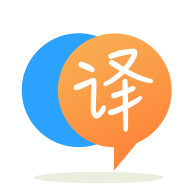
[英]jquery problem with sending json data to ASP.NET WebMethod
[英]Sending JSON object successfully to ASP.NET WebMethod, using jQuery
我已經為此工作了 3 個小時,然后放棄了。 我只是想使用 jQuery 將數據發送到 ASP.NET WebMethod。 數據基本上是一堆鍵/值對。 所以我嘗試創建一個數組並將對添加到該數組。
我的 WebMethod (aspx.cs) 看起來像這樣(這對於我用 JavaScript 構建的內容可能是錯誤的,我只是不知道):
[WebMethod]
public static string SaveRecord(List<object> items)
{
...
}
這是我的示例 JavaScript:
var items = new Array;
var data1 = { compId: "1", formId: "531" };
var data2 = { compId: "2", formId: "77" };
var data3 = { compId: "3", formId: "99" };
var data4 = { status: "2", statusId: "8" };
var data5 = { name: "Value", value: "myValue" };
items[0] = data1;
items[1] = data2;
items[2] = data3;
items[3] = data4;
items[4] = data5;
這是我的 jQuery AJAX 調用:
var options = {
error: function(msg) {
alert(msg.d);
},
type: "POST",
url: "PackageList.aspx/SaveRecord",
data: { 'items': items },
contentType: "application/json; charset=utf-8",
dataType: "json",
async: false,
success: function(response) {
var results = response.d;
}
};
jQuery.ajax(options);
我收到錯誤:
Invalid JSON primitive: items.
所以,如果我這樣做:
var DTO = { 'items': items };
並像這樣設置數據參數:
data: JSON.stringify(DTO)
然后我得到這個錯誤:
Cannot convert object of type \u0027System.String\u0027 to type \u0027System.Collections.Generic.List`1[System.Object]\u0027
在您的示例中,如果您的數據參數是:
data: "{'items':" + JSON.stringify(items) + "}"
請記住,您需要將 JSON 字符串發送到 ASP.NET AJAX。 如果您指定一個實際的 JSON 對象作為 jQuery 的數據參數,它會將其序列化為 &k=v?k=v 對。
看起來您已經閱讀過它,但請再看一下我將 JavaScript DTO 與 jQuery、JSON.stringify 和 ASP.NET AJAX 結合使用的示例。 它涵蓋了完成這項工作所需的一切。
注意:您永遠不應該使用 JavaScriptSerializer 在“ScriptService”中手動反序列化 JSON(正如其他人所建議的)。 它會根據方法的指定參數類型自動為您執行此操作。 如果你發現自己這樣做了,那你就做錯了。
使用 AJAX.NET 時,我總是將輸入參數設為一個普通的舊對象,然后使用 javascript 反序列化器將其轉換為我想要的任何類型。 至少這樣你可以調試並查看 web 方法正在接收什么類型的對象。
使用 jQuery 時需要將對象轉換為字符串
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<asp:ScriptManager ID="sm" runat="server" EnablePageMethods="true">
<Scripts>
<asp:ScriptReference Path="~/js/jquery.js" />
</Scripts>
</asp:ScriptManager>
<div></div>
</form>
</body>
</html>
<script type="text/javascript" language="javascript">
var items = [{ compId: "1", formId: "531" },
{ compId: "2", formId: "77" },
{ compId: "3", formId: "99" },
{ status: "2", statusId: "8" },
{ name: "Value", value: "myValue"}];
//Using Ajax.Net Method
PageMethods.SubmitItems(items,
function(response) { var results = response.d; },
function(msg) { alert(msg.d) },
null);
//using jQuery ajax Method
var options = { error: function(msg) { alert(msg.d); },
type: "POST", url: "WebForm1.aspx/SubmitItems",
data: {"items":items.toString()}, // array to string fixes it *
contentType: "application/json; charset=utf-8",
dataType: "json",
async: false,
success: function(response) { var results = response.d; } };
jQuery.ajax(options);
</script>
以及背后的代碼
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Services;
using System.Web.Script.Serialization;
using System.Web.Script.Services;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace CustomEquip
{
[ScriptService]
public partial class WebForm1 : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
}
[WebMethod]
public static void SubmitItems(object items)
{
//break point here
List<object> lstItems = new JavaScriptSerializer().ConvertToType<List<object>>(items);
}
}
}
以下是我們項目的代碼片段 - 我在不將對象包裝為字符串以及日期值時遇到了麻煩 - 希望這對某人有所幫助:
// our JSON data has to be a STRING - need to send a JSON string to ASP.NET AJAX.
// if we specify an actual JSON object as jQuery's data parameter, it will serialize it as ?k=v&k=v pairs instead
// we must also wrap the object we are sending with the name of the parameter on the server side – in this case, "invoiceLine"
var jsonString = "{\"invoiceLine\":" + JSON.stringify(selectedInvoiceLine) + "}";
// reformat the Date values so they are deserialized properly by ASP.NET JSON Deserializer
jsonString = jsonString.replace(/\/Date\((-?[0-9]+)\)\//g, "\\/Date($1)\\/");
$.ajax({
type: "POST",
url: "InvoiceDetails.aspx/SaveInvoiceLineItem",
data: jsonString,
contentType: "application/json; charset=utf-8",
dataType: "json"
});
服務器方法簽名如下所示:
[WebMethod]
public static void SaveInvoiceLineItem(InvoiceLineBO invoiceLine)
{
用另一個屬性裝飾你的 [WebMethod] :
[ScriptMethod(ResponseFormat = ResponseFormat.Json)]
我相信這是在 System.Web.Services.Scripting 中......
這是您定義數據的方式 (JSON)
data: { 'items': items },
這是應該的方式
data: '{ items: " '+items +' "}',
基本上你是在序列化參數。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.