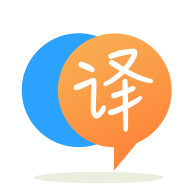
[英]I’m trying to code a blackjack game, can anyone help me with my score-checking solution? Python
[英]Need help on my first Blackjack Game (Text Version) in Python
我剛剛開始學習如何使用 python 編碼。 由於我是初學者,我正在嘗試制作僅基於文本的二十一點游戲。
二十一點中的一些規則也被簡化了:
我在運行代碼時遇到了一些錯誤:
注意:我可能遺漏了一些其他錯誤。
有沒有人願意給我一些關於修復上述錯誤的建議?
我的代碼貼在下面:
import random
def game_started():
print('Hi! This is a Blackjack Game')
print('\nDealers cards:')
dealer_card_1 = random.randint(1,11)
dealer_card_2 = random.randint(1,11)
print(dealer_card_1)
print(dealer_card_2)
sum_dealer = dealer_card_1 + dealer_card_2
print('Sum of cards on the dealer hand:')
print(sum_dealer)
print('\nYour cards:')
player_card_1 = random.randint(1,11)
player_card_2 = random.randint(1,11)
print(player_card_1)
print(player_card_2)
sum_player = player_card_1 + player_card_2
print('Sum of cards on your hand:')
print(sum_player)
#####################################################################################
print('\nPlayer, hit or stand?')
player_choose = input("")
if player_choose == 'hit':
print('***************************************************************')
while sum_player <= 11 and sum_dealer < 17:
dealer_card_new = random.randint(1,11)
print('\nDealer has the following cards:')
print(dealer_card_1)
print(dealer_card_2)
print(dealer_card_new)
sum_dealer += dealer_card_new
print('Sum of dealer cards:')
print(sum_dealer)
player_card_new = random.randint(1,11)
print('\nPlayer has the following cards:')
print(player_card_1)
print(player_card_2)
print(player_card_new)
sum_player += player_card_new
print('Sum of player cards:')
print(sum_player)
if 12 <= sum_player <= 16 and sum_dealer > sum_player and 17 <= sum_dealer <= 21:
print('Dealer wins!')
break
elif sum_dealer < 11 and sum_dealer < 11:
continue
elif sum_player <= 11 and sum_dealer > sum_player and 17 <= sum_dealer <= 21:
player_card_new = random.randint(1,11)
print('\nPlayer has the following cards:')
print(player_card_1)
print(player_card_2)
print(player_card_new)
sum_player += player_card_new
print('Sum of player cards:')
print(sum_player)
if 12 <= sum_player <= 16 and sum_dealer > sum_player and 17 <= sum_dealer <= 21:
print('Dealer wins!')
break
elif 17 <= sum_player <= 21 and sum_dealer > sum_player and 17 <= sum_dealer <= 21:
print('Dealer wins!')
break
elif 12 <= sum_player <= 16 and sum_dealer < sum_player and 17 <= sum_dealer <= 21:
print('Player wins!')
break
elif 17 <= sum_player <= 21 and sum_dealer < sum_player and 17 <= sum_dealer <= 21:
print('Player wins!')
break
elif 17 <= sum_player <= 21 and sum_dealer == sum_player == 17 and 17 <= sum_dealer <= 21:
print('Push!')
break
elif sum_player == sum_dealer == 17 or sum_player == sum_dealer == 18 or sum_player == sum_dealer == 19 or sum_player == sum_dealer == 20 or sum_player == sum_dealer == 21:
print('Push!')
break
elif sum_player > 21 and sum_dealer <= 21:
print('Player busts! Dealer wins!')
break
elif sum_dealer > 21 and sum_player <= 21:
print('Dealer busts! Player wins!')
break
else:
print('Player wins')
break
while 12 <= sum_player <= 21 and sum_dealer < 17:
dealer_card_new = random.randint(1,11)
print('\nDealer has the following cards:')
print(dealer_card_1)
print(dealer_card_2)
print(dealer_card_new)
sum_dealer += dealer_card_new
print('Sum of dealer cards:')
print(sum_dealer)
if 17 <= sum_dealer <= 21 and sum_dealer > sum_player and 12 <= sum_player <= 21:
print('Dealer wins!')
break
elif 17 <= sum_dealer <= 21 and sum_dealer < sum_player and 12 <= sum_player <= 21:
print('Player wins!')
break
elif sum_player == sum_dealer == 17:
print('Push!')
break
while sum_player <= 11 and sum_dealer == 17:
player_card_new = random.randint(1,11)
print('\nPlayer has the following cards:')
print(player_card_1)
print(player_card_2)
print(player_card_new)
sum_player += player_card_new
print('Sum of player cards:')
print(sum_player)
if 12 <= sum_player < 17 and sum_dealer > sum_player and sum_dealer == 17:
print('Dealer wins!')
break
elif 12 <= sum_player < 17 and sum_dealer < sum_player and sum_dealer == 17:
print('Player wins!')
break
elif sum_player == sum_dealer == 17:
print('Push!')
break
while sum_player <= 11 and 17 <= sum_dealer <= 21:
player_card_new = random.randint(1,11)
print('\nPlayer has the following cards:')
print(player_card_1)
print(player_card_2)
print(player_card_new)
sum_player += player_card_new
print('Sum of player cards:')
print(sum_player)
if 12 <= sum_player < 17 and sum_dealer > sum_player and sum_dealer == 17:
print('Dealer wins!')
break
elif 12 <= sum_player < 17 and sum_dealer < sum_player and sum_dealer == 17:
print('Player wins!')
break
elif sum_player == sum_dealer == 17:
print('Push!')
break
####################################################################################
elif player_choose == 'stand':
print('**************************************************************')
while sum_dealer < 17 and 12 <= sum_player <= 21:
dealer_card_new = random.randint(1,11)
print('\nDealer has the follwing cards:')
print(dealer_card_1)
print(dealer_card_2)
print(dealer_card_new)
sum_dealer += dealer_card_new
print('Sum of dealer cards:')
print(sum_dealer)
if 17 <= sum_dealer <= 21 and sum_dealer > sum_player and 12 <= sum_player <= 21:
print('Dealer wins!')
break
elif 17 <= sum_dealer <= 21 and sum_dealer < sum_player and 12 <= sum_player <= 21:
print('Player wins!')
break
elif sum_dealer > 21 and sum_dealer > sum_player and 12 <= sum_player <= 21:
print('Dealer busts! Player wins')
break
elif 17 <= sum_dealer <= 21 and sum_dealer == sum_player and 12 <= sum_player <= 21:
print('Push!')
break
while 17 <= sum_dealer <= 21 and 12 <= sum_player <= 21:
if sum_dealer > sum_player:
print('Dealer wins!')
elif sum_dealer < sum_player:
print('Player wins!')
elif sum_dealer == sum_player:
print('Push!')
game_started()
我看到了一些錯誤,包括游戲本身的邏輯。 例如,當玩家擊中時,你給庄家一張牌。 一旦發牌,庄家在玩家起立或爆牌之前不會抽牌。
您還想將程序的邏輯分解成更小的塊。 您可以將這些塊放入函數中。 下面的代碼應該讓你走上正軌。
from random import randint
# define a deal function - if you're new to functions,
# this code is just a definition. It doesn't run until
# you say 'deal()' in your program
def deal():
"""
Deal the cards. Since a hand is a group of cards, it is
easier to add them to a list, which holds a group of items.
"""
player_hand = [randint(1, 11), randint(1, 11)]
dealer_hand = [randint(1, 11), randint(1, 11)]
return player_hand, dealer_hand
# draw the next card
def draw_card():
return randint(1, 11)
# this function tells you how many aces are in your hand
# so you don't bust on a soft hand.
def count_aces(cards):
return sum([1 for card in cards if card ==11])
# take a card hand and return a boolean if the cards sum is greater than 21.
# notice we use the sum_hands function which we define below
def check_bust(cards):
return sum_hand(cards) > 21
# sum the hand given aces can be 1 or 11. Always take the maximum
# hand that doesn't bust
def sum_hand(cards):
total = sum(cards)
aces = count_aces(cards)
# return first hand less than 21
if total < 22:
return total
while aces > 0:
total = total - 10
aces = aces - 1
if total < 21:
return total
return sum(cards)
# just a helper to print out a hand for display
def display_hand(cards):
total = sum_hand(cards)
cards = [str(card) for card in cards]
print(' '.join(cards))
print(f'total is {total}')
print('')
def play_game():
# stay optimistic
player_wins = True
# deal the cards.
player_cards, dealer_cards = deal()
#
print('Hi! This is a Blackjack Game')
print('\nDealers cards:')
display_hand(dealer_cards)
# let player player
while True:
print('\nPlayer cards:')
display_hand(player_cards)
print('\nPlayer, hit or stand?')
player_choose = input("")
# this is an f-string - you can print variables inside the {}
print(f'player {player_choose}s')
if player_choose == "stand":
break
# draw the next card
next_card = draw_card()
player_cards.append(next_card)
# check for bust
busted = check_bust(player_cards)
if busted:
player_wins = False
break
print('*****************************************')
print('dealer drawing cards')
print('')
# if player busted there is no need for dealer to draw
if not player_wins:
print('dealer has')
display_hand(dealer_cards)
print('player has:')
display_hand(player_cards)
print('you lose')
return
# allow dealer to play
while True:
total = sum_hand(dealer_cards)
if total < 16:
dealer_cards.append(draw_card())
continue
if total > 21:
break
player_wins = False
break
if player_wins:
print('dealer has')
display_hand(dealer_cards)
print('player has:')
display_hand(player_cards)
print('You win')
return
print('dealer has')
display_hand(dealer_cards)
print('player has:')
display_hand(player_cards)
print('you lose')
play_game()
您可以進一步優化它。 我在那里留下了一些重復的代碼,您可以根據需要將其重寫為函數。 最終的 play game 函數讀起來很流暢:
def play_game():
player_cards, dealer_cards = deal()
prompt()
player_plays(player_cards)
dealer_plays(dealer_cards)
result_prompt()
這只是一個粗略的例子,不是黃金標准或任何東西,只是為了顯示當你把它分解成更小的部分時,事情會變得更加清晰。 如果您對此有任何疑問,請告訴我。
謝謝,我已經進一步檢查了我的代碼。 請看一下。
import random
# define a deal function
# Dealer deals the card to the player and himself
def deal():
dealer_hand = [random.randint(1,11), random.randint(1,11)]
player_hand = [random.randint(1,11), random.randint(1,11)]
return dealer_hand, player_hand
# define a function to display all the cards of the dealer and player
def display_hand(cards):
total = sum_hand(cards)
cards = [str(card) for card in cards]
print(' '.join(cards))
print(f'total is {total}')
print('')
# define a deal function for the dealer and player to draw a card
def draw_card():
return random.randint(1,11)
# define a sum function to count the value of a hand
def sum_hand(cards):
total = sum(cards)
aces = count_aces(cards)
# return the initial hand if the sum is less than 21
if total < 22:
return total
while aces > 0:
total = total - 10
aces = aces - 1
if total < 21:
return total
return sum(cards)
# define a function to count aces
def count_aces(cards):
return sum([1 for card in cards if card == 11])
# define a function to check bust for player and dealer
def check_bust(cards):
return sum_hand(cards) > 21
# define a function to play the game
def play():
# Assume the player plays the game rationally and only the dealer can go bust.
possibility_dealer_bust = True
dealer_cards, player_cards = deal()
print('')
print('Dealer has:')
display_hand(dealer_cards)
while True:
print('Player has:')
display_hand(player_cards)
ask = input("\nPlayer, hit or stand?\n")
print(f'player chooses to {ask}')
if ask == 'stand':
break
next_card = draw_card()
player_cards.append(next_card)
busted = check_bust(player_cards)
if busted:
possibility_dealer_bust = False
break
print('*****************************************')
print('dealer drawing cards')
print('')
while True:
total_d = sum_hand(dealer_cards)
total_p = sum_hand(player_cards)
# Scenario 1 (if loop = if possibility_dealer_bust)
# Dealer bust! This while loop ends and gets to the if loop in line 99 directly.
if total_d > 21:
break
# Scenario 2 (if loop = if not possibility_dealer_bust)
# Dealer not bust! This while loop continues until the dealer has a value between 17 and 21
# Goes to if loop in line 107
if total_d <= 16:
dealer_cards.append(draw_card())
continue
possibility_dealer_bust = False
break
# Scenario 1
if possibility_dealer_bust:
print('dealer has:')
display_hand(dealer_cards)
print('player has:')
display_hand(player_cards)
print('Dealer bust! Player Wins!')
# Scenario 2
if not possibility_dealer_bust:
if total_d == total_p:
print('dealer has:')
display_hand(dealer_cards)
print('player has:')
display_hand(player_cards)
print('Push!')
elif total_p > total_d:
print('dealer has:')
display_hand(dealer_cards)
print('player has:')
display_hand(player_cards)
print('Player wins!')
else:
print('dealer has:')
display_hand(dealer_cards)
print('player has:')
display_hand(player_cards)
print('Dealer wins!')
play()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.