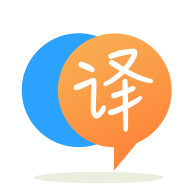
[英]How to store values in a list or array and bind all to datatable then gridview
[英]How can I get the distinct values in a Gridview column and store them into a Datatable or list?
我在名為 Gridview1 的 C# ASPX 頁面中有一個 Gridview,它從 SQL 服務器中提取一個表並將其顯示在 PageLoad 上。
Gridview 中有 10 列,我需要第 7 列中的所有不同值,即代碼
代碼
A
C
D
A
A
D
B
E
R
A
A
C
B
基本上我需要某種結構、列表甚至數據表來從“代碼”列中獲取所有不同的值。 在上面的示例中,它將是一個包含 6 個條目的列表或數據表,因為 Gridview 列中有 6 個唯一代碼。
不同 AB C DE R
任何想法如何實現?
好吧,總是但總是嘗試對數據源而不是 web 頁面 HTML 執行此類代碼(無論是列表視圖,還是網格視圖,中繼器或其他)。 web 頁面用於顯示數據,而不是數據庫內容。
現在,我想在這種情況下,我們可以對網格進行操作,但對數據進行操作通常要容易得多。
所以,說這個網格:
<div style="float:left;width:40%">
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="false"
CssClass="table table-hover table-striped"
DataKeyNames="ID">
<Columns>
<asp:BoundField DataField="FirstName" HeaderText="FirstName" />
<asp:BoundField DataField="LastName" HeaderText="LastName" />
<asp:BoundField DataField="City" HeaderText="City" />
<asp:BoundField DataField="HotelName" HeaderText="HotelName" />
<asp:BoundField DataField="Description" HeaderText="Description" />
<asp:TemplateField ItemStyle-HorizontalAlign="Center">
<ItemTemplate>
<asp:Button ID="cmdDel" runat="server"
Text="Delete"
CssClass="btn"
onclick="cmdDel_Click"
onclientClick="return confirm('really delete this?');"/>
</ItemTemplate>
</asp:TemplateField>
</Columns>
</asp:GridView>
</div>
<div style="float:left;margin-left:40px">
<h4>City list</h4>
<asp:ListBox ID="ListBox1" runat="server"
DataTextField="City"
DataValueField="City" Width="163px" Height="159px"
></asp:ListBox>
</div>
</div>
所以,在網格旁邊,我有一個列表框,我們將列出每個單獨的城市。
所以,要加載的代碼是這樣的:
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
LoadGrid();
}
void LoadGrid()
{
DataTable rstData = MyRst("SELECT * FROM tblHotelsA ORDER BY HotelName");
GridView1.DataSource = rstData;
GridView1.DataBind();
// now fill out a list box of each city
DataTable rstCity = new DataTable();
rstCity = rstData.DefaultView.ToTable(true, "City");
rstCity.DefaultView.Sort = "City";
ListBox1.DataSource = rstCity;
ListBox1.DataBind();
}
我們得到了這個:
因此,請注意我們是如何訪問數據源的——而不是網格(網格不是數據庫,在大多數情況下,我們不應將網格視為這樣)。
但是,我們也可以將值拉出網格(不明白我們為什么要這樣做)。
因此,讓我們放入列表框 2,並添加對網格進行操作的代碼:
我們的第二個列表框 - 這次不是數據綁定。
所以這:
<div style="float:left;margin-left:40px">
<h4>City list 2nd example</h4>
<asp:ListBox ID="ListBox2" runat="server"
DataTextField="Text"
DataValueField="Value"
Width="163px" Height="159px"
></asp:ListBox>
</div>
並說單擊按鈕或其他任何內容-我們運行此代碼以從數據網格中填寫第二個列表框。
僅供參考:如果您使用模板化字段,那么您必須使用 findcontrol 來獲取控件。 如果您使用數據字段,則使用 .cells[] 數組/集合。
但是,請注意,因為空單元格將呈現為非中斷空間 &nsb;
所以,我們從 html 轉換,只是為了保存,我們有這個代碼:
protected void Button1_Click1(object sender, EventArgs e)
{
List<string> CityList = new List<string>();
foreach (GridViewRow gRow in GridView1.Rows)
{
string sCity = Server.HtmlDecode(gRow.Cells[2].Text);
if (!CityList.Contains(sCity))
CityList.Add(sCity);
}
// display our list in 2nd listbox
CityList.Sort();
foreach (string sCity in CityList)
{
ListBox2.Items.Add(new ListItem(sCity, sCity));
}
}
所以,現在我們有了這個:
所以嘗試對數據進行操作。 我的意思是,我們甚至可以說通過查詢獲取不同城市的列表,這樣說:
string strSQL = "SELECT City from tblHotels GROUP BY City";
Datatable rstCity = MyRst(strSQL);
ListBox1.DataSource = rstCity;
ListBox1.DataBind();
所以,大多數情況下??
從數據源中獲取這些數據的工作量要少得多,效果更好,而不是 go 到 GV - 因為它是一個顯示和渲染系統 - 不是數據庫,也不是數據源。
現在,在上面,我使用了一個助手 function MyRst。 所做的只是返回一個基於 sql 的表 - (變得非常累非常快不得不一遍又一遍地鍵入那種類型的代碼)。 所以就是這樣:
DataTable MyRst(string strSQL)
{
DataTable rstData = new DataTable();
using (SqlConnection conn = new SqlConnection(Properties.Settings.Default.TEST4))
{
using (SqlCommand cmdSQL = new SqlCommand(strSQL, conn))
{
conn.Open();
rstData.Load(cmdSQL.ExecuteReader());
}
}
return rstData;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.