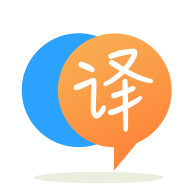
[英]How to do variable assignment inside a while(expression) loop in Python?
[英]How do i complete maths and variable assignment inside a dictionary in python?
Player1 = {
"name":"Bob",
"attack":7,
"defence":5,
"total":Player1["attack"]+Player1["defence"],
}
如何將字典中的總數設置為攻擊+防御?
不幸的是,據我所知,您要問的問題是……您最好使用 class
像這樣:
class Player:
def __init__(self):
self.name = "Bob"
self.attack = 7
self.defence = 5
self.total = self.attack + self.defence
player = Player()
print(player.total)
您必須記住的是,您在聲明 dict 時並未對其進行實例化,因此在{}
內您不能調用Player1
,因為它在上下文中尚不存在。
通過使用類,您還可以通過執行以下操作來重用上面的示例:
class Player:
def __init__(self, name, attack, defence):
self.name = name
self.attack = attack
self.defence = defence
self.total = self.attack + self.defence
player1 = Player(name="bob", attack=7, defence=5)
player2 = Player(name="bill", attack=10, defence=7)
print(player1.total)
print(player2.total)
編輯:修正錯字
您當前正在嘗試在創建Player1
之前訪問它。
你可以這樣做:
Player1 = {
"name":"Bob",
"attack":7,
"defence":5
}
Player1["total"] = Player1["attack"] + Player1["defence"]
但是,這並不理想,因為您需要記住在'attack'
或'defence'
發生變化時調整'total'
字段。 最好即時計算總值,因為它不是昂貴的計算。
這可以通過編寫具有屬性total
的Player
class 來實現。
class Player:
def __init__(self, name, attack, defence):
self.name = name
self.attack = attack
self.defence = defence
@property
def total(self):
return self.attack + self.defence
演示:
>>> Player1 = Player('Bob', 1, 2)
>>> Player1.name, Player1.attack, Player1.defence, Player1.total
('Bob', 1, 2, 3)
假設您不想自己計算鍵總計的值。 您可以將其初始化為None
(標准做法。比省略它更好)。
Player1 = {
"name":"Bob",
"attack":7,
"defence":5,
"total":None
}
然后稍后更新它的值。
Player1["total"] = Player1["attack"] + Player1["defence"]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.