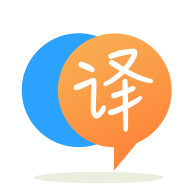
[英]How to add one-to-many relationship data to the database in ASP.NET Core?
[英]ASP.Net Core: How do I update (change/add/remove) nested item objects (One-to-Many relationship)?
我有一個帶有“MSCustomers”和“MSLocations”的.Net 5.x 項目。 MSCustomers 有一個多對一的 MSLocations。
我的“編輯”頁面正確顯示了“MSCustomer”記錄和相應的“MSLocations”字段。
問題:
“編輯”應該允許我修改或“刪除”任何 MSLocation。 但是當我保存記錄時,沒有任何 MSLocations 被更改。
MSCustomer.cs:
public class MSCustomer
{
public int ID { get; set; }
public string CustomerName { get; set; }
public string EngagementType { get; set; }
public string MSProjectNumber { get; set; }
// EF Navigation
public virtual ICollection<MSLocation> MSLocations { get; set; }
}
MSLocation.cs
public class MSLocation
{
public int ID { get; set; }
public string Address { get; set; }
public string City { get; set; }
public string State { get; set; }
public string Zip { get; set; }
public int MSCustomerId { get; set; } // FK
// EF Navigation
public MSCustomer MSCustomer { get; set; }
}
編輯.cshtml.cs:
public class EditModel : PageModel
{
[BindProperty]
public MSCustomer MSCustomer { get; set; }
...
public IActionResult OnGet(int? id)
{
if (id == null)
return NotFound();
MSCustomer = ctx.MSCustomer
.Include(location => location.MSLocations)
.FirstOrDefault(f => f.ID == id);
return Page(); // This all works...
}
public async Task<IActionResult> OnPostAsync(string? submitButton)
{
ctx.Attach(MSCustomer).State = EntityState.Modified;
await ctx.SaveChangesAsync();
return RedirectToPage("Index"); // Saves MSCustomer updates, but not MSLocations...
編輯.cshtml.cs
@page
@model HelloNestedFields.Pages.MSFRD.EditModel
@using HelloNestedFields.Models
...
<form method="POST">
<input type="hidden" asp-for="MSCustomer.ID" />
...
<table>
<thead>
<tr>
<th style="min-width:140px">Address</th>
<th style="min-width:140px">City</th>
<th style="min-width:140px">State</th>
<th style="min-width:140px">Zip</th>
</tr>
</thead>
<tbody>
@foreach (MSLocation loc in @Model.MSCustomer.MSLocations)
{
<tr id="row_@loc.ID">
<td><input asp-for="@loc.Address" /></td>
<td><input asp-for="@loc.City" /></td>
<td><input asp-for="@loc.State" /></td>
<td><input asp-for="@loc.Zip" /></td>
<td><button onclick="removeField(@loc.ID);">Remove</button></td>
</tr>
}
<tr>
<td></td>
<td></td>
<td></td>
<td></td>
<td><button id="add_location_btn">Add Location</button></td>
</tr>
</tbody>
</table>
...
@section Scripts {
<script type="text/javascript">
function removeField(element_id) {
try {
let row_id = "row_" + element_id;
console.log("removeField, element_id=" + element_id + ", row_id=" + row_id + "...");
let tr = document.getElementById(row_id);
console.log("tr:", tr);
tr.parentNode.removeChild(tr);
} catch (e) {
console.error(e);
}
debugger;
};
</script>
}
HelloNestedContext.cs
public class HelloNestedContext : DbContext
{
public HelloNestedContext(DbContextOptions<HelloNestedContext> options)
: base(options)
{
}
public DbSet<HelloNestedFields.Models.MSCustomer> MSCustomers { get; set; }
public DbSet<HelloNestedFields.Models.MSLocation> MSLocations { get; set; }
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.Entity<MSCustomer>()
.HasMany(d => d.MSLocations)
.WithOne(c => c.MSCustomer)
.HasForeignKey(d => d.MSCustomerId);
}
}
問:我錯過了什么?
問:我需要做什么才能將MSCustomers.MSLocations更新從瀏覽器傳遞回 OnPostAsync() 並正確保存?
我確定這是可能的。 但是我無法在任何地方找到任何文檔或示例代碼來修改“記錄對象”中的“嵌套項目對象”。
任何建議都將非常受歡迎!
更新:
Razor 頁面似乎不支持綁定到“復雜” object(在記錄中具有嵌套列表)。
所以我在下面嘗試了 Okan Karadag 的極好建議 - 我將“MSLocations”拆分為自己的綁定,然后將其添加回“POST”處理程序中的“MSCustomer”。 這讓我更接近- 至少現在我現在能夠更新嵌套字段。 但我仍然無法在“編輯”頁面中添加或刪除 MSLocations。
新的 Edit.cshtml.cs
[BindProperty]
public MSCustomer MSCustomer { get; set; }
[BindProperty]
public List<MSLocation> MSLocations { get; set; }
...
public IActionResult OnGet(int? id)
{
MSCustomer = ctx.MSCustomer
.Include(location => location.MSLocations)
.FirstOrDefault(f => f.ID == id);
MSLocations = new List<MSLocation>(MSCustomer.MSLocations);
...
public async Task<IActionResult> OnPostAsync(string? submitButton)
{
MSCustomer.MSLocations = new List<MSLocation>(MSLocations); // Update record with new
ctx.Update(MSCustomer);
await ctx.SaveChangesAsync();
...
新的 Edit.cshtml
<div class="row">
...
<table>
...
<tbody id="mslocations_tbody">
@for (int i=0; i < Model.MSLocations.Count(); i++)
{
<tr id="row_@Model.MSLocations[i].ID">
<td><input asp-for="@Model.MSLocations[i].Address" /></td>
<td><input asp-for="@Model.MSLocations[i].City" /></td>
<td><input asp-for="@Model.MSLocations[i].State" /></td>
<td><input asp-for="@Model.MSLocations[i].Zip" /></td>
<td>
<input type="hidden" asp-for="@Model.MSLocations[i].ID" />
<input type="hidden" asp-for="@Model.MSLocations[i].MSCustomerId" />
<button onclick="removeLocation(row_@Model.MSLocations[i].ID);">Remove</button>
</td>
</tr>
}
</tbody>
</table>
<button onclick="addLocation();">Add Location</button>
</div>
當前狀態:
我的下一步將是嘗試這個:
如何將來自不同實體的項目動態添加到 ASP.NET Core MVC 中的列表
如果它有效,那就太好了。 如果有一個不涉及Ajax調用的更簡單的替代方案,那就更好了。
發送數據時,應該是customer.Locations[0].City: "foo"
customer.Locations[1].City: "bar"
,你應該發布為 Locations[index]。 您可以在瀏覽器的網絡選項卡中查看傳遞的數據。
解決方案 1(使用for
)
@for (var i = 0; i < Model.MSCustomer.Locations.Count(); i++)
{
<tr id="row_@loc.ID">
<td><input asp-for="@Model.MSCustomer.Locations[i].Address" /></td>
<td><input asp-for="@Model.MSCustomer.Locations[i].City" /></td>
<td><input asp-for="@Model.MSCustomer.Locations[i].State" /></td>
<td><input asp-for="@Model.MSCustomer.Locations[i].Zip" /></td>
<td><button onclick="removeField(@loc.ID);">Remove</button></td>
</tr>
}
解決方案 2(使用foreach
)
@foreach (MSLocation loc in @Model.MSCustomer.MSLocations)
{
<tr id="row_@loc.ID">
<td><input asp-for="@Model.MSCustomer.MSLocations[loc.Id].Address" /></td>
<td><input asp-for="@Model.MSCustomer.MSLocations[loc.Id].City" /></td>
<td><input asp-for="@Model.MSCustomer.MSLocations[loc.Id].State" /></td>
<td><input asp-for="@Model.MSCustomer.MSLocations[loc.Id].Zip" /></td>
<td><button onclick="removeField(@loc.ID);">Remove</button></td>
</tr>
}
好的:我的基本挑戰是弄清楚如何在 ASP.Net Core MVC 中創建一個簡單的 web 表單,其中的“主記錄”具有“嵌套字段”。
一個“剃刀頁面”,我可以在其中創建和編輯這樣的架構:
* Customers
int ID
string Name
List<Location> Locations
* Locations:
int ID
string Address
string City
string State
string Zip
int CustomerID
我開始創建我的模型:
模型\客戶.cs
public class Customer { public int ID { get; set; } public string CustomerName { get; set; } public string EngagementType { get; set; } public string MSProjectNumber { get; set; } // EF Navigation public virtual ICollection<MSLocation> MSLocations { get; set; }
模型\MSLocation.cs
public class MSLocation { public int ID { get; set; } public string Address { get; set; } public string City { get; set; } public string State { get; set; } public string Zip { get; set; } public int CustomerId { get; set; } // FK // EF Navigation public Customer Customer { get; set; } <= Both Customer, MSLocation configured for EF navigation
接下來,我將 DBContext 配置為支持導航:
數據\HelloNestedContext.cs
public class HelloNestedContext: DbContext {... public DbSet<HelloNestedFields.Models.Customer> Customers { get; set; } public DbSet<HelloNestedFields.Models.MSLocation> MSLocations { get; set; } protected override void OnModelCreating(ModelBuilder modelBuilder) { base.OnModelCreating(modelBuilder); ... modelBuilder.Entity<Customer>().HasMany(d => d.MSLocations).WithOne(c => c.Customer).HasForeignKey(d => d.CustomerId); <= Configure "One::Many " relationship in DbContext
一次性使用查詢主記錄和嵌套字段既簡單又高效:
Pages\MSFRD\Edit.cshtml.cs > OnGet()
Customer = ctx.Customers.Include(location => location.MSLocations).FirstOrDefault(f => f.ID == id); <= So far, so good: this all worked fine in the original "Departments/Courses/Course Auditors" example...
挑戰:
問題 #1:Razor 頁面不支持綁定到嵌套子字段(例如“Customer.MSLocations”)
解決方案:將子字段聲明為自己的 model 變量(例如“List MSLocations”),並與“主記錄”分開綁定
問題 #2:在提交更新時合並更新以將“MSLocations”分離回“Customer.MSLocations”
解決方案:
Pages\MSFRD\Edit.cshtml.cs > OnPostAsync ()
... Customer.MSLocations = new List<MSLocation>(MSLocations); ctx.Update(Customer); await ctx.SaveChangesAsync(); <= No problem: Fairly straightforward...
問題 #3a:向“Customer.MSLocations”添加新子字段
SOLUTION:使用JS創建新的HTML元素; 遵循與 Razor 使用相同的 id/attribute 元素屬性命名約定
例子:
<td><input type="text" id="MSLocations_3__Address" name="MSLocations[3].Address" /> <td><input type="text" id="MSLocations_3__City" name="MSLocations[3].City" />...
頁面\MSFRD\Edit.cshtml
function addLocation() { console.log("addLocation(), maxRow=" + maxRow + "..."); //debugger; try { let markup = `<tr id="row_${maxRow}"> <td><input type="text" id="MSLocations_${maxRow}__Address" name="MSLocations[${maxRow}].Address" /></td> <td><input type="text" id="MSLocations_${maxRow}__City" name="MSLocations[${maxRow}].City" /></td> <td><input type="text" id="MSLocations_${maxRow}__State" name="MSLocations[${maxRow}].State" /></td> <td><input type="text" id="MSLocations_${maxRow}__Zip" name="MSLocations[${maxRow}].Zip" /></td> <td> <input type="hidden" id="MSLocations_${maxRow}__ID" name="MSLocations[${maxRow}].ID" value="0" /> <input type="hidden" id="MSLocations_${maxRow}__CustomerId" name="MSLocations[${maxRow}].CustomerId" value="@Model.Customer.ID" /> <button onclick="return removeLocation(row_${maxRow}, ${maxRow});">Remove</button> </td> </tr>`; //console.log("markup:", markup); let tbody = $("#mslocations_tbody"); tbody.append(markup); ++maxRow;
問題#3b:跟蹤當前行#
解決方案: <input type="hidden" id="MaxRow" value="@Model.MaxRow" />
問題 #4:刪除子字段
解決方案:
Pages\MSFRD\Edit.cshtml.cs > OnPostAsync ()
... JArray deletedLocations = JArray.Parse(DeletedLocations); foreach (var jobject in deletedLocations) { MSLocation MSLocation = jobject.ToObject<MSLocation>(); ctx.Remove(MSLocation); }
在“ctx.Remove()”循環之后,使用所有添加/修改更新“Customer”,並調用“ctx.SaveChangesAsync()”:
// Remove any deleted locations from DB JArray deletedLocations = JArray.Parse(DeletedLocations); foreach (var jobject in deletedLocations) { MSLocation MSLocation = jobject.ToObject<MSLocation>(); ctx.Remove(MSLocation); } // Associate MSLocations modifications/updates with Customer record Customer.MSLocations = new List<MSLocation>(MSLocations); ctx.Update(Customer); // Update DB await ctx.SaveChangesAsync();
主要優勢:
一個。 最小化數據庫的#/往返次數
灣。 最小化每次往返的 SQL 級數據量
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.