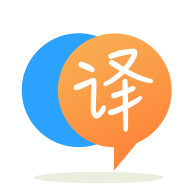
[英]How can you read data from columns in a text file into 3 1D numpy arrays in python
[英]How do I read 1 dimensional data from a text file into a 1D array? [Python]
我有一個文本文件 DATALOG1.TXT,其值從 0 到 1023,與信號有關。 該文件每行有一個值,所有值都存儲在一列中。 這是第一個值如何存儲在文件中的示例:
0
0
576
0
643
60
0
1012
0
455
69
0
1023
0
258
我有以下代碼,它只輸出 0 到 9 之間的“幅度”值,就像標准化一樣。 這是我的代碼:
import matplotlib.pyplot as plt
import numpy as np
values = [] #array to be filled
#reading data from the file into the array
f = open('DATALOG1.TXT', 'r')
for line in f:
values.append(line[0])
#creating another array of the same size to plot against
time = np.arange(0, len(values), 1)
plt.bar(time, values, color='g', label='File Data')
plt.xlabel('Time', fontsize=12)
plt.ylabel('Magnitude', fontdsize=12)
plt.title('Magnitude changes', fontsize=20)
plt.show()
為什么 output 介於 0 和 9 之間,而不是顯示文本文件中的值(介於 0 和 1023 之間)?
你的問題出在這里:
for line in f:
values.append(line[0])
你 append 是每行的第一個字符到你的列表values
。 列表values
包含整行,包括行尾。 如果你要使用
for line in f:
values.append(line.strip())
你應該更接近你的最終答案。
通過使用line[0]
,您只需 append 將每行的第一個字符添加到列表中。
由於您已經使用了 numpy,因此您可以使用genfromtxt
加載數據文件。
您的代碼將變為:
import matplotlib.pyplot as plt
import numpy as np
values = [] #array to be filled
#reading data from the file into the array
values = np.genfromtxt('DATALOG1.TXT')
#creating another array of the same size to plot against
time = np.arange(0, len(values), 1)
plt.bar(time, values, color='g', label='File Data')
plt.xlabel('Time', fontsize=12)
plt.ylabel('Magnitude', fontsize=12)
plt.title('Magnitude changes', fontsize=20)
plt.show()
我建議閱讀完整的文件,拆分每個換行符\n
的值(我為此使用了列表理解)並將字符串轉換為整數。 此外,如果您使用range
而不是 numpys arange
,則可以完全避免使用 numpy。
import matplotlib.pyplot as plt
#reading data from the file into the array
data = open('DATALOG1.TXT', 'r').read()
values = [int(f) for f in data.split("\n")]
time = range(len(values))
plt.bar(time, values, color='g', label='File Data')
plt.xlabel('Time', fontsize=12)
plt.ylabel('Magnitude', fontdsize=12)
plt.title('Magnitude changes', fontsize=20)
plt.show()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.