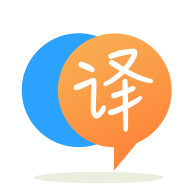
[英]How to upload image in CkEditor in ASP.NET Core MVC project?
[英]How to upload an image with ASP.NET Core 5.0 MVC
我想在 post 方法中上傳圖像以編輯記錄,這是將數據發送到控制器的表單:
<form asp-action="Edit">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<input type="hidden" asp-for="IdEmpresa" />
<div class="form-group mt-3">
<label asp-for="CodEmpresa" class="control-label"></label>
<input required asp-for="CodEmpresa" class="form-control" />
<span asp-validation-for="CodEmpresa" class="text-danger"></span>
</div>
<div class="form-group mt-3">
<label asp-for="Empresa" class="control-label"></label>
<input required asp-for="Empresa" class="form-control" />
<span asp-validation-for="Empresa" class="text-danger"></span>
</div>
<div class="form-group mt-3">
<label asp-for="Contacto" class="control-label"></label>
<input required asp-for="Contacto" class="form-control" />
<span asp-validation-for="Contacto" class="text-danger"></span>
</div>
<div class="form-group mt-3">
<label asp-for="Imagen" class="control-label"></label>
<input asp-for="Imagen" class="form-control" type="file" />
<span asp-validation-for="Imagen" class="text-danger"></span>
</div>
<br />
<div class="form-group d-flex justify-content-end">
<input type="submit" value="Actualizar" class="btn btn-primary bg-primary" />
</div>
</form>
這里是更新數據但只更新字符串或數字的方法,我不知道如何處理 type = 'file' 的輸入以將圖像復制到本地目錄並將路徑分配給'Image ' 模型中的屬性
public async Task<IActionResult> Edit(int id, [Bind("IdEmpresa,CodEmpresa,Empresa,Contacto")] MnEmpresa mnEmpresa)
{
if (id != mnEmpresa.IdEmpresa)
{
return NotFound();
}
if (ModelState.IsValid)
{
try
{
MnEmpresa empresa = _context.MnEmpresas.Where(x => x.IdEmpresa == mnEmpresa.IdEmpresa).FirstOrDefault();
_context.Entry(empresa).State = EntityState.Detached;
mnEmpresa.Imagen = empresa.Imagen;
_context.Update(mnEmpresa);
await _context.SaveChangesAsync();
}
catch (DbUpdateConcurrencyException)
{
if (!MnEmpresaExists(mnEmpresa.IdEmpresa))
{
return NotFound();
}
else
{
throw;
}
}
return RedirectToAction(nameof(Index));
}
return View(mnEmpresa);
}
這是我的模型:
public MnEmpresa()
{
MnAplicactivos = new HashSet<MnAplicactivo>();
MnUsuarios = new HashSet<MnUsuario>();
}
public int IdEmpresa { get; set; }
public int? CodEmpresa { get; set; }
public string Empresa { get; set; }
public string Contacto { get; set; }
public string Imagen { get; set; }
public virtual ICollection<MnAplicactivo> MnAplicactivos { get; set; }
public virtual ICollection<MnUsuario> MnUsuarios { get; set; }
要上傳文件,請在form
標簽中使用enctype="multipart/form-data"
屬性。 僅當 method="post" 時才能使用enctype
屬性。
<form asp-action="Edit" method="post" enctype="multipart/form-data">
....
<input type="file" name="postedFile" id="postedFile" multiple />
<div class="form-group d-flex justify-content-end">
<input type="submit" value="Actualizar" class="btn btn-primary bg-primary" />
</div>
<form>
在控制器中:
public class YourController : Controller
{
private readonly ILogger<HomeController> _logger;
private readonly IWebHostEnvironment _web;
public YourController(IWebHostEnvironment env, ILogger<HomeController> logger)
{
_web = env;
_logger = logger;
}
....
[HttpPost]
public async Task<IActionResult> Edit(int id, [Bind("IdEmpresa,CodEmpresa,Empresa,Contacto")] MnEmpresa mnEmpresa, IFormFile postedFile)
{
if (id != mnEmpresa.IdEmpresa)
{
return NotFound();
}
if (ModelState.IsValid)
{
string wwwPath = _web.WebRootPath;
string contentPath = _web.ContentRootPath;
string path = Path.Combine(_web.WebRootPath, "Uploads");
if (!Directory.Exists(path))
{
Directory.CreateDirectory(path);
}
if (postedFile != null)
{
var target = Path.Combine(path, fileName);
string fileName = Path.GetFileName(postedFile.FileName);
using (FileStream stream = new FileStream(target, fileName), FileMode.Create))
{
postedFile.CopyTo(stream);
}
#region Your previous code
try
{
MnEmpresa empresa = _context.MnEmpresas.Where(x => x.IdEmpresa == mnEmpresa.IdEmpresa).FirstOrDefault();
_context.Entry(empresa).State = EntityState.Detached;
mnEmpresa.Imagen = target;
_context.Update(mnEmpresa);
await _context.SaveChangesAsync();
}
catch (DbUpdateConcurrencyException)
{
if (!MnEmpresaExists(mnEmpresa.IdEmpresa))
{
return NotFound();
}
else
{
throw;
}
}
return RedirectToAction(nameof(Index));
#endregion
}
return View();
}
return View(mnEmpresa);
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.