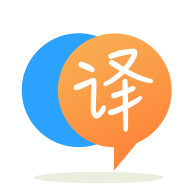
[英]How to Convert a String ArrayList to a Double ArrayList and calculate the sum of values?
[英]How to convert a arraylist <String> to arrayList <Integer> and Calculate the values of Integers on it?
我應該如何將 ArrayList 字符串從 Listview 值計算為 ArrayList Integer 並返回 Listview,因為我一直在申請 Android 計算來自 ArrayList 的 Listview 項目。這是最讓我煩惱的一個,它將最后的 arrayList 值顯示為 textView,而不是計算它們
public class Menu extends AppCompatActivity {
public FloatingActionButton fab1, fab2, fab3;
public Animation fab_open, fab_close, rotate_cw, rotate_ccw;
public EditText editText, editText2, editText3, editText4;
public TextView textView1;
public ListView listView;
public ArrayList<String> arrayList;
public ArrayAdapter<String> arrayAdapter;
public boolean isOpen = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_menu);
fab1 = findViewById(R.id.fab_1);
fab2 = findViewById(R.id.fab_2);
fab3 = findViewById(R.id.fab_3);
textView1 = findViewById(R.id.info_text4);
listView = findViewById(R.id.listview_items);
fab_open = AnimationUtils.loadAnimation(getApplicationContext(), R.anim.fab_open);
fab_close = AnimationUtils.loadAnimation(getApplicationContext(), R.anim.fab_close);
rotate_cw = AnimationUtils.loadAnimation(getApplicationContext(), R.anim.rotate_cw);
rotate_ccw = AnimationUtils.loadAnimation(getApplicationContext(), R.anim.rotate_ccw);
loadData();
calculate();
arrayAdapter = new ArrayAdapter<>(getApplicationContext(), android.R.layout.simple_list_item_1, arrayList);
listView.setAdapter(arrayAdapter);
fab1.setOnClickListener(view -> {
if (isOpen) {
fab2.startAnimation(fab_close);
fab3.startAnimation(fab_close);
fab1.startAnimation(rotate_cw);
fab2.setClickable(true);
fab3.setClickable(true);
isOpen = false;
} else {
fab2.startAnimation(fab_open);
fab3.startAnimation(fab_open);
fab1.startAnimation(rotate_ccw);
fab2.setClickable(true);
fab3.setClickable(true);
isOpen = true;
}
});
// Budget
fab2.setOnClickListener(view -> {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("Add Budget");
builder.setIcon(R.drawable.ic_wallet_budget);
final View customLayout = getLayoutInflater().inflate(R.layout.add_budget_popup, null);
builder.setView(customLayout);
builder.setPositiveButton("OK", (dialog, which) -> {
// Budget Name
editText = customLayout.findViewById(R.id.editText);
// Budget Input
editText2 = customLayout.findViewById(R.id.editText2);
// Conditional Statements
if(TextUtils.isEmpty(editText.getText().toString()) && TextUtils.isEmpty(editText2.getText().toString())) {
Toast toast = Toast.makeText(getApplicationContext(), "No Budget Name Data and No Budget Data been added", Toast.LENGTH_SHORT);
toast.show();
} else if(TextUtils.isGraphic(editText.getText().toString()) && TextUtils.isEmpty(editText2.getText().toString())){
Toast toast = Toast.makeText(getApplicationContext(), "Budget Name Data has a input, but No Budget Data input", Toast.LENGTH_SHORT);
toast.show();
} else if(TextUtils.isEmpty(editText.getText().toString()) && TextUtils.isDigitsOnly(editText2.getText().toString())){
Toast toast = Toast.makeText(getApplicationContext(), "Budget Data input has a input, but No Budget Name Data input", Toast.LENGTH_SHORT);
toast.show();
} else {
arrayList.add(editText2.getText().toString());
arrayAdapter.notifyDataSetChanged();
Toast toast = Toast.makeText(getApplicationContext(), "Budget Data Added", Toast.LENGTH_SHORT);
toast.show();
saveData();
}
});
AlertDialog dialog = builder.create();
dialog.show();
dialog.setCancelable(false);
});
// Expense
fab3.setOnClickListener(view -> {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("Add Expense");
builder.setIcon(R.drawable.ic_money_exp);
final View customLayout = getLayoutInflater().inflate(R.layout.add_expense_popup, null);
builder.setView(customLayout);
builder.setPositiveButton("OK", (dialog, which) -> {
// Expense Name
editText3 = customLayout.findViewById(R.id.editText3);
// Expense Input
editText4 = customLayout.findViewById(R.id.editText4);
// Conditional Statements
if(TextUtils.isEmpty(editText3.getText().toString()) && TextUtils.isEmpty(editText4.getText().toString())) {
Toast toast = Toast.makeText(getApplicationContext(), "No Expense Name Data and No Expense Data been added", Toast.LENGTH_SHORT);
toast.show();
} else if(TextUtils.isGraphic(editText3.getText().toString()) && TextUtils.isEmpty(editText4.getText().toString())){
Toast toast = Toast.makeText(getApplicationContext(), "Expense Name Data has a input, but No Expense Data input", Toast.LENGTH_SHORT);
toast.show();
} else if(TextUtils.isEmpty(editText3.getText().toString()) && TextUtils.isDigitsOnly(editText4.getText().toString())){
Toast toast = Toast.makeText(getApplicationContext(), "Expense Data input has a input, but No Expense Name Data input", Toast.LENGTH_SHORT);
toast.show();
} else {
arrayList.add(editText4.getText().toString());
arrayAdapter.notifyDataSetChanged();
Toast toast = Toast.makeText(getApplicationContext(), "Expense Data Added", Toast.LENGTH_SHORT);
toast.show();
saveData();
}
});
AlertDialog dialog = builder.create();
dialog.show();
dialog.setCancelable(false);
});
}
private void saveData() {
SharedPreferences sharedPreferences = getSharedPreferences("SHAREDPREFS", MODE_PRIVATE);
SharedPreferences.Editor editor = sharedPreferences.edit();
Gson gson = new Gson();
String json = gson.toJson(arrayList);
editor.putString("Test", json);
editor.apply();
}
private void loadData(){
SharedPreferences sharedPreferences = getSharedPreferences("SHAREDPREFS", MODE_PRIVATE);
Gson gson = new Gson();
String json = sharedPreferences.getString("Test", null);
Type type = new TypeToken<ArrayList<String>>(){}.getType();
arrayList = gson.fromJson(json, type);
if(arrayList == null){
arrayList = new ArrayList<>();
}
}
private void calculate(){
for (int i = 0; i < arrayList.size(); i++) {
textView1.setText(String.valueOf(arrayList.get(i)));
}
}
@Override
public boolean onCreateOptionsMenu(android.view.Menu menu){
getMenuInflater().inflate(R.menu.list, menu);
return true;
}
@SuppressLint("NonConstantResourceId")
@Override
public boolean onOptionsItemSelected(MenuItem items){
switch (items.getItemId()){
case R.id.items:
// Email Feedback System
Intent intent=new Intent(Intent.ACTION_SEND);
String[] recipients={"companyenode@gmail.com"};
intent.putExtra(Intent.EXTRA_EMAIL, recipients);
intent.putExtra(Intent.EXTRA_SUBJECT,"Subject text here...");
intent.putExtra(Intent.EXTRA_TEXT,"Body of the content here...");
intent.putExtra(Intent.EXTRA_CC,"mailcc@gmail.com");
intent.setType("text/html");
intent.setPackage("com.google.android.gm");
startActivity(Intent.createChooser(intent, "Send mail"));
case R.id.items3:
// About App
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("About");
builder.setIcon(R.drawable.ic_baseline_info_24);
builder.setMessage("Our app allows you to monitor and categorize your expenses across different bank and investment accounts and credit cards, our app also offer budgeting tools, credit monitoring, mileage tracking, receipt keeping, and advice to grow your net worth.");
builder.setCancelable(false);
builder.setPositiveButton("Close", (dialog, id) -> dialog.cancel());
AlertDialog alert = builder.create();
alert.show();
default:
return super.onOptionsItemSelected(items);
}
}
@Override
public void onBackPressed() {
new AlertDialog.Builder(this).
setIcon(android.R.drawable.ic_dialog_alert)
.setTitle("Exit")
.setMessage("Are you sure you want to close app?")
.setPositiveButton("Yes", (dialog, which) -> finish())
.setNegativeButton("No", null)
.show();
}
}
那是我的問題,我的代碼有問題嗎?
問題出在這個function
private void calculate(){
for (int i = 0; i < arrayList.size(); i++) {
textView1.setText(String.valueOf(arrayList.get(i)));
}
}
您正在遍歷數組列表並按順序將文本設置為每個數組列表條目。 這意味着它將覆蓋之前的任何文本並最終只顯示最后一個條目。 如果您的數組列表是["1","2","3"]
這與調用相同
testView1.setText("1");
testView1.setText("2"); // overrides the "1"
testView1.setText("3"); // overrides the "2"
// ends with it showing "3"
如果你想顯示所有數組條目的總和,你應該首先計算總和,然后顯示它,如下所示:
private void calculate() {
// first calculate the total
int total = 0;
for (int i = 0; i < arrayList.size(); i++) {
total += Integer.parseInt(arrayList.get(i));
}
// then display it
textView1.setText(String.valueOf(total));
}
如果數組列表中的任何數字不是整數,當您調用parseInt
時,這將拋出NumberFormatException
,因此如果有可能,您應該添加一些異常處理
private void calculate() {
int total = 0;
for (int i = 0; i < arrayList.size(); i++) {
try {
total += Integer.parseInt(arrayList.get(i));
} catch( NumberFormatException e) {
e.printStackTrace();
}
}
textView1.setText(String.valueOf(total));
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.