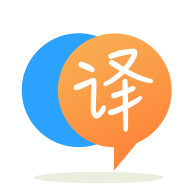
[英]Button Click not firing When focus is set to next cell in DataGridView on CellEndEdit
[英]current cell focus problem after using cellendedit event
我正在設計一個 c# 數據網格視圖,其中回車鍵用作制表符,后退鍵導致返回到上一個單元格。 此外,如果焦點位於最后一列最后一行的最后一個單元格,它會創建一個新行。
private void dataGridView1_KeyDown(object sender, KeyEventArgs e)
{
e.SuppressKeyPress = true;
int iColumn = dataGridView1.CurrentCell.ColumnIndex;
int iRow = dataGridView1.CurrentCell.RowIndex;
if (e.KeyCode == Keys.Enter || e.KeyCode == Keys.Right || e.KeyCode == Keys.Tab)
{
//to check if the pointer reach last column
if (iColumn == dataGridView1.ColumnCount - 1)
{
//executing when line ends
if (dataGridView1.RowCount > (iRow + 1))
{
dataGridView1.CurrentCell = dataGridView1[0, iRow + 1];
}
// fires when you reach last cell of last row and last column
else
{
dataGridView1.Rows.Add();
dataGridView1.CurrentCell = dataGridView1[0, iRow +1];
}
}
else
{
dataGridView1.CurrentCell = dataGridView1[iColumn + 1, iRow];
}
}
if (e.KeyCode == Keys.Down)
{
int c = dataGridView1.CurrentCell.ColumnIndex;
int r = dataGridView1.CurrentCell.RowIndex;
if (r < dataGridView1.Rows.Count - 1) //check for index out of range
dataGridView1.CurrentCell = dataGridView1[c, r + 1];
}
if (e.KeyCode == Keys.Up)
{
int c = dataGridView1.CurrentCell.ColumnIndex;
int r = dataGridView1.CurrentCell.RowIndex;
if (r > 0) //check for index out of range
dataGridView1.CurrentCell = dataGridView1[c, r - 1];
}
if (e.KeyCode == Keys.Back || e.KeyCode == Keys.Left)
{
//to check if you are in first cell of any line
if (iColumn == 0)
{
//to check if you are in first cell of fist line
if (iRow == 0)
{
MessageBox.Show("you reached first cell");
}
else
{
dataGridView1.CurrentCell = dataGridView1[dataGridView1.ColumnCount - 1, iRow - 1];
}
}
else
{
dataGridView1.CurrentCell = dataGridView1[iColumn - 1, iRow];
}
}
}
Now , after this i want to edit the cell values and want to move to next cell when i press enter key , so i add the following event (as i am in cell editing mode due to this, the above key down event will not raise )
private void dataGridView1_CellEndEdit(object sender, DataGridViewCellEventArgs e)
{
// MOVE TO NEXT CELL WHEN DONE EDITING
SendKeys.Send("{right}");
}
但是在使用上面的事件 cellendedit 之后,我發現單元格正在向下移動到下一行(datagridview 的默認行為),然后移動到下一行的下一個單元格(由於 cellendedit)。 相反,它應該移動到同一行的下一列。 請建議我修復此行為。
您的問題是如何取消默認的DataGridView
導航並替換您自己的導航。 默認導航發生在OnKeyDown
方法中,如果您在自定義控件中覆蓋它(讓我們稱之為DataGridViewEx
),您應該能夠通過覆蓋該方法來抑制默認導航,確保您不調用基本 class 版本Keys.Enter
和Keys.Back
。
BindingList<Record> Records = new BindingList<Record>();
protected override void OnKeyDown(KeyEventArgs e)
{
if (CurrentCell != null)
{
var row = CurrentCell.RowIndex;
var col = CurrentCell.ColumnIndex;
switch (e.KeyData)
{
case Keys.Return:
col++;
// [ToDo] Allow for non-visible rows (will fail).
if(col.Equals(Columns.Count))
{
col = 0;
row++;
// Either insert after the current row as
// shown here, or add to the end of the list.
Records.Insert(row, new Record());
}
CurrentCell = this[col, row];
break;
case Keys.Back:
col--;
if(col >= 0)
{
CurrentCell = this[col, row];
}
break;
default:
base.OnKeyDown(e);
break;
}
}
}
試驗台
以下是我在更改 MainForm.Designer.cs 中的兩個條目以使用DataGridViewEx
后測試此解決方案的方式:
namespace current_cell_focus
{
public partial class MainForm : Form
{
public MainForm() => InitializeComponent();
}
// Custom control
class DataGridViewEx : DataGridView
{
protected override void OnHandleCreated(EventArgs e)
{
base.OnHandleCreated(e);
AllowUserToAddRows = false;
DataSource = Records;
// Format columns
Records.Add(new Record());
foreach (DataGridViewColumn col in Columns)
{
col.AutoSizeMode = DataGridViewAutoSizeColumnMode.Fill;
}
Records.Clear();
// Load test data
Records.Add(new Record
{
Column1 = "1",
Column2 = "2",
Column3 = "3"
});
Records.Add(new Record
{
Column1 = "4",
Column2 = "5",
Column3 = "6"
});
Records.Add(new Record
{
Column1 = "7",
Column2 = "8",
Column3 = "9"
});
}
BindingList<Record> Records = new BindingList<Record>();
protected override void OnKeyDown(KeyEventArgs e)
{
if (CurrentCell != null)
{
var row = CurrentCell.RowIndex;
var col = CurrentCell.ColumnIndex;
switch (e.KeyData)
{
case Keys.Return:
col++;
// [ToDo] Allow for non-visible rows (will fail).
if(col.Equals(Columns.Count))
{
col = 0;
row++;
// Either insert after the current row as
// shown here, or add to the end of the list.
Records.Insert(row, new Record());
}
CurrentCell = this[col, row];
break;
case Keys.Back:
col--;
if(col >= 0)
{
CurrentCell = this[col, row];
}
break;
default:
base.OnKeyDown(e);
break;
}
}
}
}
class Record
{
public string Column1 { get; set; }
public string Column2 { get; set; }
public string Column3 { get; set; }
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.