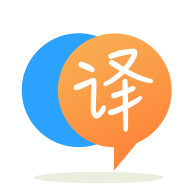
[英]How do I access wity python ctypes to array values returned with a pointer from a dll coded in Delphi?
[英]How do i read the values of a returned pointer from ctypes?
我目前正在與 ctypes 作斗爭。 我能夠將 python 列表轉換為浮點數組並將其提供給 C 函數。 但我不知道如何將這個數組從 C 函數返回到 python 列表......
Python代碼
class Point(ctypes.Structure):
_fields_= [("a", ctypes.c_float * 4),
("aa", ctypes.c_int)]
floats = [1.0, 2.0, 3.0, 4.0]
FloatArray4 = (ctypes.c_float * 4)
parameter_array = FloatArray4(*floats)
test1 = clibrary.dosth
test1.argtypes = [ctypes.c_float * 4, ctypes.c_int]
test1.restype = ctypes.POINTER(Point)
struc = test1(parameter_array, 9)
p = (struc.contents.a)
print(p)
clibrary.free_memory(struc)
C 函數基本上將 parameter_array 放入結構 ant 返回結構.. C 代碼:
#include <stdio.h>
#include <stdlib.h>
struct a{float *a;
int aa;
} ;
struct a *dosth(float *lsit, int x){
struct a *b = malloc(200000);
b -> a = lsit;
b -> aa = 3;
return b;
}
void free_memory(struct a *pointer){
free(pointer);
}
Python 中 print(p) 的 Output 是:
<__main__.c_float_Array_4 object at 0x000001FE9EEA79C0>
我如何獲得這些值?
切片ctypes
指針將生成 Python 內容列表。 由於指針不知道它指向多少項,因此您需要知道大小,通常是通過另一個參數:
>>> import ctypes as ct
>>> f = (ct.c_float * 4)(1,2,3,4)
>>> f
<__main__.c_float_Array_4 object at 0x00000216D6B7A840>
>>> f[:4]
[1.0, 2.0, 3.0, 4.0]
這是一個基於您的代碼的充實示例:
測試.c
#include <stdlib.h>
#ifdef _WIN32
# define API __declspec(dllexport)
#else
# define API
#endif
struct Floats {
float *fptr;
size_t size;
};
API struct Floats *alloc_floats(float *fptr, size_t size) {
struct Floats *pFloats = malloc(sizeof(struct Floats));
pFloats->fptr = fptr;
pFloats->size = size;
return pFloats;
}
API void free_floats(struct Floats *pFloats) {
free(pFloats);
}
測試.py
import ctypes as ct
class Floats(ct.Structure):
_fields_= (('fptr', ct.POINTER(ct.c_float)), # Pointer, not array.
('size', ct.c_int)) # Used to know the size of the array pointed to.
# Display routine when printing this class.
# Note the slicing of the pointer to generate a Python list.
def __repr__(self):
return f'Floats({self.fptr[:self.size]})'
dll = ct.CDLL('./test')
dll.alloc_floats.argtypes = ct.POINTER(ct.c_float), ct.c_size_t
dll.alloc_floats.restype = ct.POINTER(Floats)
dll.free_floats.argtypes = ct.POINTER(Floats),
dll.free_floats.restype = None
data = (ct.c_float * 4)(1.0, 2.0, 3.0, 4.0)
p = dll.alloc_floats(data, len(data))
print(p.contents)
dll.free_floats(p)
Output:
Floats([1.0, 2.0, 3.0, 4.0])
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.