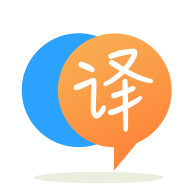
[英]405 method not allowed error in AWS Cognito oauth2/token endpoint
[英]How to configure Spring Boot to authenticate Web-app users and REST clients using AWS Cognito (OAuth2/OIDC)
我需要配置 Spring 引導服務器以使用 AWS Cognito 用戶池對 Web 用戶和 REST 客戶端進行身份驗證:
Authorization: Bearer...
header。問題是:
讓我們從術語開始:
Spring 的spring-security-oauth2-client
模塊負責“授權碼授予流程”, spring-security-oauth2-resource-server
模塊負責“客戶端憑證流程”。
為了同時使用這兩種流程/方法,我們需要告訴 spring 如何確定對傳入的 HTTP 請求使用哪種身份驗證方法。 正如https://stackoverflow.com/a/64752665/2692895中所解釋的,這可以通過查找Authorization: bearer...
header 來完成:
Authorization
header,則假定其為 REST 客戶端並使用“客戶端憑據流”。我正在使用 Spring-Boot 2.6.6(Spring 5.6.2)。
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-oauth2-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-oauth2-jose</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-oauth2-resource-server</artifactId>
</dependency>
application.yaml
spring:
security:
oauth2:
# Interactive/web users authentication
client:
registration:
cognito:
clientId: ${COGNITO_CLIENT_ID}
clientSecret: ${COGNITO_CLIENT_SECRET}
scope: openid
clientName: ${CLIENT_APP_NAME}
provider:
cognito:
issuerUri: https://cognito-idp.eu-central-1.amazonaws.com/${COGNITO_POOL_ID}
user-name-attribute: email
# REST API authentication
resourceserver:
jwt:
issuer-uri: https://cognito-idp.eu-central-1.amazonaws.com/${COGNITO_POOL_ID}
交互式/網絡用戶身份驗證:
@Configuration
@EnableWebSecurity
@EnableGlobalMethodSecurity(
// Needed for method access control via the @Secured annotation
prePostEnabled = true,
jsr250Enabled = true,
securedEnabled = true
)
@Profile({"cognito"})
@Order(2)
public class CognitoSecurityConfiguration extends WebSecurityConfigurerAdapter {
@SneakyThrows
@Override
protected void configure(HttpSecurity http) {
http
// TODO disable CSRF because when enabled controllers aren't initialized
// and if they are, POST are getting 403
.csrf().disable()
.authorizeRequests()
.anyRequest().authenticated()
.and()
.oauth2Client()
.and()
.logout()
.and()
.oauth2Login()
.redirectionEndpoint().baseUri("/login/oauth2/code/cognito")
.and()
;
}
}
REST 客戶端認證:
/**
* Allow users to use a token (id-token, jwt) instead of the interactive login.
* The token is specified as the "Authorization: Bearer ..." header.
* </p>
* To get a token, the cognito client-app needs to support USER_PASSWORD_AUTH then use the following command:
* <pre>
* aws cognito-idp initiate-auth --auth-flow USER_PASSWORD_AUTH --output json \
* --region $region --client-id $clientid --auth-parameters "USERNAME=$username,PASSWORD=$password" \
* | jq .AuthenticationResult.IdToken
* </pre>
*/
@Slf4j
@Configuration
@Profile({"cognito"})
@Order(1)
public class CognitoTokenBasedSecurityConfiguration extends WebSecurityConfigurerAdapter {
@SneakyThrows
@Override
protected void configure(HttpSecurity http) {
http
.requestMatcher(new RequestHeaderRequestMatcher("Authorization"))
.authorizeRequests().anyRequest().authenticated()
.and().oauth2ResourceServer().jwt()
;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.