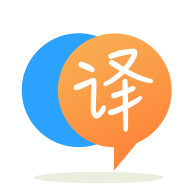
[英]Why an array pointer with index returns the value in that index instead of returning the specific index adress?
[英]Returning the value of a specific array[index] - C
我是 C 編程語言的新手,我再次發現自己陷入了困境。 我需要在生成隨機數的數組中找到最大值和最小值(以及它們各自的索引)。 我能夠在屏幕上顯示這些值,但不能顯示它們的具體索引。 我應該如何繼續讓它工作。 這是我的嘗試:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define SIZE 10
#define MIN 100
#define MAX 990
int main(void) {
int index;
float array[SIZE];
float largestValue, smallestValue;
srand(time(NULL));
for (index = 0; index < SIZE; index++) {
array[index] = (rand() % (MAX - MIN + 1) + MIN) / 10.0;
printf("Index [%i]: %.1f\n", index, array[index]);
}
largestValue = array[0];
smallestValue = array[0];
for (index = 0; index < SIZE; index++){
if (array[index] > largestValue)
largestValue = array[index];
if (array[index] < smallestValue)
smallestValue = array[index];
}
/*
printf("This is the smallest value: [%i] %.1f\n", index, smallestValue);
printf("This is the largest value: [%i] %.1f\n", index, largestValue);
OR
printf("This is the smallest value: [%f] %.1f\n", array[smallestValue], smallestValue);
printf("This is the largest value: [%f] %.1f\n", array[largestValue], largestValue);
*/
return 0;
}
如果您需要知道索引,則只需將它們與您已經找到的最大值和最小值一起保存。
例如:
int index;
float array[SIZE];
float largestValue, smallestValue;
int largestIndex, smallestIndex;
然后在搜索最大值和最小值時:
largestValue = array[0];
largestIndex = 0;
smallestValue = array[0];
smallestIndex = 0;
for (index = 1; index < SIZE; index++){ //You can start at index 1 instead of 0
if (array[index] > largestValue){
largestValue = array[index];
largestIndex = index;
}
if (array[index] < smallestValue){
smallestValue = array[index];
smallestIndex = index;
}
}
您可以聲明兩個整數來保存最后一個 if 語句中的index
值。 像這樣:
int largestValueIndex = 0, smallestValueIndex = 0;
for (index = 0; index < SIZE; index++){
if (array[index] > largestValue){
largestValue = array[index];
largestValueIndex = index;
}
if (array[index] < smallestValue){
smallestValue = array[index];
smallestValueIndex = index;
}
}
希望這對您有所幫助,我希望對您來說是最好的,作為一個初學者
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.