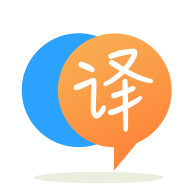
[英]searching for a particular object in array list and printing all the objects in array list
[英]Printing objects in an array list
我正在 Java 做一個醫院管理系統項目。我有 3 個班級。 一個 class 有主要方法,另一個 class 是醫院 class,它有方法打印出醫院的詳細信息,另一個方法打印出醫院的工作人員。 第三個 class 是 HospitalStaff class,它有一個用於員工所有詳細信息的 toString 方法和一個 addStaff 方法。 員工被添加到 arraylist 中。我想要打印所有員工的方法存在於醫院 class 中,我該怎么做?
這是我的代碼:
import java.util.ArrayList;
import java.util.List;
public class HospitalProject {
public static void main(String[] args) {
Hospital h = new Hospital();
h.Hospitalinformation();
HospitalStaff hs = new HospitalStaff();
hs.addHospitalStaff("A103", "Name1", "Lastname1", "Surgery", "Doctor");
hs.addHospitalStaff("A124", "Name2", "Lastname2", "Surgery", "Doctor");
}
}
/* This is the Hospital class. It has 3 methods. The HospitalInformation method prints the name and the address, name, email address and phone number of the hospital.
* It declares and array list by the name of listofStaff of the type HospitalStaff.
* It consists of a seeStaff method which is supposed to show all staff working at the hospital.
*/
class Hospital{
public String name = "Red Cross";
public String Address = "whatever";
public String phonenum = "whatever2";
private String email = "whatever@gmail.com";
public void Hospitalinformation() {
System.out.println("The name of the hospital is: " +name + " \nThe name of the Address: " + Address + "\nPhone number: "+phonenum + " \nEmail Address: " + email);
}
public void seeStaff() {
HospitalStaff hs1 = new HospitalStaff();
for(String stafflist: hs1.listofStaff) {
System.out.println(stafflist);
}
}
}
/*The Hospital Staff method consists of toString method as well as a addHospitalStaff method.
* The seeStaff method is added for debugging purposes, it is however, supposed to exist in the Hospital method.
*/
class HospitalStaff {
private String StaffId;
public String firstname;
public String lastname;
public String department;
public String stafftype;
List<String>listofStaff = new ArrayList<String>();
public void addHospitalStaff(String StaffId, String firstname, String lastname, String department, String stafftype) {
String here = StafftoString(StaffId, firstname, lastname, department, stafftype);
listofStaff.add(here);
}
public String StafftoString(String StaffId, String firstname, String lastname, String department, String stafftype) {
return String.valueOf(firstname) + " " + String.valueOf(lastname) +" " + String.valueOf(StaffId) +" " + String.valueOf(department) +" " + String.valueOf(stafftype);
}
public void seeStaff() {
for(String stafflist: listofStaff) {
System.out.println(stafflist);
}
}
}
我嘗試在 Hospital class 中創建一個名為 seeStaff() 的方法,並在該方法中創建了一個名為“hs1”的 HospitalStaff object。 然后,我使用 HospitalStaff object 調用在 HospitalStaff class 中創建的 arraylist(稱為 listofStaff)。我在 for each 循環中將其用作(String stafflist:hs1.listofStaff)。 但是,當我在 main 中創建 Hospital class object 並調用 seeStaff() 方法時,它不會打印任何內容。 我不確定為什么會這樣。
在您的seeStaff
方法中,您正在創建HospitalStaff
的新實例,創建時其中沒有任何工作人員。 因此,當您遍歷該空實例中的工作人員列表時,您不會得到 output。您在main()
中創建了另一個HospitalStaff
實例,但您不對該實例執行任何操作。
解決此問題的一種方法是首先創建您的HospitalStaff
實例。 然后,當您創建Hospital
實例時,將HospitalStaff
實例傳遞到Hospital
的構造函數中,以便它可以存儲在實例中。 然后您的showStaff
方法可以打印HospitalStaff
實例中的值。 這是它的樣子:
import java.util.ArrayList;
import java.util.List;
public class Test {
public static void main(String[] args) {
// Create an object containing the hospital's staff
HospitalStaff hs = new HospitalStaff();
hs.addHospitalStaff("A103", "Name1", "Lastname1", "Surgery", "Doctor");
hs.addHospitalStaff("A124", "Name2", "Lastname2", "Surgery", "Doctor");
// Create a Hospital object, passing it the `HospitalStaff` instance containing the hospital's staff.
Hospital h = new Hospital(hs);
h.Hospitalinformation();
h.seeStaff(); // <- Print a list of hospital staff members
}
}
/* This is the Hospital class. It has 3 methods. The HospitalInformation method prints the name and the address, name, email address and phone number of the hospital.
* It declares and array list by the name of listofStaff of the type HospitalStaff.
* It consists of a seeStaff method which is supposed to show all staff working at the hospital.
*/
class Hospital{
public String name = "Red Cross";
public String Address = "whatever";
public String phonenum = "whatever2";
private String email = "whatever@gmail.com";
private HospitalStaff hs;
public Hospital(HospitalStaff hs) {
this.hs = hs; // <- Associate the passed in HospitalStaff instance with this `Hospital` instance.
}
public void Hospitalinformation() {
System.out.println("The name of the hospital is: " +name + " \nThe name of the Address: " + Address + "\nPhone number: "+phonenum + " \nEmail Address: " + email);
}
// Print the members of the hospital's staff
public void seeStaff() {
for(String stafflist: hs.listofStaff) {
System.out.println(stafflist);
}
}
}
/*The Hospital Staff method consists of toString method as well as a addHospitalStaff method.
* The seeStaff method is added for debugging purposes, it is however, supposed to exist in the Hospital method.
*/
class HospitalStaff {
private String StaffId;
public String firstname;
public String lastname;
public String department;
public String stafftype;
List<String>listofStaff = new ArrayList<String>();
public void addHospitalStaff(String StaffId, String firstname, String lastname, String department, String stafftype) {
String here = StafftoString(StaffId, firstname, lastname, department, stafftype);
listofStaff.add(here);
}
public String StafftoString(String StaffId, String firstname, String lastname, String department, String stafftype) {
return String.valueOf(firstname) + " " + String.valueOf(lastname) +" " + String.valueOf(StaffId) +" " + String.valueOf(department) +" " + String.valueOf(stafftype);
}
public void seeStaff() {
for(String stafflist: listofStaff) {
System.out.println(stafflist);
}
}
}
結果:
The name of the hospital is: Red Cross
The name of the Address: whatever
Phone number: whatever2
Email Address: whatever@gmail.com
Name1 Lastname1 A103 Surgery Doctor
Name2 Lastname2 A124 Surgery Doctor
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.