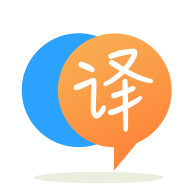
[英]In JavaScript, how can I ignore blank lines when converting csv to json
[英]How to dynamically ignore certain fields in a javascript object when converting it to json?
目前,我在方法上使用toJSON()
對象來忽略任何帶下划線的字段,例如
toJSON() {
const properties = Object.getOwnPropertyNames(this);
const publicProperties = properties.filter(property => {
return property.charAt(0) !== '_'
})
const json = publicProperties.reduce((obj, key) => {
obj[key] = this[key]
return obj
}, {})
return json
}
這很好。 但是我的 API 中有角色的概念,如果用戶是管理員,我想返回私有字段。
這讓我想到了這樣做:
toJSON(role='user') {
const properties = Object.getOwnPropertyNames(this);
const publicProperties = properties.filter(property => {
return property.charAt(0) !== '_' || role === 'admin'
})
const json = publicProperties.reduce((obj, key) => {
key = key.charAt(0) === '_' ? key.substring(1) : key
obj[key] = this[key]
return obj
}, {})
return json
}
但是接下來的問題就變成了如何將角色參數傳遞給toJSON()
方法,尤其是在調用 JSON.stringify( JSON.stringify()
並且調用JSON.stringify()
的方法時,我可能無法訪問。
在返回 json 響應之前,我可以在我的對象上設置一個role
屬性,例如
const getCurrentProject = async (c) => {
const project = await projectService.getCurrentProject(c.get('projectId'));
project._role = c.get('payload').role
return c.json(project, httpStatus.OK);
};
但這似乎並不理想,然后在對象數組上調用JSON.stringify()
時會出現更多問題,因為我必須為每個對象設置它。
我的下一個想法是使用我自己的 json 響應函數,該函數將具有JSON.stringify()
的替換函數
const jsonResponse = (context, object, status) => {
const role = c.get('payload').role
const body = JSON.stringify(object, (key, value) => {
// function to set private vars to null based on role
})
headers = 'application/json; charset=UTF-8'
return c.body(body, status, headers)
}
這樣做的問題是替換函數只會將它們設置為 null 而不會隱藏它們,而且我不能盲目地刪除具有 null 值的鍵,因為我可能需要它們。 我可以將它們設置為'remove'
或其他占位符,然后再刪除它們,但這似乎不是最好的方法。
所以目前我對我應該做什么感到困惑。 有沒有辦法全局覆蓋JSON.stringify()
並將角色參數添加為參數,我是否缺少更好的方法? 或者我應該堅持使用_role
屬性,並為對象列表為每個對象設置它。
謝謝!
您可以使用替換功能。 如果您返回Function
、 Symbol
或undefined
,則該屬性不包含在輸出中。 請參閱https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/JSON/stringify#the_replacer_parameter
我會使用Object.entries
,然后使用Array.filter
你想要的鍵,最后使用Object.fromEntries
來返回一個對象。
示例->
const obj = { _adminOnly: 'Lets have a party!', name: 'bob', age: 22, _hideme: 'Hide unless admin', toJSON: function (role='user') { return Object.fromEntries( Object.entries(this). filter(([k]) => { if (k === 'toJSON') return false; return role === 'admin' ? true : k.charAt(0) !== '_' } ) ); } } console.log(obj.toJSON()); console.log(obj.toJSON('admin'));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.