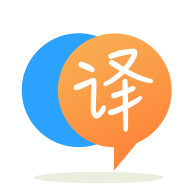
[英]Find absolute maximum or minimum at specific position in list by Python
[英]How to find the position of the maximum and minimum number in a list?
對於這個作業,我需要編寫一個程序來詢問每個月的降雨量。 用戶輸入金額並將其附加到列表中。 每月收集數據后,程序將計算並輸出總降雨量、平均降雨量、降雨量最高的月份和降雨量最少的月份。 我遇到問題的部分是返回降雨量最高和最低的月份。 我不想使用 sort 方法,因為我打算使用 maxRain 函數遍歷rainList 來查找最大值並返回位置。 然后在主函數中打印月份列表中對應位置的月份。 除非有另一種方法可以將該月的降雨量分配給該月並以另一種方式返回。
# PURPOSE: This program lets the user enter the total rainfall for each of
# of 12 months then calculates total, average, min and max rainfall
months = ["January", "February", "March", "April",
"May", "June", "July", "August",
"September", "October", "November", "December"]
# function that gets amount of rainfall for each month and appends it to a list
def getRainFall():
nums = []
for i in range(len(months)):
m = months[0+i]
print("Enter the rainfall for ", m)
x = input()
x = float(x)
nums.append(x)
return nums
# function that adds the numbers of the list and returns the sum
def totalRain(nums):
total = 0
for num in nums:
total = total + num
return total
# function to calculate and return the average of numbers from a list
def mean(nums):
total = 0.0
for num in nums:
total = total + num
return total / len(nums)
def maxRain(nums):
for i in range(len(nums)):
if nums[i] > nums[i+1]:
x = nums[i]
else:
x = nums[i+1]
return x
#def minRain(nums):
def main():
rainList = getRainFall()
rainAverage = mean(rainList)
total = totalRain(rainList)
highest = maxRain(rainList)
#lowest = minRain(rainList)
print("Total rainfall:", total)
print("Average rainfall:", rainAverage)
print("Highest rainfall:", highest)
#print("Lowest rainfall:", lowest)
# close the program
input("Press the <Enter> key to quit")
main()
回答核心問題:
nums = [32, 37, 28, 30, 37, 25, 27, 24, 35, 55, 23, 31, 55, 21, 40, 18, 50, 35, 41, 49,
37, 19, 40, 41, 31]
list_min, list_max = min(nums), max(nums)
mins = [i for i, j in enumerate(nums) if j == list_min]
maxs = [i for i, j in enumerate(nums) if j == list_max]
print(f'{mins=}, {maxs=}') $ -> mins=[15], maxs=[9, 12]
所以我對你的代碼做了一些改動,我通常不喜歡這樣做,因為我認為它不太有用。 然而,在這種情況下,它只會使整個過程變得更加簡單。
這使用內置的 Python 函數來完成您手動執行的操作。
months = ["January", "February", "March", "April",
"May", "June", "July", "August",
"September", "October", "November", "December"]
# function that gets amount of rainfall for each month and appends it to a list
def getRainFall():
nums = []
for month in months:
m = month
print("Enter the rainfall for ", m)
x = input()
x = float(x)
nums.append(x)
return nums
def main():
rainList = getRainFall()
total = sum(rainList)
rainAverage = total/len(rainList) # Sum/number of points
highest = months[rainList.index(max(rainList))] # Max rain index into months arr
lowest = months[rainList.index(min(rainList))] # Min rain index into months arr
print("Total rainfall:", total)
print("Average rainfall:", rainAverage)
print("Highest rainfall:", highest)
print("Lowest rainfall:", lowest)
# close the program
input("Press the <Enter> key to quit")
main()
示例輸出:
Enter the rainfall for January
1
Enter the rainfall for February
2
Enter the rainfall for March
3
Enter the rainfall for April
45
Enter the rainfall for May
5
Enter the rainfall for June
6
Enter the rainfall for July
7
Enter the rainfall for August
8
Enter the rainfall for September
9
Enter the rainfall for October
0
Enter the rainfall for November
1
Enter the rainfall for December
2
Total rainfall: 89.0
Average rainfall: 7.416666666666667
Highest rainfall: April
Lowest rainfall: October
Press the <Enter> key to quit
由於這個nums[i+1]
,你 maxRain 不起作用,當i
在最后一個索引中時,你要求下一個並給你一個錯誤,要修復它,你的范圍從 1 (range(1,len(nums) )) 並將您的[i]
更改為[i-1]
並將您的[i+1]
更改為[i]
或將其更改為len(nums)-1
以避免此問題。
無論您采用上述解決方案,在此函數中,您還可以跟蹤索引並將其與值一起返回,因為如果放入元組或其他對象中,則可以返回多個值,就像return index,x
簡單return index,x
或return (index,x)
如果您喜歡帶括號的
或者,您可以輕松地使用內置enumerate
與鍵函數一起使用max
或min
給定列表的索引和最大或最小元素
>>> nums=[1,2,34,4,25,0,3]
>>> max(enumerate(nums),key=lambda x:x[1])
(2, 34)#index, element
>>> min(enumerate(nums),key=lambda x:x[1])
(5, 0)
>>>
一個關鍵函數只不過是一個函數,它接受列表中的元素或可迭代的元素(任何你可以調用iter
東西)並將其提取或轉換成我們希望它進行比較的東西,在示例中是.. .
>>> list(enumerate(nums))
[(0, 1), (1, 2), (2, 34), (3, 4), (4, 25), (5, 0), (6, 3)]
>>>
...我們從枚舉中獲得的元組的第二個元素, lambda部分是一種聲明簡單匿名函數的方法,因此我們不必執行以下操作並改用它
def second_elem(obj):
return obj[1]
另一件事是您可以直接在 for 循環中使用列表,無需在列表的 len 范圍內執行此操作
>>> months = ["January", "February", "March", "April",
"May", "June", "July", "August",
"September", "October", "November", "December"]
>>> for month in months:
print(month)
January
February
March
April
May
June
July
August
September
October
November
December
>>>
對於更多的對象也是如此,例如元組、字典、字符串等,任何可迭代的對象
您的mean
和totalRain
函數沒有錯,但可以通過使用sum
函數使其更簡單
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.