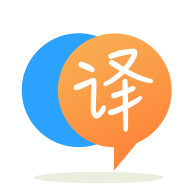
[英]WPF SelectedValue of combobox not updating from viewmodel property
[英]WPF Combobox in view not updating when bound property in viewmodel updated
我在視圖中有一個 Combobox,綁定到一個視圖模型,該視圖模型具有我的自定義 class 的 ObservableCollection。 這個自定義的 class 只是一個包含值的枚舉的包裝器,以及一個作為枚舉描述屬性的字符串。 combobox 將 DisplayMemberPath 屬性設置為此名稱屬性,以顯示更易於閱讀的描述屬性值,而不是枚舉本身
我發現當我將 combobox 的 ItemsSource 設置為這些枚舉包裝類的集合,然后將 SelectedItem 屬性設置為這些項目之一時,當我啟動我的應用程序時,combobox 不會在 UI 中更新。 如果我將其更改為字符串列表,它似乎可以工作。
這是我的 combobox:
<ComboBox
DisplayMemberPath="Name"
IsEditable="False"
IsReadOnly="True"
ItemsSource="{Binding SelectableTags}"
SelectedItem="{Binding SelectedTag, Mode=TwoWay, UpdateSourceTrigger=PropertyChanged}" />
我的自定義 class combobox 綁定到以下集合:
public class EnumNamePair : ObservableObject
{
private string name;
public string Name
{
get => name;
set => SetProperty(ref name, value);
}
private Enum enumValue;
public Enum EnumValue
{
get => enumValue;
set
{
SetProperty(ref enumValue, value);
Name = enumValue.GetEnumDescription();
}
}
public EnumNamePair(Enum enumValue)
{
EnumValue = enumValue;
}
}
我的視圖模型的一部分:
private ObservableCollection<EnumNamePair> selectableTags = new();
public ObservableCollection<EnumNamePair> SelectableTags
{
get => selectableTags;
set => SetProperty(ref selectableTags, value);
}
private EnumNamePair selectedTag;
public EnumNamePair SelectedTag
{
get => selectedTag;
set => SetProperty(ref selectedTag, value);
}
public TaggingRuleViewModel(string tag)
{
SelectableTags = new List<EnumNamePair>(
Enum.GetValues(typeof(AllowedTag)).Cast<AllowedTag>().Select(x => new EnumNamePair(x)));
SelectedTag = SelectableTags.First(x => x.EnumValue.ToString() == tag),
}
這是簡化的,但會重現問題。 我已經為我的綁定屬性嘗試了 OnPropertyChanged 的各種額外提升,更改了 combobox 上的只讀/可編輯屬性設置器,降低了我的視圖模型/自定義 class(它曾經有一個用於 name 屬性的 getter,而不是設置 EnumValue ETC)。 似乎所有的工作是將我的自定義 class 列表更改為字符串列表,並在視圖模型中處理轉換。 我知道還有其他方法可以處理在 combobox 中顯示枚舉描述屬性,但此時我只是想知道為什么這不起作用。
轉換器實現示例:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Core2022.SO.JosephWard
{
public static class EnumExtensions
{
private static readonly Dictionary<Type, Dictionary<string, string>> enums
= new Dictionary<Type, Dictionary<string, string>>();
public static string GetEnumDescription<T>(this T value)
where T : Enum
{
Type enumType = value.GetType();
if (!enums.TryGetValue(enumType, out Dictionary<string, string>? descriptions))
{
descriptions = enumType.GetFields()
.ToDictionary(
info => info.Name,
info => ((DescriptionAttribute?)info.GetCustomAttributes(typeof(DescriptionAttribute), false)?.FirstOrDefault())?.Description ?? info.Name);
enums.Add(enumType, descriptions);
}
string name = value.ToString();
if (descriptions.TryGetValue(name, out string? description))
{
return description;
}
else
{
throw new ArgumentException("UnexpectedValue", nameof(value));
}
}
}
}
using System;
using System.Globalization;
using System.Windows;
using System.Windows.Data;
namespace Core2022.SO.JosephWard
{
[ValueConversion(typeof(Enum), typeof(string))]
public class EnumToDescriptionConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (value is Enum @enum)
return @enum.GetEnumDescription();
return DependencyProperty.UnsetValue;
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
throw new NotImplementedException();
}
public static EnumToDescriptionConverter Instance { get; } = new EnumToDescriptionConverter();
}
}
using System;
using System.Windows.Markup;
namespace Core2022.SO.JosephWard
{
[MarkupExtensionReturnType(typeof(EnumToDescriptionConverter))]
public class EnumToDescriptionExtension : MarkupExtension
{
public override object ProvideValue(IServiceProvider serviceProvider)
{
return EnumToDescriptionConverter.Instance;
}
}
}
using System.ComponentModel;
namespace Core2022.SO.JosephWard
{
public enum AllowedTag
{
[Description("Value not set")]
None,
[Description("First value")]
First,
[Description("Second value")]
Second
}
}
using System.Collections.ObjectModel;
namespace Core2022.SO.JosephWard
{
public class EnumsViewModel
{
public ObservableCollection<AllowedTag> Tags { get; } = new ObservableCollection<AllowedTag>()
{ AllowedTag.None, AllowedTag.Second, AllowedTag.First };
}
}
<Window x:Class="Core2022.SO.JosephWard.EnumsWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:Core2022.SO.JosephWard"
mc:Ignorable="d"
Title="EnumsWindow" Height="150" Width="300">
<Window.DataContext>
<local:EnumsViewModel/>
</Window.DataContext>
<Grid>
<ListBox ItemsSource="{Binding Tags}">
<ItemsControl.ItemTemplate>
<DataTemplate DataType="{x:Type local:AllowedTag}">
<TextBlock Text="{Binding Converter={local:EnumToDescription}}"/>
</DataTemplate>
</ItemsControl.ItemTemplate>
</ListBox>
</Grid>
</Window>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.