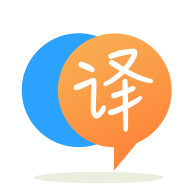
[英]C# Console App executable (.exe) does not save updated file to disk
[英]How to make a c# console app .exe to open a save dialog to save a file generated by the app?
我的代碼:
static void Main(string[] args)
{
string filePath = "./MOCK_DATA.json";
List<UserModel> datas = JsonConvert.DeserializeObject<List<UserModel>>(File.ReadAllText(filePath));
int count = datas.Count;
for (var i = 0; i < count - 1; i++)
{
for (var j = 0; j < count - 1 - i; j++)
{
if (String.Compare(datas[j].VIN, datas[j + 1].VIN) > 0)
{
UserModel temp = datas[j + 1];
datas[j + 1] = datas[j];
datas[j] = temp;
}
}
}
File.WriteAllText("./MOCK_DATA_SORTED.json",JsonConvert.SerializeObject(datas, Formatting.Indented));
}
當我運行.exe
時,顯然我無法將新文件MOCK_DATA_SORTED.json
保存在我想要的位置。
幫助!
您真的要在控制台應用程序中顯示另存為對話框嗎? 如果是這樣,請查看問題的評論,了解如何從您的控制台應用程序引用System.Windows.Forms
程序集,並將 using 指令添加到System.Windows.Forms
到您的Program
class。我覺得有點不對,如果你想一個打開對話框的應用程序,那么創建 Windows Forms 或 WPF 應用程序比控制台應用程序更容易。 但是我要判斷誰呢?
但是,如果您想要一個控制台應用程序,並且您希望運行該應用程序的人在運行該應用程序時決定將 output 文件保存在何處,我建議采用兩種方法。
首先,擴展Klaus Gutter 關於使用命令行 arguments 的評論。
在方法簽名中
public static void Main(string[] args)
該參數args
表示傳遞給您的應用程序的所有命令行 arguments。 例如,假設您的應用程序可執行文件名為 MyApp.exe 並且您從命令行調用它:
MyApp first second "third fourth fifth" sixth
那么args
會是一個有4個元素的數組,每個元素都是一個字符串,有如下值
first
second
third fourth fifth
sixth
(請注意命令行中“third fourth fifth”是如何用引號括起來的,這會導致 3 個單獨的單詞作為數組的單個元素而不是 3 個不同的元素傳遞,如果您的 arguments 包含空格,這將很有用。)
因此,對於您希望用戶在運行時指定目標路徑的用例,請考慮以下程序:
namespace StackOverflow68880709ConsoleAppArgs
{
using System;
using System.IO;
public class Program
{
public static void Main(string[] args)
{
if (args.Length < 1)
{
Console.WriteLine(@"Usage: MyApp C:\Temp");
return; // or you could throw an exception here
}
var destinationFolder = args[0];
if (!Directory.Exists(destinationFolder))
{
Console.WriteLine("Destination folder " + destinationFolder + " doesn't exist");
return; // or you could throw an exception here
}
var data = "stuff to write to the file"; // put your logic to create the data here
var destinationPath = Path.Combine(destinationFolder, "MOCK_DATA_SORTED.json");
File.WriteAllText(destinationPath, data);
}
}
}
假設您的應用程序仍然編譯為名為 MyApp.exe 的可執行文件,您可以像這樣從命令提示符運行它
MyApp C:\SomewhereIWantToSaveTheFile
或者
MyApp "C:\Some folder with spaces in the name"
如果您不希望您的用戶必須處理命令提示符並導航到部署 .exe 的位置,並且您希望他們能夠通過在文件資源管理器中雙擊它來啟動應用程序,您可以改用這種方法:
namespace StackOverflow68880709ConsoleAppArgs
{
using System;
using System.IO;
public class Program
{
public static void Main(string[] args)
{
Console.WriteLine("Please enter destination folder");
var destinationFolder = Console.ReadLine();
if (!Directory.Exists(destinationFolder))
{
Console.WriteLine("Destination folder " + destinationFolder + " doesn't exist");
return; // or you could loop back and prompt the user again
}
var data = "stuff to write to the file"; // put your logic to create the data here
var destinationPath = Path.Combine(destinationFolder, "MOCK_DATA_SORTED.json");
File.WriteAllText(destinationPath, data);
}
}
}
此版本允許用戶通過雙擊啟動應用程序,並在控制台 window 中提示他們輸入 output 文件應保存的文件夾路徑。
我已經向您展示了兩種在運行時將信息傳遞到控制台應用程序的非常常用的方法,希望您會發現它們有用。 如果您既是該應用程序的用戶又是其開發者,那么您也應該能夠以這兩種方式中的任何一種方式運行它。
但是,如果應用程序的用戶是其他一些人,他們堅持需要某種“另存為”對話框才能瀏覽到目標文件夾,那么只要您從 Windows Forms 或 WPF 等 UI 框架中引入該對話框,您不妨圍繞該框架構建您的應用程序,而不是使其成為控制台應用程序。
控制台應用程序非常適合非交互式批處理過程,它們非常適合技術嫻熟的用戶社區(想想開發人員、服務器管理員等),但對於習慣於做任何事情的非技術用戶來說就不是很好了通過單擊和拖放。
歡迎來到 Stack Overflow 順便說一句:-)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.