.IO.FileInfo]$File
)
begin
{
$Paths = @{
Documents = "C:\Users\dgoud\Desktop\TP4\Documents"
Music = "C:\Users\dgoud\Desktop\TP4\Musique"
Video = "C:\Users\dgoud\Desktop\TP4\VidBo"
}
}
process
{
switch -Regex($File.Extension)
{
'^\.txt$'{
$File | Move-Item -Destination $Paths['Documents']
Write-Verbose ('Moved {0} to {1}' -f $file.Name,$Paths['Documents'])
break
}
'^\.mp3$|^\.wav$'{
$File | Move-Item -Destination $Paths['Music']
Write-Verbose ('Moved {0} to {1}' -f $file.Name,$Paths['Music'])
break
}
'^\.mp4$|^\.mkv$'{
$File | Move-Item -Destination $Paths['Video']
Write-Verbose ('Moved {0} to {1}' -f $file.Name,$Paths['Video'])
break
}
}
}
}
function的使用方法:
Get-ChildItem 'C:\Users\dgoud\Desktop\TP4\Telechargement' -File | DeplacerDansBonDossier
# If you want to see which Files are being moved and their destination
# you can use -Verbose
Get-ChildItem 'C:\Users\dgoud\Desktop\TP4\Telechargement' -File | DeplacerDansBonDossier -Verbose
不用說,有很多方法可以做到這一點。 您可能根本不需要 function,因為這些文件可以直接通過管道傳輸到ForEach-Object
循環。 無論如何,如果我們使用 function,我會首先將Get-ChildItem
生成的[FileInfo]
對象的集合發送到 function。 這是代替擴展名。
這可能看起來像這樣:
Function DeplacerDansBonDossier {
param (
$Files
)
foreach ( $file in $files ) {
$extn = $File.Extension
if ($extn -eq ".txt" ) {
Move-Item -Path $File -Destination "C:\Users\dgoud\Desktop\TP4\Documents"
}
elseif ($extn -eq ".mp3" ) {
Move-Item -Path $File -Destination "C:\Users\dgoud\Desktop\TP4\Musique"
}
elseif ($extn -eq ".wav" ) {
Move-Item -Path $File -Destination "C:\Users\dgoud\Desktop\TP4\Musique"
}
elseif ($extn -eq ".mp4" ) {
Move-Item -Path $File -Destination "C:\Users\dgoud\Desktop\TP4\VidBo"
}
elseif ($extn -eq ".mkv" ) {
Move-Item -Path $File -Destination "C:\Users\dgoud\Desktop\TP4\VidBo"
}
}
} # End Function DeplacerDansBonDossier
在您的演示代碼中,您有擴展名,但您手頭沒有 object 文件,因此實際上沒有什么可移動的。
@AbrahamZinala 建議使用 switch 語句的另一個變體:
Function DeplacerDansBonDossier {
param (
$Files
)
foreach ($file in $files) {
$extn = $File.Extension
Switch ($extn)
{
'.txt' { Move-Item -Path $File -Destination "C:\Users\dgoud\Desktop\TP4\Documents"; Break }
'.mp3' { Move-Item -Path $File -Destination "C:\Users\dgoud\Desktop\TP4\Musique"; Break }
'.wav' { Move-Item -Path $File -Destination "C:\Users\dgoud\Desktop\TP4\Musique"; Break }
'.mp4' { Move-Item -Path $File -Destination "C:\Users\dgoud\Desktop\TP4\VidBo"; Break }
'.mkv' { Move-Item -Path $File -Destination "C:\Users\dgoud\Desktop\TP4\VidBo"; Break }
}
}
} # End Function DeplacerDansBonDossier
$Files = Get-ChildItem -Path C:\temp\SourceFolder
DeplacerDansBonDossier $Files
顯然Switch
使事情更簡潔,但沒有描述性。 另外,請注意break
語句。 與If\ElseIf
不同, Switch
語句將評估多個條件和腳本塊。 我們用Break
停止這種行為
不是多余的,但這里可以做的還有很多。 例如,我們可以通過管道啟用 function 以便它可以在Get-ChildItem
之后立即進行管道傳輸,但考慮到上述ForEach-Object
的潛力將運行大致相同,這可能不值得。
ForEach-Object
示例:
Get-ChildItem -Path C:\temp\SourceFolder |
ForEach-Object{
foreach ( $file in $files ) {
$extn = $File.Extension
if ($extn -eq ".txt" ) {
Move-Item -Path $_ -Destination "C:\Users\dgoud\Desktop\TP4\Documents"
}
elseif ($extn -eq ".mp3" ) {
Move-Item -Path $_ -Destination "C:\Users\dgoud\Desktop\TP4\Musique"
}
elseif ($extn -eq ".wav" ) {
Move-Item -Path $_ -Destination "C:\Users\dgoud\Desktop\TP4\Musique"
}
elseif ($extn -eq ".mp4" ) {
Move-Item -Path $_ -Destination "C:\Users\dgoud\Desktop\TP4\VidBo"
}
elseif ($extn -eq ".mkv" ) {
Move-Item -Path $_ -Destination "C:\Users\dgoud\Desktop\TP4\VidBo"
}
}
}
注意:我不會在ForEach-Object
腳本塊中使用Switch
。 $_
在Switch
上下文中的操作方式不同。
注意:這些都沒有經過測試。 您可能需要為-Path
參數使用.FullName
屬性,即$File.FullName
或$_.FullName
。 *-Item
cmdlet 在這種情況下可能會很麻煩。
已經添加到其他很好的答案,如果擴展總是 map 到這些路徑,則可以在此處使用將它們存儲在 hash 表中。
function DeplacerDansBonDossier {
[CmdletBinding()]
param (
[Parameter(Mandatory, ValueFromPipeline)]
[System.IO.FileInfo]
$File,
[Parameter(Mandatory = $false)]
[ValidateNotNullOrEmpty()]
[string]
$Username = $env:USERNAME
)
begin {
$extensionDestinationMappings = @{
".txt" = "C:\Users\$Username\Desktop\TP4\Documents"
".mp3" = "C:\Users\$Username\Desktop\TP4\Musique"
".wav" = "C:\Users\$Username\Desktop\TP4\Musique"
".mp4" = "C:\Users\$Username\Desktop\TP4\VidBo"
".mkv" = "C:\Users\$Username\Desktop\TP4\VidBo"
}
}
process {
$extension = $File.Extension
# Check extension exists in hashtable beforehand
if ($extensionDestinationMappings.ContainsKey($extension)) {
Move-Item -Path $File.FullName -Destination $extensionDestinationMappings[$extension]
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.