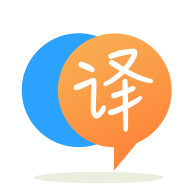
[英]How to delete a row from datagridview using c# in Windows application
[英]How to delete selected data from Excel table in dataGridView using C# Windows Form?
我想通過在 C# windows 窗體中的 dataGridView 中選擇一行來刪除一行,但我希望從 Excel 表中刪除這條記錄。 在我編寫的代碼中,只刪除了在 dataGridView 中選擇的行。 請幫我解決這個問題。
我使用以下代碼刪除 DataGridView 中的選定行。
.
.
using System.Data.OleDb;
namespace Ünistop
{
public partial class SoforİlanlarıGoruntuleForm : Form
{
public SoforİlanlarıGoruntuleForm()
{
InitializeComponent();
this.StartPosition = FormStartPosition.CenterScreen;
}
public static DataTable ExcelTabloDondur(string yol)
{
DataTable tablo = new DataTable();
string sorgu = "select*from[Sayfa1$]";
OleDbConnection baglanti = new OleDbConnection("Provider=Microsoft.Jet.OLEDB.4.0; Data Source=" + yol + ";Extended Properties=Excel 8.0;");
try
{
baglanti.Open();
OleDbCommand cekilenveri = new OleDbCommand(sorgu, baglanti);
cekilenveri.CommandTimeout = 250;
OleDbDataReader oku = cekilenveri.ExecuteReader(CommandBehavior.Default);
tablo.Load(oku);
oku.Close();
baglanti.Close();
cekilenveri.Dispose();
oku.Dispose();
baglanti.Dispose();
}
catch
{
baglanti.Close();
baglanti.Dispose();
}
return tablo;
}
private void SoforİlanlarıGoruntuleForm_Load(object sender, EventArgs e)
{
DataTable tablo = İlanAraFormu.ExcelTabloDondur("ilanlar.xls");
dataGridView1.DataSource = tablo;
}
private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
{
int secilen = dataGridView1.SelectedCells[0].RowIndex;
plakaNo_txt.Text = dataGridView1.Rows[secilen].Cells[0].Value.ToString();
aracKapasitesi_txt.Text = dataGridView1.Rows[secilen].Cells[1].Value.ToString();
sehir_txt.Text = dataGridView1.Rows[secilen].Cells[2].Value.ToString();
durak_txt.Text = dataGridView1.Rows[secilen].Cells[3].Value.ToString();
}
private void sil_btn_Click(object sender, EventArgs e)
{
string yol = "ilanlar.xls";
OleDbConnection baglanti = new OleDbConnection("Provider=Microsoft.Jet.OLEDB.4.0; Data Source =" + yol + ";Extended Properties=Excel 8.0;");
baglanti.Open();
if (DialogResult.Yes == MessageBox.Show("İlanınızı silmek istediğinize emin misiniz?", "İlan Silme", MessageBoxButtons.YesNo))
{
foreach (DataGridViewRow secilen in this.dataGridView1.SelectedRows)
{
dataGridView1.Rows.RemoveAt(secilen.Index);
MessageBox.Show("Verdiğiniz ilan silinmiştir.", "İlan Silme", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
plakaNo_txt.Clear();
aracKapasitesi_txt.Clear();
sehir_txt.Clear();
durak_txt.Clear();
}
}
}
}
.
.
using System.Data.OleDb;
namespace Ünistop
{
public partial class SoforİlanlarıGoruntuleForm : Form
{
public SoforİlanlarıGoruntuleForm()
{
InitializeComponent();
this.StartPosition = FormStartPosition.CenterScreen;
}
public static DataTable ExcelTabloDondur(string yol)
{
DataTable tablo = new DataTable();
string sorgu = "select*from[Sayfa1$]";
OleDbConnection baglanti = new OleDbConnection("Provider=Microsoft.Jet.OLEDB.4.0; Data Source=" + yol + ";Extended Properties=Excel 8.0;");
try
{
baglanti.Open();
OleDbCommand cekilenveri = new OleDbCommand(sorgu, baglanti);
cekilenveri.CommandTimeout = 250;
OleDbDataReader oku = cekilenveri.ExecuteReader(CommandBehavior.Default);
tablo.Load(oku);
oku.Close();
baglanti.Close();
cekilenveri.Dispose();
oku.Dispose();
baglanti.Dispose();
}
catch
{
baglanti.Close();
baglanti.Dispose();
}
return tablo;
}
private void SoforİlanlarıGoruntuleForm_Load(object sender, EventArgs e)
{
DataTable tablo = İlanAraFormu.ExcelTabloDondur("ilanlar.xls");
dataGridView1.DataSource = tablo;
}
private void dataGridView1_CellClick(object sender, DataGridViewCellEventArgs e)
{
int secilen = dataGridView1.SelectedCells[0].RowIndex;
plakaNo_txt.Text = dataGridView1.Rows[secilen].Cells[0].Value.ToString();
aracKapasitesi_txt.Text = dataGridView1.Rows[secilen].Cells[1].Value.ToString();
sehir_txt.Text = dataGridView1.Rows[secilen].Cells[2].Value.ToString();
durak_txt.Text = dataGridView1.Rows[secilen].Cells[3].Value.ToString();
}
private void sil_btn_Click(object sender, EventArgs e)
{
string yol = "ilanlar.xls";
OleDbConnection baglanti = new OleDbConnection("Provider=Microsoft.Jet.OLEDB.4.0; Data Source =" + yol + ";Extended Properties=Excel 8.0;");
baglanti.Open();
if (DialogResult.Yes == MessageBox.Show("İlanınızı silmek istediğinize emin misiniz?", "İlan Silme", MessageBoxButtons.YesNo))
{
foreach (DataGridViewRow secilen in this.dataGridView1.SelectedRows)
{
dataGridView1.Rows.RemoveAt(secilen.Index);
MessageBox.Show("Verdiğiniz ilan silinmiştir.", "İlan Silme", MessageBoxButtons.OK, MessageBoxIcon.Warning);
}
plakaNo_txt.Clear();
aracKapasitesi_txt.Clear();
sehir_txt.Clear();
durak_txt.Clear();
}
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.