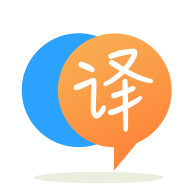
[英]C# Double - ToString() formatting with two decimal places but no rounding
[英]Rounding multiple double numbers to two decimal places
我是編程新手,我決定嘗試編寫這個程序,作為練習,最后它運行良好,沒有問題。
在第三條評論下方,我正在初始化一個執行計算的變量,在該語句之后,我將值四舍五入到小數點后兩位。 看着那段代碼塊,我將這兩行重復了 7 次,我想可能有更好的方法來縮短那段代碼。
double weightEarth, earthG, mercuryG, marsG, uranusG, venusG, saturnG, neptuneG, jupiterG;
//Assigning gravity force value corresponding to each planet
earthG = 9.81;
mercuryG = 3.7;
marsG = 3.711;
uranusG = 8.69;
venusG = 8.87;
saturnG = 10.44;
neptuneG = 11.15;
jupiterG = 24.79;
//Receive user input
Console.ForegroundColor = ConsoleColor.Green;
Console.WriteLine("This program will calculate how much you will weight on all planets");
Console.Write("Type in your weight in kg: ");
weightEarth = double.Parse(Console.ReadLine());
//Calculating mass of object by dividing and gravitional force by multiplication
double weightMercury = (weightEarth / earthG) * mercuryG;
weightMercury = Math.Round(weightMercury, 2);
double weightMars = (weightEarth / earthG) * marsG;
weightMars = Math.Round(weightMars, 2);
double weightUranus = (weightEarth / earthG) * uranusG;
weightUranus = Math.Round(weightUranus, 2);
double weightVenus = (weightEarth / earthG) * venusG;
weightVenus = Math.Round(weightVenus, 2);
double weightSaturn = (weightEarth / earthG) * saturnG;
weightSaturn = Math.Round(weightSaturn, 2);
double weightNeptune = (weightEarth / earthG) * neptuneG;
weightNeptune = Math.Round(weightNeptune, 2);
double weightJupiter = (weightEarth / earthG) * jupiterG;
weightJupiter = Math.Round(weightJupiter, 2);
Console.ForegroundColor = ConsoleColor.Blue;
Console.WriteLine("\r\nYou weight:");
Console.WriteLine(weightMercury + "kg on Mercury");
Console.WriteLine(weightMars + "kg on Mars");
Console.WriteLine(weightUranus + "kg on Uranus");
Console.WriteLine(weightVenus + "kg on Venus");
Console.WriteLine(weightSaturn + "kg on Saturn");
Console.WriteLine(weightNeptune + "kg on Neptune");
Console.WriteLine(weightJupiter + "kg on Jupiter");
Console.ReadKey();
根據您想達到 go 的距離,您可以遵循一些概念。
第一個是不要重復自己 (DRY)。 您可以將權重計算提取到一種新方法中,例如,
private static double GetWeightForPlanet(double weightEarth, double earthG, double planetG)
{
double weightPanet = (weightEarth / earthG) * planetG;
weightPanet = Math.Round(weightPanet, 2);
return weightPanet;
}
現在你的程序看起來像這樣,
//Calculating mass of object by dividing and gravitional force by multiplication
double weightMercury = GetWeightForPlanet(weightEarth, earthG, mercuryG);
double weightMars = GetWeightForPlanet(weightEarth, earthG, marsG);
double weightUranus = GetWeightForPlanet(weightEarth, earthG, uranusG);
double weightVenus = GetWeightForPlanet(weightEarth, earthG, venusG);
double weightSaturn = GetWeightForPlanet(weightEarth, earthG, saturnG);
double weightNeptune = GetWeightForPlanet(weightEarth, earthG, neptuneG);
double weightJupiter = GetWeightForPlanet(weightEarth, earthG, jupiterG);
Console.ForegroundColor = ConsoleColor.Blue;
Console.WriteLine("\r\nYou weight:");
Console.WriteLine(weightMercury + "kg on Mercury");
Console.WriteLine(weightMars + "kg on Mars");
Console.WriteLine(weightUranus + "kg on Uranus");
Console.WriteLine(weightVenus + "kg on Venus");
Console.WriteLine(weightSaturn + "kg on Saturn");
Console.WriteLine(weightNeptune + "kg on Neptune");
Console.WriteLine(weightJupiter + "kg on Jupiter");
仍然有一些重復代碼,但我可以看到一些常見的數據模式。 也許數據結構會有所幫助; 讓我們介紹一下概念(類) Pl.net
,
public class Planet
{
public string Name { get; set; }
public double Gforce { get; set; }
}
您的代碼將如下所示,
double earthG = 9.81, weightEarth;
var planets = new List<Planet>
{
new Planet { Name = "Mercury", Gforce = 3.7 },
new Planet { Name = "Mars", Gforce = 3.711},
new Planet { Name = "Uranus", Gforce = 8.69 },
new Planet { Name = "Saturn", Gforce = 10.44 },
new Planet { Name = "Neptune", Gforce = 11.15 },
new Planet { Name = "Venus", Gforce = 8.87 },
new Planet { Name = "Jupiter", Gforce = 24.79 }
};
//Receive user input
Console.ForegroundColor = ConsoleColor.Green;
Console.WriteLine("This program will calculate how much you will weight on all planets");
Console.Write("Type in your weight in kg: ");
weightEarth = double.Parse(Console.ReadLine());
Console.ForegroundColor = ConsoleColor.Blue;
Console.WriteLine("\r\nYou weight:");
foreach (var planet in planets)
{
Console.WriteLine($"{GetWeightForPlanet(weightEarth, earthG, planet.Gforce)} kg on {planet.Name}");
}
我可以繼續前進,但可能會停在這里。 這僅用於演示目的,不適用於生產代碼。 善待代碼審查:)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.