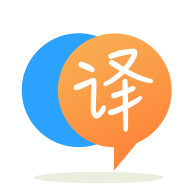
[英]Saving pointer to last node in doubly linked list when performing insertion sort
[英]code crashes when i use the last pointer in doubly linked list
所以我為雙向鏈表編寫了一些代碼,並在制作 function 以在末尾添加一個節點時,我想為最后一個節點制作一個指針,但是當我執行它以最后添加時它崩潰但在前面添加結束它工作正常。 一切看起來都很好,它甚至沒有顯示任何錯誤,只是崩潰了。
#include <stdio.h>
#include <stdlib.h>
struct node
{
int data;
struct node *lptr;
struct node *rptr;
};
typedef struct node *Node;
Node pos(Node first, Node last)
{
Node new;
new = (Node)malloc(sizeof(struct node));
new->lptr = NULL;
new->rptr = NULL;
printf("Enter data: ");
scanf("%d", &new->data);
if (first == NULL)
{
first = new;
last = new;
}
else
{
int p;
printf("1) First\n2) Last\n");
scanf("%d", &p);
switch (p)
{
case 1:
first->lptr = new;
new->rptr = first;
first = new;
break;
case 2:
last->rptr = new;
new->lptr = last;
last = new;
break;
default:
break;
}
}
return first;
}
void dis(Node first)
{
Node p;
int c = 1;
if (first == NULL)
{
printf("Empty");
}
else
{ p=first;
while (p != NULL)
{
printf("%dst element is %d\n", c, p->data);
c++;
p = p->rptr;
}
}
}
int main()
{
int ch;
Node first, last, t;
first = NULL;
last = NULL;
for (;;)
{
printf("Insert: \n");
scanf("%d", &ch);
switch (ch)
{
case 1:
first = pos(first, last);
break;
case 2:
dis(first);
break;
default:
printf("invalid");
exit(0);
}
}
return 0;
}
認為問題出在這部分;
case 2:
last->rptr = new;
new->lptr = last;
last = new;
break;
問題是 function pos
不會更改last
在main
中聲明的指針。 它last
改變了它的局部變量(參數),該變量由main
中last
聲明的指針的值 pf 的副本初始化。 但是last
在 main 中聲明的指針保持不變。
您應該再聲明一個結構,例如
struct List
{
struct node *first;
struct node *last;
};
並將此結構用作函數的參數。
//...
int pos( struct List *list );
int main( void )
{
struct List list = { .first = NULL, .last = NULL };
pos( &list );
//...
}
此外,最好將 function 分成兩個功能。 第一個將數據添加到列表的開頭,第二個將數據添加到列表的尾部。
例如
int push_front( struct List *list, int data );
int push_back( struct List *list, int data );
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.