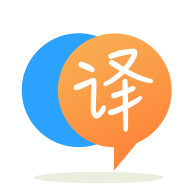
[英]C++ Template : Choosing overloaded functions using implicit conversion to template instantiated type!
[英]implicit type conversion in C++ using overloaded =
在下面的代碼中(這是一個類型轉換的例子):
// implicit conversion of classes:
#include <iostream>
using namespace std;
class A {};
class B {
public:
// conversion from A (constructor):
B (const A& x) {}
// conversion from A (assignment):
B& operator= (const A& x) {return *this;}
// conversion to A (type-cast operator)
operator A() {return A();}
};
int main ()
{
A foo;
B bar = foo; // calls constructor
bar = foo; // calls assignment
foo = bar; // calls type-cast operator
return 0;
}
在第 21 行: bar = foo; // calls assignment
bar = foo; // calls assignment
它調用重載的運算符 =。 並且在“=”運算符的類重載主體中,第 12 行編寫了: B& operator= (const A& x) {return *this;}
它的語句是“return this”。 這里指點一下吧。 並且只有一個 A 類類型的參數: const A& x
這個函數對這個參數有什么作用? 在第 21 行中,通過寫入將什么轉換為什么: bar = foo;
?
您可能要問的真正問題是 operator= 函數的用途是什么?
通常我們從常量引用中獲取一些數據 例如
struct A{
std::string data;
}
struct B{
std::string differentData;
B& operator=(const A& other){
differentData = other.data;
return *this;
}
}
然后,如果我們調用代碼
int main(){
A a;
a.data = "hello";
B b;
b = a;
std::cout << b.differentData;
}
我們應該將“hello”打印到控制台
由於您沒有與函數中給出的類進行交互,因此這些代碼所做的只是運行一個空函數。
僅當您將相等的調用鏈接在一起時才使用返回
int main(){
B b1, b2, b3;
b3.differentData = "hi";
b1 = b2 = b3;
//Pushes hi from b3 >> b2 and returns b2
//Pushes hi from b2 >> b1 and returns b1
}
這個函數用這個參數做什么?
沒有什么。
通過寫入將什么轉換為什么:
bar = foo;
沒有什么。 或者等價地, bar
的狀態被foo
的狀態替換。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.