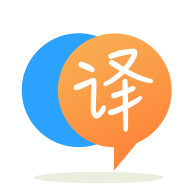
[英]How much logic should i put my repository methods when using repository pattern?
[英]How should I pass the author of the change into PUT/POST methods?
我有一個類別 class,它看起來像這樣:
public class Category
{
[Required]
public Guid Id { get; set; }
[Required]
public string CategoryName { get; set; }
public string CategoryDescription { get; set; }
public DateTime CreatedDate { get; set; }
public bool isDeleted { get; set; } = false;
public virtual ICollection<UpdateInfo> Updates { get; set; } = new List<UpdateInfo>();
}
和一個 UpdateInfo class 看起來像這樣(帶有枚舉):
public enum Status
{
Created,
Changed,
Deleted
}
public class UpdateInfo
{
public Guid Id { get; set; }
public string Author { get; set; }
public DateTime Date { get; set; }
public Status Status { get; set; }
public virtual Category Category { get; set; }
}
我正在尋找一種正確的方法將作者與從主體傳遞的 Id 或 CategoryDTO 一起傳遞到 PUT/POST/DELETE 方法。 我首先嘗試使用 POST 方法,我需要一個意見,以及執行此操作的正確方法。
[HttpPost]
[ProducesResponseType(StatusCodes.Status201Created)]
[ProducesResponseType(StatusCodes.Status400BadRequest)]
[ProducesResponseType(StatusCodes.Status409Conflict)]
public async Task<ActionResult<Category>> PostCategory(CategoryDTO categoryDTO, string author)
{
_logger.LogInformation("Creating a new category DB object from a DTO objcect passed in PostOperation method.");
var category = _mapper.Map<CategoryDTO, Category>(categoryDTO);
if (CategoryRepeat(category.CategoryName))
{
return Conflict("Such category already exists.");
}
_logger.LogInformation("Adding an initial update status.");
var initialUpdate = new UpdateInfo { Author = author, Status = Status.Created, Date = DateTime.UtcNow, Id = Guid.NewGuid(), Category = category };
category.Updates.Add(initialUpdate);
try
{
_logger.LogInformation($"Trying to save created category {category.Id} into the Database.");
_context.Categories.Add(category);
await _context.SaveChangesAsync();
_logger.LogInformation($"Created category id: {category.Id} saved into the Database successfully.");
}
catch (DbUpdateConcurrencyException ex)
{
_logger.LogError(ex.Message);
return BadRequest(new { message = ex.Message });
}
return CreatedAtAction("GetCategory", new { id = category.Id }, category);
}
如果您需要在某些請求中傳遞作者而不是其他請求,那么通過將它作為單獨的變量傳遞來實現的方式可能會更靈活。 所以你做得很好。 如果您發現自己到處都是作者,最好改為更改 CategoryDTO model。
另一種方法是為您要查找的特定操作創建另一個 DTO。 例如,用於“創建”POST 請求的 CreateCategoryDTO 並在那里更改 model。 這實際上取決於您的編碼風格和項目的整個“編碼標准”。
如果我正確理解你的問題,你也可以在請求 header 中傳遞author
。你可以創建一個自定義 header 並在發送請求時設置它,然后你可以獲得像string author = Request.Headers["Header-Author"];
另一種方法是將屬性添加到現有的CategoryDTO
本身。 然后得到它像string author = categoryDTO.Author;
一種方法是像您一樣通過作為單獨的參數傳遞。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.