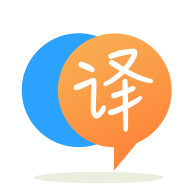
[英]Can someone give me an example of “pure pointer notation” to an array in C?
[英]Pure Pointer notation in C
我有這個編碼分配,我只能使用純指針表示法。 我幾乎完成了它,但我剛剛意識到我使用了一個數組。 我不允許這樣做,除非我以某種方式將其更改為指針。 這就是我有點卡住的地方。
這是我的代碼。
#include <stdio.h>
#include <stdlib.h>
/* Function Prototypes */
int main();
void s1(char *random);
void s2(char *s2_input, int index);
void strfilter(char *random, char *s2_input, char replacement);
int main()
{
for(;;)
{
int s1_index = 41;
char s1_random[s1_index];
s1(s1_random);
printf("\ns1 = ");
puts(s1_random);
printf("s2 = ");
int s2_index = 21;
char s2_input[s2_index];
s2(s2_input, s2_index);
if(s2_input[1] == '\0')
{
printf("Size too small");
exit(0);
}
if(s2_input[21] != '\0' )
{
printf("Size too big");
exit(0);
}
printf("ch = ");
int replacement = getchar();
if(replacement == EOF)
break;
while(getchar() != '\n');
printf("\n");
strfilter(s1_random, s2_input, replacement);
printf("\ns1 filtered = ");
puts(s1_random);
printf("Do you wish to run again? Yes(Y), No(N) ");
int run = getchar();
// or include ctype.h and do:
// run == EOF || toupper(run) == 'N'
if(run == EOF || run == 'N' || run == 'n')
break;
while(getchar() != '\n');
}
}
void s1(char *random)
{
int limit = 0;
char characters;
while((characters = (('A' + (rand() % 26))))) /* random generator */
{
if(limit == 41)
{
*(random + 41 - 1) = '\0';
break;
}
*(random + limit) = characters;
limit++;
}
}
void s2(char *s2_input, int index)
{
char array[21] = "123456789012345678901"; /* populated array to make sure no random memory is made */
char input;
int count = 0;
int check = 0;
while((input = getchar() ))
{
if(input == '\n')
{
*(s2_input + count) = '\0';
break;
}
else if(input < 65 || input > 90)
{
printf("invalid input");
exit(0);
}
*(s2_input + count) = input;
count++;
}
index = count;
}
void strfilter(char *random, char *s2_input, char replacement) /* replacement function */
{
while(*s2_input)
{
char *temp = random;
while(*temp)
{
if(*temp == *s2_input)
*temp = replacement;
temp++;
}
s2_input++;
}
}
我的問題是這部分我不確定如何編輯它以不包含數組,並且仍然以相同的方式擁有它 output 程序。
if(s2_input[1] == '\0')
{
printf("Size too small");
exit(0);
}
if(s2_input[21] != '\0' )
{
printf("Size too big");
exit(0);
}
我試圖在某個點獲取數組的地址,然后用指針取消引用它,但它仍在使用數組。 這是我要避免的。 任何幫助將不勝感激!
s2_input[i]
可以寫成*(s2_input+i)
其中i
是某個索引。
if ((s2_input[1]) == '\0')
相當於:
if (*(s2 + 1) == '\0')
這意味着取消引用 s2 處的值(這是第 0 個 [0] 元素的位置),並向其加一。 可以對任何其他位置進行相同的操作。
指針表示法和通常稱為索引表示法(使用[ ]
下標運算符)是完全等價的。 任何一個概念都提供指針地址加上該指針地址的偏移量。 參見C11 標准 - 6.5.2.1 數組下標即array[offset]
或*(array + offset)
1
例如,使用*array
訪問第一個元素是*(array + 0)
的簡寫,它只是索引符號中的array[0]
。 0
是原始指針地址的偏移量(在該類型的元素中)。 (類型控制指針算法)
所以array[10]
就是*(array + 10)
。 如果 array 是char
類型,則array[10]
是array
地址后的 10 個字節。 如果數組是int
類型(其中int
是 4 字節),則array[10]
是array
地址 (10-int) 之后的 40 字節。
對於二維數組, arr2d[1][2]
的表示法只是*(arr2d[1] + 2)
,進一步擴展就是*(*(arr2d + 1) + 2)
。
所以通常array[i]
是*(array + i)
和arr2d[i][j]
是*(*(arr2d + i) + j)
。
腳注:
array[offset]
等同於*(array + offset)
等同於offset[array]
。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.