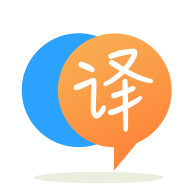
[英](javafx) I'm currently making an app which requires people to create an account, how can i make sure the texts fields aren't null?
[英]I'm not sure how to get these values given in different classes to connect to each other in Javafx for a GUI I'm making
這是 Javafx,我正在制作一個包含多個類的 GUI。
代碼:
import javafx.application.Application;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.GridPane;
import javafx.scene.layout.HBox;
import javafx.stage.Stage;
public class AutoLoanCalculator extends Application{
public static void main(String[] args) {
launch(args);
}
public void start(Stage primaryStage) {
PaymentSection section1 = new PaymentSection();
LoanTermSection section2 = new LoanTermSection();
FinancingSection section3 = new FinancingSection();
OptionsSection section4 = new OptionsSection();
Button calc = new Button("Calculate");
Button reset = new Button("Reset");
Button exit = new Button("Exit");
GridPane comboGrid = new GridPane();
comboGrid.add(section1, 0, 0);
comboGrid.add(section2, 1, 0);
comboGrid.add(section3, 0, 1);
comboGrid.add(section4, 1, 1);
comboGrid.add(calc, 0,2);
comboGrid.add(reset, 1,2);
comboGrid.add(exit, 2,2);
HBox combo = new HBox();
combo.getChildren().add(comboGrid);
calc.setOnAction(event ->{
Double basePrice = Double.parseDouble(section3.getPriceNum());
Double downPayment = Double.parseDouble(section3.getDownPayNum());
Double taxRate = Double.parseDouble(section3.getTaxNum()) / 100;
Double optionPrice = section4.getCost();
Double interestRate = section2.getRate() / 100;
int loanTermMonths = section2.getMonth();
//calculates the values of rate and total sales tax
double rate = interestRate/12;
double salesTaxAmt = (basePrice - downPayment + optionPrice) * taxRate;
Double totalLoanAmt = basePrice - downPayment + optionPrice + salesTaxAmt;
Double monthPayment = totalLoanAmt * (rate * Math.pow(1 + rate, loanTermMonths)) / (Math.pow(1 + rate, loanTermMonths) - 1);
Double totalPayment = monthPayment * loanTermMonths + downPayment;
//section1.setLoanNum(String.format("%.2f", totalLoanAmt));
System.out.println(basePrice);
System.out.println(downPayment);
System.out.println(taxRate);
});
reset.setOnAction(event ->{
section1.reset();
section2.reset();
section3.reset();
section4.reset();
});
exit.setOnAction(event ->{
primaryStage.close();
});
Scene scene = new Scene(combo);
primaryStage.setTitle("Auto Loan Calculator");
primaryStage.setScene(scene);
primaryStage.show();
}
}
//我稱這個部分為1
import javafx.scene.control.Label;
import javafx.scene.layout.GridPane;
public class PaymentSection extends GridPane{
private Label payInfo;
private Label loanAmount;
private Label monthlyPayment;
private Label totalPayment;
private Label loanNum;
private Label monthNum;
private Label totalNum;
private String loanInt;
private String monthInt;
private String totalInt;
public PaymentSection () {
payInfo = new Label("Payment Information");
loanAmount = new Label("Total Loan Amount: $ ");
monthlyPayment = new Label("Monthly Payment: $ ");
totalPayment = new Label("Total Payment: $ ");
loanNum = new Label();
monthNum = new Label();
totalNum = new Label();
loanNum.setText("0.0");
monthNum.setText("0.0");
totalNum.setText("0.0");
add(payInfo,0,0);
add(loanAmount,0,1);
add(monthlyPayment,0,2);
add(totalPayment,0,3);
add(loanNum,1,1);
add(monthNum,1,2);
add(totalNum,1,3);
setStyle("-fx-padding: 10;" +
"-fx-border-style: solid inside;" +
"-fx-border-width: 2;" +
"-fx-border-color: black;");
}
public String setLoanNum(String string) {
return loanInt;
}
public void reset() {
loanNum.setText("0.0");
monthNum.setText("0.0");
totalNum.setText("0.0");
}
}
//我稱這個部分為 3
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.GridPane;
public class FinancingSection extends GridPane{
private Label finance;
private Label price;
private Label tax;
private Label downPay;
private TextField priceNum;
private TextField taxNum;
private TextField downPayNum;
private String priceInt;
private String downPayInt;
private String taxInt;
public FinancingSection() {
LoanTermSection term = new LoanTermSection();
term.getRate();
finance = new Label("Fianancing Information");
price = new Label("Base Price: $ ");
downPay = new Label("Down Payment: $ ");
tax = new Label("Sales Tax: % ");
priceNum = new TextField("0.0");
downPayNum = new TextField("0.0");
taxNum = new TextField("7.0");
priceInt = priceNum.getText();
downPayInt = downPayNum.getText();
taxInt = taxNum.getText();
add(finance,0,0);
add(price,0,1);
add(downPay,0,2);
add(tax,0,3);
add(priceNum,1,1);
add(downPayNum,1,2);
add(taxNum,1,3);
setStyle("-fx-padding: 10;" +
"-fx-border-style: solid inside;" +
"-fx-border-width: 2;" +
"-fx-border-color: black;");
}
public String getPriceNum() {
return priceInt;
}
public void setPriceNum(String newPriceNum) {
priceInt = newPriceNum;
}
public String getDownPayNum() {
return downPayInt;
}
public void setDownPayNum(String newDownPay) {
downPayInt = newDownPay;
}
public String getTaxNum() {
return taxInt;
}
public void setTaxNum(String newTax) {
taxInt = newTax;
}
public void reset() {
priceNum.setText("0.0");
downPayNum.setText("0.0");
taxNum.setText("7.0");
}
}
問題是我無法設置 basePrice、downPayment 和 taxRate - 我無法從第 3 節代碼中設置的值中獲取它們:我想使用 setter 和 getter 但它似乎不起作用而且我是不知道為什么或如何解決它。- 也許這與我將它們設置為的價值觀有關 - 真的不確定。 至於第 1 節,我無法打印它——我假設這與第 3 節是同一個問題。所以主要是我只需要幫助獲取第 3 節的值。 提前致謝!
您需要在檢索值時對文本字段調用getText()
。 所以,例如,
public class FinancingSection extends GridPane{
// ...
public String getPriceNum() {
return priceNum.getText();
}
// ...
}
您可能可以刪除priceInt
變量等,並且您可能應該刪除FinancingSection
中未使用的設置方法。
您在PaymentSection
中使用的設置方法應更新其相應的標簽,例如
public class PaymentSection extends GridPane{
// ...
public void setLoanNum(String string) {
loanNum.setText(string) ;
}
// ...
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.