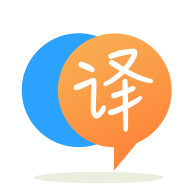
[英]How to serialize the DateTime field with DateTimeKind option through ProtoBuf-net
[英]Using `serde::Serialize` with `Option<chrono::DateTime>`
嘗試序列化Option<chrono::DateTime<Utc>>
時遇到錯誤:
error[E0308]: mismatched types
--> src/main.rs:39:14
|
39 | #[derive(Serialize, Debug)]
| ^^^^^^^^^ expected struct `DateTime`, found enum `std::option::Option`
|
= note: expected reference `&DateTime<Utc>`
found reference `&'__a std::option::Option<DateTime<Utc>>`
= note: this error originates in a derive macro (in Nightly builds, run with -Z macro-backtrace for more info)
代碼( 游樂場):
use chrono::{serde::ts_seconds, DateTime, NaiveDate, Utc};
use serde::Serialize;
fn main() {
let test_struct = TestStruct {
a: 2.45,
date: Some(DateTime::from_utc(
NaiveDate::from_ymd(2000, 1, 1).and_hms(1, 1, 1),
Utc,
)),
};
let string = serde_json::to_string(&test_struct).unwrap();
println!("default: {}", string);
#[derive(Serialize, Debug)]
struct TestStruct {
pub a: f32,
#[serde(with = "ts_seconds")]
pub date: Option<DateTime<Utc>>,
}
}
看着chrono::ts_seconds
和serde_with我不知道該往哪里前進。
我真的很感激任何幫助。
Chrono 已經有一個 function 用於Option<DateTime<Utc>>
,即chrono::serde::ts_seconds_option
。
#[derive(Serialize, Debug)]
struct TestStruct {
pub a: f32,
#[serde(with = "ts_seconds_option")]
pub date: Option<DateTime<Utc>>,
}
使用serde_with
的解決方案如下所示:
#[serde_as]
#[derive(Serialize, Debug)]
struct TestStruct {
pub a: f32,
#[serde_as(as = "Option<DurationSeconds<i64>>")]
pub date: Option<DateTime<Utc>>,
}
您可以編寫自己的包裝器並將其與serialize_with
和skip_serializing_if
結合使用:
pub fn serialize_dt<S>(
dt: &Option<DateTime<Utc>>,
serializer: S
) -> Result<S::Ok, S::Error>
where
S: Serializer {
match dt {
Some(dt) => ts_seconds::serialize(dt, serializer),
_ => unreachable!(),
}
}
#[derive(Serialize, Debug)]
struct TestStruct {
pub a: f32,
#[serde(serialize_with = "serialize_dt", skip_serializing_if = "Option::is_none")]
pub date: Option<DateTime<Utc>>,
}
當您想使用DateTime<Utc>
而不是 Unix 時間戳時。
const FORMAT: &str = "%Y-%m-%d %H:%M:%S";
pub fn serialize<S>(date: &Option<DateTime<Utc>>, serializer: S) -> Result<S::Ok, S::Error>
where
S: Serializer,
{
match date.is_some() {
true => {
let s = format!("{}", date.as_ref().unwrap().format(FORMAT));
serializer.serialize_str(&s)
}
false => serializer.serialize_none(),
}
}
"{\"a\":2.45,\"date\":\"2022-12-16 16:40:36\"}"
TestStruct { a: 2.45, date: Some(2022-12-16T16:40:36Z) }
"{\"a\":2.45,\"date\":null}"
TestStruct { a: 2.45, date: None }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.