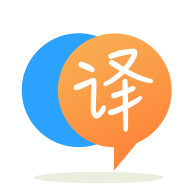
[英]Facing issues in Creating Asp.net Web Api in C# with details below:
[英]Creating ASP.Net MVC Web Application in C# with details below:
如何保存用戶上次登錄的歷史記錄並在用戶登錄后立即顯示。 (例如;LastLogin:2021 年 5 月 31 日星期一)
我對如何顯示它感到困惑的一件事,我在這里分享我的詳細信息,任何幫助將不勝感激。
Controller 登錄碼
public ActionResult Login()
{
return View();
}
[HttpPost]
public ActionResult Login(LoginViewModel login)
{
if (ModelState.IsValid)
{
if (new UserEntity().IsValidUser(login.EmailId, login.Password))
{
/*Very Much important line of code, now we can use this session
variable in Emloyee control and only valid user can access employee
data otherwise we will redirect the user to login page in case of null
session */
Session["login"] = login;
//Redirect to Employee Controller after Validation
return RedirectToAction("Index", "Employee");
}
else
{
ViewBag.InvalidUser = "Invalid User Name or Password";
return View(login);
}
}
return View(login);
}
public ActionResult Logout()
{
Session["login"] = null;
Session.Abandon();
return RedirectToAction("Login");
}
LoginController
中使用的LoginViewModel
:
using System;
using System.Collections.Generic;
using System.ComponentModel.DataAnnotations;
using System.Linq;
using System.Web;
namespace Project_Login.Models
{
public class LoginViewModel
{
[Display(Name = "Email Address")]
[Required]
public string EmailId { get; set; }
[Display(Name = "Password")]
[Required]
[DataType(DataType.Password)]
public string Password { get; set; }
}
}
驗證用戶(類):
public Boolean IsValidUser(string emailId, string password)
{
Boolean isValid = false;
try
{
string ConnectionString = ConfigurationManager.ConnectionStrings["myConnectionString"].ConnectionString;
sqlConnection = new SqlConnection(ConnectionString);
string query = @"Select * from UserProfile where EmailID='" + emailId + "' and Password = '" + password + "'";
cmd = new SqlCommand(query, sqlConnection);
sqlConnection.Open();
SqlDataReader dataReader = cmd.ExecuteReader();
if (dataReader.Read())
{
isValid = true;
}
}
catch (Exception exp)
{
//exception logging
}
return isValid;
}
登錄視圖:
@model Project_Login.Models.LoginViewModel
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Login</title>
<link href="~/Content/bootstrap.min.css" rel="stylesheet" />
</head>
<body>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<h4>Login</h4>
<hr />
@if (ViewBag.InvalidUser != null)
{
<p class="alert-danger"> @ViewBag.InvalidUser </p>
}
<div class="form-group">
@Html.LabelFor(model => model.EmailId, htmlAttributes:
new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.EmailId, new {
htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model => model.EmailId,
"", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
@Html.LabelFor(model => model.Password,
htmlAttributes: new { @class = "control-label col-md-2" })
<div class="col-md-10">
@Html.EditorFor(model => model.Password, new {
htmlAttributes = new { @class = "form-control" } })
@Html.ValidationMessageFor(model =>
model.Password, "", new { @class = "text-danger" })
</div>
</div>
<div class="form-group">
<div class="col-md-offset-2 col-md-10">
<input type="submit" value="Login" class="btn btndefault" />
</div>
</div>
</div>
}
<div>
@Html.ActionLink("Not Registered? Click to Signup", "Signup")
</div>
</body>
</html>
數據庫( UserProfile
表):
您可以嘗試使用 HttpCookie 來存儲用戶上次登錄信息。
這是一個您可以參考的代碼示例:
public ActionResult Login()
{
var username = Request.Cookies["UserName"] == null ? "" : Request.Cookies["UserName"].Value.ToString();
var time = Request.Cookies["Time"] == null ? "" : Request.Cookies["Time"].Value.ToString();
string message = string.Format("The Last login user is {0} and time is {1}", username, time);
Response.Write(message);
return View();
}
[HttpPost]
public ActionResult Login(LoginViewModel login)
{
if (ModelState.IsValid)
{
if (IsValidUser(login.EmailId, login.Password))
{
/*Very Much important line of code, now we can use this session
variable in Emloyee control and only valid user can access employee
data otherwise we will redirect the user to login page in case of null
session */
//Session["login"] = login;
HttpCookie cookie1 = new HttpCookie("UserName");
cookie1.Value = login.EmailId;
Response.AppendCookie(cookie1);
HttpCookie cookie2 = new HttpCookie("Time");
cookie2.Value = DateTime.Now.ToString();
Response.AppendCookie(cookie2);
ViewBag.InvalidUser = "Correct User Name or Password";
string message = string.Format("The Last login user is {0} and time is {1}", cookie1.Value, cookie2.Value);
Response.Write(message);
}
else
{
ViewBag.InvalidUser = "Invalid User Name or Password";
return View(login);
}
}
return View(login);
}
結果:
首先,我建議使用 ASP.NET Identity ( https://docs.microsoft.com/en-us/aspnet/core/security/authentication/identity?view=aspnetcore-5.0&tabs=visual-studio )默認情況下,讓您的身份驗證安全(永遠不要以明文形式存儲您的密碼,請使用參數化查詢使您的 SQL 不易被注入,您的代碼會同時受到這兩種情況的影響。)。
要回答您的問題:您應該創建一個捕獲上次登錄的數據庫屬性,在用戶登錄時更新該行(使用當前日期和時間),然后將該屬性返回給您的 controller。 然后您的 controller 可以在您的視圖中設置數據,並在您的視圖中顯示屬性。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.