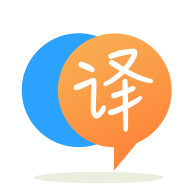
[英]Unable to create an object of type 'ApplicationDbContext' in asp .net core 5
[英]Unable to create an object of type 'ApplicationDbContext'. Ef core 5.0
我使用 CleanArchitecture 解決方案。 我有 ApplicationDbContext 和 UnitOfWork 所在的數據層:
namespace Portal.Data.MyDbContexts
{
internal class ApplicationDbContext : DbContext
{
public ApplicationDbContext(DbContextOptions<ApplicationDbContext> options) : base(options)
{
Database.EnsureCreated();
}
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
base.OnModelCreating(modelBuilder);
modelBuilder.ApplyConfigurationsFromAssembly(typeof(BaseEntity).Assembly);
}
// **********
public DbSet<WebsiteMonitoredCategory> WebsiteMonitoredCategories { get; set; }
// **********
}
工作單元:
public abstract class BaseUnitOfWork : object, IBaseUnitOfWork
{
//public UnitOfWork() : base()
//{
//}
public BaseUnitOfWork(Options options) : base()
{
Options = options;
}
// **********
protected Options Options { get; set; }
// **********
// **********
// **********
// **********
private ApplicationDbContext _databaseContext;
// **********
// **********
/// <summary>
/// Lazy Loading = Lazy Initialization
/// </summary>
internal ApplicationDbContext DatabaseContext
{
get
{
if (_databaseContext == null)
{
var optionsBuilder =
new DbContextOptionsBuilder<ApplicationDbContext>();
switch (Options.Provider)
{
case Provider.SqlServer:
{
optionsBuilder.UseSqlServer
(connectionString: Options.ConnectionString);
break;
}
case Provider.MySql:
{
//optionsBuilder.UseMySql
// (connectionString: Options.ConnectionString);
break;
}
case Provider.Oracle:
{
//optionsBuilder.UseOracle
// (connectionString: Options.ConnectionString);
break;
}
case Provider.PostgreSQL:
{
//optionsBuilder.UsePostgreSQL
// (connectionString: Options.ConnectionString);
break;
}
case Provider.InMemory:
{
optionsBuilder.UseInMemoryDatabase(databaseName: "Temp");
break;
}
default:
{
break;
}
}
_databaseContext =
new ApplicationDbContext(options: optionsBuilder.Options);
}
return _databaseContext;
}
}
我也有 IoC 層,用於向表示層注入依賴項:
public class DependencyContainer
{
public static void RegisterServices(IServiceCollection services,IConfiguration configuration)
{
//DataLayer
services.AddTransient<IUnitOfWork, UnitOfWork>(_ =>
{
Options options =
new Options
{
Provider =
(Provider)System.Convert.ToInt32(configuration.GetSection(key: "DatabaseProvider").Value),
ConnectionString =
configuration.GetSection(key: "ConnectionStrings").GetSection(key: "MyConnectionString").Value,
};
return new UnitOfWork(options: options);
});
}
}
最后我有一個 Windows Forms 應用程序 Net 5.0(所有項目都是 Net5.0)
namespace Portal.Desktop
{
public static class Program
{
private static IConfiguration Configuration { get; set; }
public static IServiceProvider ServiceProvider { get; set; }
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
var services = ConfigureServices();
var builder = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json", optional: true, reloadOnChange: true);
Configuration = builder.Build();
Application.SetHighDpiMode(HighDpiMode.SystemAware);
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
RegisterServices(services, Configuration);
}
public static void RegisterServices(IServiceCollection services, IConfiguration configuration)
{
DependencyContainer.RegisterServices(services, configuration);
}
public static IServiceCollection ConfigureServices()
{
var services = new ServiceCollection();
//services.AddTransient<IUnitOfWork, UnitOfWork>(_ =>
//{
// Options options =
// new Options
// {
// Provider =
// (Provider)System.Convert.ToInt32(Configuration.GetSection(key: "DatabaseProvider").Value),
// //using Microsoft.EntityFrameworkCore;
// //ConnectionString =
// // Configuration.GetConnectionString().GetSection(key: "MyConnectionString").Value,
// ConnectionString =
// Configuration.GetSection(key: "ConnectionStrings").GetSection(key: "MyConnectionString").Value,
// };
// return new Portal.Data.UoW.UnitOfWork(options: options);
//});
ServiceProvider = services.BuildServiceProvider();
return services;
}
}
}
我使用 Ef 核心 5.0、windows forms 設置為啟動項目,package 管理器控制台設置為 Protal.Data,但在 PMC 中運行時得到一個錯誤( dbcontext和 unitofwork 運行時)
無法創建“ApplicationDbContext”類型的 object。 有關設計時支持的不同模式,請參閱https://go.microsoft.com/fwlink/?linkid=851728
在數據層安裝了這個包:
<ItemGroup>
<PackageReference Include="Microsoft.EntityFrameworkCore" Version="5.0.7" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Design" Version="5.0.7">
<IncludeAssets>runtime; build; native; contentfiles; analyzers; buildtransitive</IncludeAssets>
</PackageReference>
<PackageReference Include="Microsoft.EntityFrameworkCore.InMemory" Version="5.0.7" />
<PackageReference Include="Microsoft.EntityFrameworkCore.SqlServer" Version="5.0.7" />
<PackageReference Include="Microsoft.EntityFrameworkCore.Tools" Version="5.0.7">
<IncludeAssets>runtime; build; native; contentfiles; analyzers; buildtransitive</IncludeAssets>
</PackageReference>
</ItemGroup>
不知道為什么,給我一個錯誤。
- - -更新 - -
我遵循https://go.microsoft.com/fwlink/?linkid=851728並將ApplicationDbContextFactory.cs添加到 Portal.Desktop (windows forms Net5.0) 項目:
namespace Portal.Desktop
{
public class ApplicationDbContextFactory:IDesignTimeDbContextFactory<ApplicationDbContext>
{
public ApplicationDbContext CreateDbContext(string[] args)
{
var connectionString = Program.Configuration.GetSection(key: "ConnectionStrings").GetSection(key: "MyConnectionString").Value;
var provider = Program.Configuration.GetSection(key: "DatabaseProvider").Value;
var optionsBuilder =
new DbContextOptionsBuilder<ApplicationDbContext>();
var options =
new Options
{
Provider =
(Provider)System.Convert.ToInt32(provider),
//using Microsoft.EntityFrameworkCore;
//ConnectionString =
// Configuration.GetConnectionString().GetSection(key: "MyConnectionString").Value,
ConnectionString = connectionString,
};
switch (options.Provider)
{
case Provider.SqlServer:
{
optionsBuilder.UseSqlServer
(connectionString: options.ConnectionString);
break;
}
case Provider.MySql:
{
//optionsBuilder.UseMySql
// (connectionString: Options.ConnectionString);
break;
}
case Provider.Oracle:
{
//optionsBuilder.UseOracle
// (connectionString: Options.ConnectionString);
break;
}
case Provider.PostgreSQL:
{
//optionsBuilder.UsePostgreSQL
// (connectionString: Options.ConnectionString);
break;
}
case Provider.InMemory:
{
optionsBuilder.UseInMemoryDatabase(databaseName: "Temp");
break;
}
default:
{
break;
}
}
return
new ApplicationDbContext(options: optionsBuilder.Options);
}
}
}
當我想添加遷移時,核心拋出異常已被調用的目標拋出。 例外:
System.Reflection.TargetInvocationException:調用的目標已引發異常。 ---> System.NullReferenceException:Object 引用未設置為 object 的實例。 在 C 中的 Portal.Desktop.ApplicationDbContextFactory.CreateDbContext(String[] args):\Users\Arman Es\source\repos\Spider\Portal.Desktop\ApplicationDbContextFactory.cs:line 20 --- 內部異常堆棧跟蹤結束 -- - at System.RuntimeMethodHandle.InvokeMethod(Object target, Object[] arguments, Signature sig, Boolean constructor, Boolean wrapExceptions) at System.Reflection.RuntimeMethodInfo.Invoke(Object obj, BindingFlags invokeAttr, Binder binder, Object[] parameters, CultureInfo culture )
在 System.Reflection.MethodBase.Invoke(Object obj, Object[] parameters) 在 Microsoft.EntityFrameworkCore.Design.Internal.DbContextOperations.CreateContextFromFactory(Type factory, Type contextType) 在 Microsoft.EntityFrameworkCore.Design.Internal.DbContextOperations.<>c__DisplayClass13_2 .b__9() 在 Microsoft.EntityFrameworkCore.Design.Internal.DbContextOperations.CreateContext(Func1 factory) at Microsoft.EntityFrameworkCore.Design.Internal.DbContextOperations.CreateContext(String contextType) at Microsoft.EntityFrameworkCore.Design.Internal.MigrationsOperations.AddMigration(String name, String outputDir, String contextType, String namespace) at Microsoft.EntityFrameworkCore.Design.OperationExecutor.AddMigrationImpl(String name, String outputDir, String contextType, String namespace) at Microsoft.EntityFrameworkCore.Design.OperationExecutor.AddMigration.<>c__DisplayClass0_0.<.ctor>b__0() at Microsoft.EntityFrameworkCore.Design.OperationExecutor.OperationBase.<>c__DisplayClass3_0
1.b__0( ) 在 Microsoft.EntityFrameworkCore.Design.OperationExecutor.OperationBase.Execute(Action action) 調用的目標已引發異常。
最后,我在這篇文章https://snede.net/you-dont-need-a-idesigntimedbcontextfactory/中找到了我的答案
在 Portal.Data 項目中創建ApplicationDbContextFactory :
using System.IO;
using Microsoft.EntityFrameworkCore;
using Microsoft.EntityFrameworkCore.Design;
using Microsoft.Extensions.Configuration;
using Portal.Domain.Tools;
using Portal.Domain.Tools.Enums;
namespace Portal.Data.MyDbContexts
{
public class ApplicationDbContextFactory:IDesignTimeDbContextFactory<ApplicationDbContext>
{
private IConfiguration Configuration { get; set; }
public ApplicationDbContext CreateDbContext(string[] args)
{
var builder = new ConfigurationBuilder()
.SetBasePath(Directory.GetCurrentDirectory())
.AddJsonFile("appsettings.json");
Configuration = builder.Build();
var optionsBuilder =
new DbContextOptionsBuilder<ApplicationDbContext>();
var options =
new Options
{
Provider =
(Provider)System.Convert.ToInt32(Configuration.GetSection(key: "DatabaseProvider").Value),
ConnectionString = Configuration.GetSection(key: "ConnectionStrings").GetSection(key: "MyConnectionString").Value
};
switch (options.Provider)
{
case Provider.SqlServer:
{
optionsBuilder.UseSqlServer
(connectionString: options.ConnectionString);
break;
}
case Provider.MySql:
{
//optionsBuilder.UseMySql
// (connectionString: Options.ConnectionString);
break;
}
case Provider.Oracle:
{
//optionsBuilder.UseOracle
// (connectionString: Options.ConnectionString);
break;
}
case Provider.PostgreSQL:
{
//optionsBuilder.UsePostgreSQL
// (connectionString: Options.ConnectionString);
break;
}
case Provider.InMemory:
{
optionsBuilder.UseInMemoryDatabase(databaseName: "Temp");
break;
}
default:
{
break;
}
}
return
new ApplicationDbContext(options: optionsBuilder.Options);
}
}
}
appsettings.json仍在 Portal.Desktop 項目中:
namespace Portal.Desktop
{
public static class Program
{
public static IConfiguration Configuration { get; set; }
public static IServiceProvider ServiceProvider { get; set; }
/// <summary>
/// The main entry point for the application.
/// </summary>
[STAThread]
static void Main()
{
var services = ConfigureServices();
RegisterServices(services, Configuration);
Application.SetHighDpiMode(HighDpiMode.SystemAware);
Application.EnableVisualStyles();
Application.SetCompatibleTextRenderingDefault(false);
Application.Run(new MainForm());
}
public static void RegisterServices(IServiceCollection services, IConfiguration configuration)
{
DependencyContainer.RegisterServices(services, configuration);
}
public static IServiceCollection ConfigureServices()
{
var services = new ServiceCollection();
ServiceProvider = services.BuildServiceProvider();
return services;
}
}
}
最后IoC項目是:
namespace Portal.IoC
{
public class DependencyContainer
{
public static void RegisterServices(IServiceCollection services,IConfiguration configuration)
{
//DataLayer
services.AddTransient<IUnitOfWork, UnitOfWork>(_ =>
{
var options =
new Options
{
Provider =
(Provider)System.Convert.ToInt32(configuration.GetSection(key: "DatabaseProvider").Value),
ConnectionString =
configuration.GetSection(key: "ConnectionStrings").GetSection(key: "MyConnectionString").Value,
};
return new UnitOfWork(options: options);
});
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.