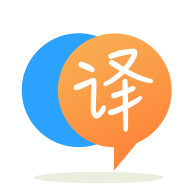
[英]Accessing variables from another classes with setter and getter JAVA
[英]Accessing array attributes from other classes with setter/getter
我是 Java 新手,想知道如何使用 setter/getter 訪問其他類的屬性(如果它們是數組)。
目前,我有一個日期類,它使用月/日/年的參數設置日期。 我需要創建另一個類,該類使用 date 類來設置雇用日期以存儲為屬性,以及其他類。
我的代碼目前看起來像這樣:
public class DateClass {
private int month;
private int day;
private int year;
// MONTH
public int getMonth() {
return month;
}
public void setMonth(int month) {
this.month = month;
}
// DAY
public int getDay() {
return day;
}
public void setDay(int day) {
this.day = day;
}
// YEAR
public int getYear() {
return year;
}
public void setYear(int year) {
this.year = year;
}
// FULL DATE
public void setDate(int month, int day, int year) {
setMonth(month);
setDay(day);
setYear(year);
}
public int[] getDate() {
return new int[] { month, day, year };
}
}
這是第二堂課:
public class EmployeeClass {
private int[] dateOfHire;
public void setDateOfHire(int[] dateOfHire) {
dateOfHire.setDate(dateOfHire);
}
public String[] getDateOfHire() {
return dateOfHire.getDate(dateOfHire);
}
}
錯誤說:無法在數組類型 int[] 上調用 setDate(int[])
我怎樣才能解決這個問題? 謝謝!
您需要進行一些更改。 首先,您的EmployeeClass
應該有一個DateClass
屬性而不是int[]
並且setDateOfHire()
方法必須調用具有 3 個參數的DateClass
中可用的setDate()
方法:
public class EmployeeClass {
private DateClass dateOfHire;
public void setDateOfHire(int[] dateOfHire) {
this.dateOfHire.setDate(dateOfHire[0], dateOfHire[1], dateOfHire[2]);
}
public int[] getDateOfHire() {
return dateOfHire.getDate();
}
}
這會奏效,但我建議你檢查一下你的類設計。 讓int
數組定義日期是一種非常糟糕的做法。
您正在聲明一個整數基元數組private int[] dateOfHire;
要聲明一個DateClass
數組,只需使用private DateClass[] dateOfHire
[]
之前定義的原語或類決定了被初始化的數組的類型
您可以將此作為參考
您需要創建一個 DateClass 對象才能使用其方法。 考慮像這樣改變你的 EmployeeClass :
public class EmployeeClass {
private int[] dateOfHire; // not sure of the purpose of this array
private DateClass dateClassObject; // your variable name is up to you
public EmployeeClass () { // Constructor for this class
dateClassObject = new DateClass(); // created a new DateClass in the constructor
}
public void setDateOfHire(int[] dateOfHire) {
// this dateOfHire array is the array that is passed in when
// method is called. Do not confuse it the array declared as a property.
int day = dateOfHire[0] // assuming the day is in index 0 of the array
int month = dateOfHire[1] // assuming the month is in index 1 of the array
int year = dateOfHire[2] // assuming the year is in index 2 of the array
dateClassObject.setDate(month, day, year); // changed to dateClassObject. Also changed setDate to pass correct parameters
}
public int[] getDateOfHire() { // changed String[] to int[] because returned value of getDate() is a int array.
return dateClassObject.getDate(); // changed to dateClassObject. Removed the parameter.
}
}
在setDateOfHire
只需設置值而不是對其調用方法:
public void setDateOfHire(int[] dateOfHire) {
this.dateOfHire = dateOfHire;
}
將它與您在 DateClass 中實現 setter 的方式進行比較。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.