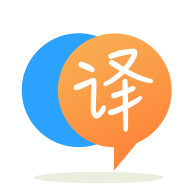
[英]How to get the current CPU/RAM/Disk usage in a C# web application using .NET CORE?
[英]How to get current CPU and RAM usage, and also how much is available in C# .NET core without looping over all processes?
C# 中是否有任何方法可以使用當前內存量和可用內存量,而無需遍歷所有進程並添加每個 workingset64 值?
好吧,你可以這樣做:
public class MemoryMetrics
{
public double Total;
public double Used;
public double Free;
}
public class MemoryMetricsService : IMemoryMetricsService
{
public IRuntimeInformationService RuntimeInformationService { get; set; }
public MemoryMetricsService(IRuntimeInformationService runtimeInformationService)
{
this.RuntimeInformationService = runtimeInformationService;
}
public MemoryMetrics GetMetrics()
{
if (RuntimeInformationService.IsUnix())
{
return GetUnixMetrics();
}
return GetWindowsMetrics();
}
private MemoryMetrics GetWindowsMetrics()
{
var output = "";
var info = new ProcessStartInfo();
info.FileName = "wmic";
info.Arguments = "OS get FreePhysicalMemory,TotalVisibleMemorySize /Value";
info.RedirectStandardOutput = true;
using (var process = Process.Start(info))
{
output = process.StandardOutput.ReadToEnd();
}
var lines = output.Trim().Split("\n");
var freeMemoryParts = lines[0].Split("=", StringSplitOptions.RemoveEmptyEntries);
var totalMemoryParts = lines[1].Split("=", StringSplitOptions.RemoveEmptyEntries);
var metrics = new MemoryMetrics();
metrics.Total = Math.Round(double.Parse(totalMemoryParts[1]) / 1024, 0);
metrics.Free = Math.Round(double.Parse(freeMemoryParts[1]) / 1024, 0);
metrics.Used = metrics.Total - metrics.Free;
return metrics;
}
private MemoryMetrics GetUnixMetrics()
{
var output = "";
var info = new ProcessStartInfo("free -m");
info.FileName = "/bin/bash";
info.Arguments = "-c \"free -m\"";
info.RedirectStandardOutput = true;
using (var process = Process.Start(info))
{
output = process.StandardOutput.ReadToEnd();
Console.WriteLine(output);
}
var lines = output.Split("\n");
var memory = lines[1].Split(" ", StringSplitOptions.RemoveEmptyEntries);
var metrics = new MemoryMetrics();
metrics.Total = double.Parse(memory[1]);
metrics.Used = double.Parse(memory[2]);
metrics.Free = double.Parse(memory[3]);
return metrics;
}
}
至於IRuntimeInformationService
:
using System.Runtime.InteropServices;
public class RuntimeInformationService : IRuntimeInformationService
{
public OSPlatform GetOsPlatform()
{
if(RuntimeInformation.IsOSPlatform(OSPlatform.OSX)) return OSPlatform.OSX;
if(RuntimeInformation.IsOSPlatform(OSPlatform.Linux)) return OSPlatform.Linux;
if(RuntimeInformation.IsOSPlatform(OSPlatform.FreeBSD)) return OSPlatform.FreeBSD;
if(RuntimeInformation.IsOSPlatform(OSPlatform.Windows)) return OSPlatform.Windows;
return OSPlatform.Create("Unknown");
}
public bool IsUnix()
{
var isUnix = RuntimeInformation.IsOSPlatform(OSPlatform.OSX) ||
RuntimeInformation.IsOSPlatform(OSPlatform.Linux);
return isUnix;
}
public bool IsWindows()
{
return RuntimeInformation.IsOSPlatform(OSPlatform.Windows);
}
public bool IsLinux()
{
return RuntimeInformation.IsOSPlatform(OSPlatform.Linux);
}
public bool IsFreeBSD()
{
return RuntimeInformation.IsOSPlatform(OSPlatform.FreeBSD);
}
public bool IsOSX()
{
return RuntimeInformation.IsOSPlatform(OSPlatform.OSX);
}
public string GetRuntimeIdentifier()
{
return RuntimeInformation.RuntimeIdentifier;
}
public Architecture GetProcessArchitecture()
{
return RuntimeInformation.ProcessArchitecture;
}
public Architecture GetOSArchitecture()
{
return RuntimeInformation.OSArchitecture;
}
public string GetOSDescription()
{
return RuntimeInformation.OSDescription;
}
public string GetFrameworkDescription()
{
return RuntimeInformation.FrameworkDescription;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.