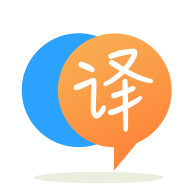
[英]I am currently writing code in node. It is showing error for specifying the path . what am i doing wrong?
[英]pruneRoots function returning undefined in the below tree algorithm when removing a node. Unable to make it work. What am I doing wrong?
我正在處理 Atlassian 的植物您的代碼挑戰。 我在 4.1 級。 我是新手。 我知道樹,關於這個問題,當在遞歸函數中將樹的節點作為參數發送時,它們由變量接收,而不是引用到原始樹。
遞歸后,我在最終返回值中不斷得到未定義。 我無法解決這個問題。 必須編輯原始樹,在這種情況下,修剪。
我被這個挑戰困了好幾天。 我已經嘗試過我閱讀過的方法,觀看了所有關於此的視頻,我即將在這個級別上放棄這個挑戰。 此后我只有 2 個級別,我想裝備它們進入。 我需要知道正確答案背后的原因以及我的代碼哪里出錯了。
這就是我一直在學習、參與挑戰和解決問題的方式。 對我來說,這個學習很重要。 我認真對待這件事。 我希望得到一個答案以加強我的理解。
你能幫我嗎?
謝謝你。
問:
編寫一個函數,在您修剪了所有在其下方沒有健康節點的不健康節點后,該函數將返回您的新根系統。
那里提供的提示:
二叉樹節點的定義:
function Node(data, left, right) { this.data = data === undefined ? 0 : data; this.left = left === undefined ? null : left; this.right = right === undefined ? null : right; }
完成以下確定要修剪的節點,以便修剪所有沒有健康節點作為子節點的不健康節點並返回結果。 將修剪的節點設置為空。
@param {Node} root - 代表植物根系的二叉樹數據
@return {Node} - 代表植物修剪根系的二叉樹數據
我的代碼:
function pruneRoots(root) {
const removeNode = function(node) {
if (node === null) {
return null;
}
if (node.data === 0) {
if(node.left && node.right){
if(node.left.data === 0 && node.right.data === 0)
{
return null;
}
}
if(node.right && node.right !== null){
node.right = removeNode(node.right);
}
if(node.left && node.left !== null){
node.left = removeNode(node.left);
}
} else if(node.data === 1){
if(node.right && node.right !== null){
node.right = removeNode(node.right);
}
if(node.left && node.left != null){
node.left = removeNode(node.left);
}}
return node
}
var a = removeNode(root);
console.log(a); //or return a
}
//function call, the root is structured as shown in the argument passed.
pruneRoots({
"data": 1,
"left": null,
"right": {
"data": 0,
"left": {
"data": 0,
"left": null,
"right": null
},
"right": {
"data": 0,
"left": null,
"right": null
}
}
});
您代碼中的注釋揭示了一些誤解。
例如,下面的注釋沒有描述下一行代碼在做什么:
// node has no children
if (node.left && node.right){
上面的if
條件實際上是在測試node
是否有兩個孩子! node.left
當它是一個對象(即一個節點)時是一個“真”值,當它為null
時是“假” null
。 因此,您正在測試node.left
和node.right
是否不為null
(因為這是它們在此上下文中可能具有的唯一可能的“假”值)。
以下評論並不總是正確的:節點可能只有一個右孩子,但沒有左孩子——一個if
條件可能為真,而另一個則不是。
// node has two children
if(node.right && node.right !== null) {
你可以改進的另一件事:
console.log(a) //since its on my code editor
//otherwise return a, for the main challenge page
你應該總是return
這里。 出於調試目的執行console.log
很好,但確保在測試時也return
它。
您的算法存在邏輯錯誤:
if (node.left && node.right){
if(node.left.data === 0 && node.right.data === 0) {
return null
}
}
這是不對的,因為node.left.left
仍然可以有一個值 1(即健康),所以返回null
是錯誤的。 這里的關鍵見解是,您應該首先對node.left
和node.right
進行遞歸調用,然后檢查這些節點是否因此被修剪。 如果是這樣,只有這樣您才能決定還修剪當前node
(並返回null
)。
您創建了一個嵌套函數來執行遞歸。 但與遞歸使用主pruneRoots
函數相比,它確實沒有任何優勢:它采用相同類型的參數並返回相同類型。 您不妨遞歸地使用pruneRoots
。
所以這是一個更正的版本:
function pruneRoots(root) { if (root === null) { return null; } // First perform the recursive pruning root.left = pruneRoots(root.left); root.right = pruneRoots(root.right); // Now we can be sure that if a child still exists, // there must be a healthy node in its subtree if (root.data === 0 && !root.left && !root.right) { // Not healthy and no children return null; } // In all other cases, don't prune the node return root; } let result = pruneRoots({ "data": 1, "left": null, "right": { "data": 0, "left": { "data": 0, "left": null, "right": null }, "right": { "data": 0, "left": null, "right": null } } }); console.log(result);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.