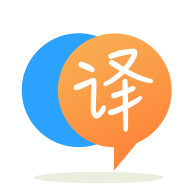
[英]('…').each not a valid function for asp.net core mvc with jquery bundle
[英]Display a total of values by each person in ASP.NET Core MVC
我有一個籃球統計數據的數據庫表,我想知道顯示一個單獨的表的簡單方法,該表顯示每個球員的每個統計數據的總數。
我在下面有一張圖片,展示了統計表的樣子。
我是 .NET 的新手,所以我不太確定最好的方法是什么。 我在互聯網上環顧四周,但找不到與我的問題類似的東西。
控制器
using Block_City_Website.Data;
using Block_City_Website.Models;
using Block_City_Website.Models.ViewModels;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Rendering;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
namespace Block_City_Website.Controllers
{
public class StatController : Controller
{
private readonly ApplicationDbContext _db;
public StatController(ApplicationDbContext db)
{
_db = db;
}
public IActionResult Index()
{
IEnumerable<Stat> objList = _db.Stats;
foreach(var obj in objList)
{
obj.Player = _db.Players.FirstOrDefault(u => u.Id == obj.PlayerId);
obj.Team = _db.Teams.FirstOrDefault(u => u.Id == obj.TeamId);
}
return View(objList);
}
// GET-Create
public IActionResult Create()
{
//IEnumerable<SelectListItem> TypeDropDown = _db.Players.Select(i => new SelectListItem
//{
// Text = i.FirstName + " " +i.LastName,
// Value = i.Id.ToString()
//});
//ViewBag.TypeDropDown = TypeDropDown;
StatVM statVM = new StatVM()
{
Stat = new Stat(),
TypeDropDown = _db.Players.Select(i => new SelectListItem
{
Text = i.FirstName + " " + i.LastName,
Value = i.Id.ToString()
}),
TypeDropDown1 = _db.Teams.Select(i => new SelectListItem
{
Text = i.TeamName,
Value = i.Id.ToString()
})
};
return View(statVM);
}
//POST-Create
[HttpPost]
[ValidateAntiForgeryToken]
public IActionResult Create(StatVM obj)
{
if (ModelState.IsValid)
{
//obj.PlayerId = 1;
//obj.TeamId = 1;
_db.Stats.Add(obj.Stat);
_db.SaveChanges();
return RedirectToAction("Index");
}
return View(obj);
}
// GET-Delete
public IActionResult Delete(int? id)
{
if (id == null || id == 0)
{
return NotFound();
}
var obj = _db.Stats.Find(id);
if (obj == null)
{
return NotFound();
}
return View(obj);
}
// POST-Delete
[HttpPost]
[ValidateAntiForgeryToken]
public IActionResult DeletePost(int? id)
{
var obj = _db.Stats.Find(id);
if (obj == null)
{
return NotFound();
}
_db.Stats.Remove(obj);
_db.SaveChanges();
return RedirectToAction("Index");
}
// GET Update
public IActionResult Update(int? id)
{
StatVM expenseVM = new StatVM()
{
Stat = new Stat(),
TypeDropDown = _db.Players.Select(i => new SelectListItem
{
Text = i.FirstName + " " + i.LastName,
Value = i.Id.ToString()
}),
TypeDropDown1 = _db.Teams.Select(i => new SelectListItem
{
Text = i.TeamName,
Value = i.Id.ToString()
})
};
if (id == null || id == 0)
{
return NotFound();
}
expenseVM.Stat = _db.Stats.Find(id);
if (expenseVM.Stat == null)
{
return NotFound();
}
return View(expenseVM);
}
// POST UPDATE
[HttpPost]
[ValidateAntiForgeryToken]
public IActionResult Update(StatVM obj)
{
if (ModelState.IsValid)
{
_db.Stats.Update(obj.Stat);
_db.SaveChanges();
return RedirectToAction("Index");
}
return View(obj);
}
public IActionResult TotStats()
{
IEnumerable<Stat> objList = _db.Stats;
foreach (var obj in objList)
{
obj.Player = _db.Players.FirstOrDefault(u => u.Id == obj.PlayerId);
obj.Team = _db.Teams.FirstOrDefault(u => u.Id == obj.TeamId);
}
return View(objList);
}
}
}
看法
@model IEnumerable<Block_City_Website.Models.Stat>
@using static MoreLinq.Extensions.LagExtension;
@using static MoreLinq.Extensions.LeadExtension;
@using MoreEnumerable = MoreLinq.MoreEnumerable;
<div class="container p-3">
<div class="row pt-4">
<div class="col-6">
<h2 class="text-primary">Stats List</h2>
</div>
<div class="col-6 text-right">
<a asp-controller="Stat" asp-action="Index" class="btn btn-primary">Back to Stats</a>
</div>
</div>
<br />
@if (Model.Count() > 0)
{
<table class="table table-bordered table-striped" style="width:100%">
<thead>
<tr>
<th>
Player
</th>
<th>
Team
</th>
<th>
Points
</th>
<th>
Rebounds
</th>
<th>
Assists
</th>
<th>
Steals
</th>
<th>
Blocks
</th>
</tr>
</thead>
<tbody>
@foreach (var stat in Model)
{
<tr>
<td width="10%">@stat.Player.FirstName @stat.Player.LastName</td>
<td width="10%">@stat.Team.TeamName</td>
<td width="10%">@stat.Points</td>
<td width="10%">@stat.Rebounds</td>
<td width="10%">@stat.Assists</td>
<td width="10%">@stat.Steals</td>
<td width="10%">@stat.Blocks</td>
</tr>
}
</tbody>
</table>
}
else
{
<p>No Stats created yet</p>
}
</div>
模型
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.ComponentModel.DataAnnotations;
using System.ComponentModel.DataAnnotations.Schema;
using System.Linq;
using System.Threading.Tasks;
namespace Block_City_Website.Models
{
public class Stat
{
[Key]
public int Id { get; set; }
[Required]
[Range(0, int.MaxValue, ErrorMessage ="Amount must not be less than 0")]
public int Points { get; set; }
[Required]
[Range(0, int.MaxValue, ErrorMessage = "Amount must not be less than 0")]
public int Rebounds { get; set; }
[Required]
[Range(0, int.MaxValue, ErrorMessage = "Amount must not be less than 0")]
public int Assists { get; set; }
[Required]
[Range(0, int.MaxValue, ErrorMessage = "Amount must not be less than 0")]
public int Steals { get; set; }
[Required]
[Range(0, int.MaxValue, ErrorMessage = "Amount must not be less than 0")]
public int Blocks { get; set; }
[Required]
[DisplayName("Player")]
public int PlayerId { get; set; }
[ForeignKey("PlayerId")]
public virtual Player Player { get; set; }
[Required]
[DisplayName("Team")]
public int TeamId { get; set; }
[ForeignKey("TeamId")]
public virtual Team Team { get; set; }
}
}
您可以添加顯示每個統計數據總和的表格,例如
<-----table----->
-----------------
| Player | Total |
------------------
| Player1 | 54 |
| Player2 | 60 |
| Player3 | 75 |
| Player4 | 80 |
| Player5 | 90 |
------------------
在 Html 中添加表並使用相同的模型創建此表。 在列中的每個循環 sum stat 中,例如:
<table>
<thead>
<th>Player Name</th>
<th>Stat Total</th>
</thead>
<tbody>
@foreach (var stat in Model)
{
<tr>
<td width ="10%">@stat.Player.FirstName @stat.Player.LastName</td>
<td width ="10%">@stat.Points + @stat.Rebounds + @stat.Assists + @stat.Steals + @stat.Blocks</td>
</tr>
}
</tbody>
</table>
由於 stat 模型屬性數據類型是“int”,您可以輕松地將它們相加以獲得總數。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.