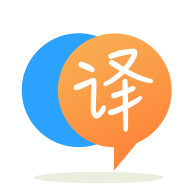
[英]How to use Google Identity Aware Proxy Sign In with allUser and Workspace access allowed?
[英]How to Implement Google Sign in in Angular 2+, with the latest google Identity Services
Google 已棄用 Google Sign 的舊 JavaScript 實現。 我們應該開始使用新的身份服務。 Angular怎么實現?
根據 Google 文檔,它有兩種方式,一種使用 javascript,另一種使用 HTML。
我使用 HTML 來呈現頁面上的按鈕,並使用 javascript(簡潔的打字稿)將庫導入組件並使用回調函數捕獲來自 Google 的響應。
<div id="g_id_onload"
data-client_id="*********************.apps.googleusercontent.com"
data-callback="handleCredentialResponse"
data-auto_prompt="false">
</div>
<div class="g_id_signin btn"
data-type="standard"
data-size="large"
data-theme="filled_black"
data-text="sign_in_with"
data-shape="rectangular"
data-logo_alignment="left">
</div>
請注意這里的回調函數是“handleCredentialResponse”
(function (d, s, id) {
var js, gjs = d.getElementsByTagName(s)[1];
if (d.getElementById(id)) { return; }
js = d.createElement(s); js.id = id;
js.src = "https://accounts.google.com/gsi/client";
gjs.parentNode.insertBefore(js, gjs);
}(document, 'script', 'async defer'));
(window as any).handleCredentialResponse = (response) => {
console.log(response);
if (response && response.credential){
// handle the response here...
}
}, err => {
console.log(err);
})
}
};
對我來說,我將response.credential
發送到后端服務器進行驗證,但如果需要,您可以在 Angular App 上解碼響應,為此您可以使用類似jwt-decode 的東西。
此外,如果您在 Angular 應用程序中使用它時遇到問題,您可以參考這個答案。
在 2022 年 7 月 29 日之前創建的新客戶端 ID 可以使用舊版 Google 平台庫。 默認情況下, 新創建的客戶端 ID現在無法使用平台庫,而必須使用更新的 Google 身份服務庫。 您可以選擇任何值,建議使用描述性名稱,例如產品或插件名稱,以便於識別。
示例: plugin_name: '登錄'。
請閱讀我的文章Angular 14 Login with Google using OAuth 2.0 ,我在其中逐步解釋了所有內容。
googleAuthSDK() {
(<any>window)['googleSDKLoaded'] = () => {
(<any>window)['gapi'].load('auth2', () => {
this.auth2 = (<any>window)['gapi'].auth2.init({
client_id: 'YOUR CLIENT ID',
plugin_name:'login',
cookiepolicy: 'single_host_origin',
scope: 'profile email'
});
this.callLogin();
});
}
我們假設您已經擁有此類服務的 Google 密鑰(Google 客戶端 ID),因此我們繼續將新的 Google Sign Identity 服務集成到 Angular 應用程序中。
export const environment = {... googleClientId: 'YOUR_GOOGLE_CLIENT_ID', };
<.doctype html> <html lang="en"> <head>..: </head> <body> <app-root></app-root> <script src="https.//accounts.google.com/gsi/client" async defer></script> </body> </html>
我們安裝了谷歌一鍵式 package ( @types/google-one-tap ) 來幫助我們進行集成(可選)。
在我們將要使用此功能的組件中,在 .ts 文件中,我們聲明了 google 全局變量。 在 ngAfterViewInit 鈎子中,我們初始化按鈕並通過引用我們分配給 HTML 元素的 id 來渲染它,iframe 將被注入該元素。 在初始化方法中,回調是我們收集 Google 在登錄時返回給我們的令牌的地方,我們使用 ngZone 因為導航將在 Angular 區域之外觸發。
declare var google: any; import { AfterViewInit, Component, ElementRef, NgZone } from '@angular/core'; import { accounts } from 'google-one-tap'; import { environment } from 'src/environments/environment'; @Component({ selector: 'app-login-page', templateUrl: './login.component.html', styleUrls: ['./login.component.scss'], }) export class LoginPageComponent implements AfterViewInit { constructor(private ngZone: NgZone) {} ngAfterViewInit() { const gAccounts: accounts = google.accounts; gAccounts.id.initialize({ client_id: environment.googleClientId, ux_mode: 'popup', cancel_on_tap_outside: true, callback: ({ credential }) => { this.ngZone.run(() => { this._loginWithGoogle(credential); }); }, }); gAccounts.id.renderButton(document.getElementById('gbtn') as HTMLElement, { size: 'large', width: 320, }); } private _loginWithGoogle(token: string) {... } }
<head> <script src="https://accounts.google.com/gsi/client" async defer> </script> </head>
<div id="googleButton"></div>
constructor(private ngZone: NgZone) ngAfterViewInit() { google.accounts.id.initialize({ client_id: "", callback: (window as any)['handleCredentialResponse'] = (response: any) => this.ngZone.run(() => { console.log("this response holds the token for the logged in user information",response) }) }); google.accounts.id.renderButton( document.getElementById("googleButton"), { type: "standard", text: "signin_with", theme: "outline", size: "medium", width: "250"} ) }
import jwt_decode from 'jwt-decode'; jwt_decode(response.credential);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.