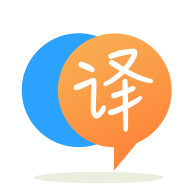
[英]How do I resolve collection error with my controller in ASP.NET MVC in C#?
[英]C# ASP.Net How do I give my sqlite database connection to the home controller
我正在嘗試制作一個非常簡單的應用程序,其中我使用 sqlite 數據庫。 我使用存儲庫模式來處理所有數據庫調用。 我想把這個存儲庫給我的 controller。
Controller
using Microsoft.AspNetCore.Components;
using Microsoft.AspNetCore.Mvc;
using Microsoft.AspNetCore.Mvc.Routing;
using WebApplication2.Models;
using WebApplication2.Data;
namespace WebApplication2.Controllers
{
//[Microsoft.AspNetCore.Mvc.Route("/Addresses")]
[ApiController]
public class AddressController : Controller
{
private readonly AddressRepository _addressReposity;
public AddressController(AddressRepository addressRepository)
{
_addressReposity = addressRepository;
}
[HttpGet("/addresses")]
[ProducesResponseType(200, Type = typeof(List<(int, Address)>))]
public IActionResult getAddresses(Address address, bool orderByAscending)
{
List<(int, Address)> addresses = _addressReposity.getAddresses(address, orderByAscending);
if (!ModelState.IsValid)
return BadRequest(ModelState);
return Ok(addresses);
}
[HttpPost("/addresses")]
[ValidateAntiForgeryToken]
public IActionResult addAddress(Address address)
{
return View();
}
[HttpGet("/addresses/{id}")]
[ProducesResponseType(200, Type = typeof(Address))]
public IActionResult getSingleAddress(int id)
{
if(!_addressReposity.existAddress(id))
return BadRequest(ModelState);
if (!ModelState.IsValid)
return BadRequest(ModelState);
return Ok(_addressReposity.getSingleAddress(id));
}
[HttpPut("/addresses/{id}")]
public IActionResult updateAddress(Address address, int id)
{
if (!_addressReposity.existAddress(id))
return BadRequest(ModelState);
if (!ModelState.IsValid)
return BadRequest(ModelState);
_addressReposity.UpdateAddress(address, id);
return Ok();
}
[HttpDelete("/addresses/{id}")]
public IActionResult deleteAddress(int id)
{
if (!_addressReposity.existAddress(id))
return BadRequest(ModelState);
if (!ModelState.IsValid)
return BadRequest(ModelState);
return Ok();
}
}
}
用於處理數據庫調用的存儲庫
using System.Data;
using WebApplication2.Models;
using Microsoft.Data.Sqlite;
using Microsoft.EntityFrameworkCore.Sqlite;
using System.Net;
namespace WebApplication2.Data
{
//TODO: REGEX expressions in order to make sure no SQL injection happens on the input or use a library for that
public class AddressRepository : IAddressRepository
{
private readonly SqliteConnection _db_connection;
public AddressRepository(SqliteConnection db_connection)
{
_db_connection = db_connection;
}
public List<(int, Address)> readerToAddresses(SqliteDataReader reader)
{
List<(int, Address)> addresses = new List<(int, Address)>();
while (reader.Read())
{
int ID = reader.GetInt32("ID");
string street = reader[2].ToString();
string city = reader[3].ToString();
string zipcode = reader[4].ToString();
int houseNumber = (int)reader[5];
string addition;
if (reader[6] == null)
addition = null;
else
addition = reader[6].ToString();
addresses.Append((ID, new Address(street, city, zipcode, houseNumber)));
}
return addresses;
}
public string getSearchAddressesString(string start, Address search_info)
{
string search_command_string = start;
// In case the search command is not null loop through all non null properties and add them to the search
if (search_info != null)
{
foreach (var prop in search_info.GetType().GetProperties())
{
if (prop.GetValue(search_info) != null)
{
if (prop.PropertyType.Name == "String")
{
search_command_string += prop.Name.ToString() + " = '" + prop.GetValue(search_info).ToString() + "'" + ", ";
}
else if (prop.PropertyType.Name == "Int32")
{
search_command_string += prop.Name.ToString() + " = " + ((int)prop.GetValue(search_info)).ToString() + ", ";
}
}
}
}
return search_command_string;
}
public List<(int, Address)> getAddresses(Address search_info, bool orderByAscending)
{
// Initialize search_command in case there is no info in the address to search on
string search_command_string = $"SELECT * FROM ADDRESSES WHERE ";
// In case the search command is not null loop through all non null properties and add them to the search
search_command_string = getSearchAddressesString(search_command_string, search_info);
// In case there isn't any info to search on just return the entire table
if (search_command_string == "SELECT * FROM ADDRESSES WHERE ")
search_command_string = "SELECT * FROM ADDRESSES";
// Execute command
SqliteCommand search_command = new SqliteCommand(search_command_string, _db_connection);
SqliteDataReader reader = search_command.ExecuteReader();
return readerToAddresses(reader);
}
public void InsertAddress(Address address)
{
string insert_command_string_part_one = "INSERT INTO ADDRESSES (";
string insert_command_string_part_two = "VALUES(";
if (address != null)
{
foreach (var prop in address.GetType().GetProperties())
{
insert_command_string_part_one += prop.Name.ToString() + ", ";
if (prop.GetValue(address) != null)
{
if (prop.PropertyType.Name == "String")
{
insert_command_string_part_two += "'" + prop.GetValue(address).ToString() + "'" + ", ";
}
else if (prop.PropertyType.Name == "Int32")
{
insert_command_string_part_two += ((int)prop.GetValue(address)).ToString() + ", ";
}
}
}
}
insert_command_string_part_one = insert_command_string_part_one.Substring(0, insert_command_string_part_one.Length - 2) + ") ";
insert_command_string_part_two = insert_command_string_part_two.Substring(0, insert_command_string_part_two.Length - 2) + ')';
SqliteCommand insert_command = new SqliteCommand(insert_command_string_part_one + insert_command_string_part_two, _db_connection);
insert_command.ExecuteNonQuery();
}
public void UpdateAddress(Address address, int id)
{
bool not_all_null = false;
string update_command_string = "UPDATE ADDRESSES SET ";
if (address != null)
{
foreach (var prop in address.GetType().GetProperties())
{
if (prop.GetValue(address) != null)
{
not_all_null = true;
if (prop.PropertyType.Name == "String")
{
update_command_string += prop.Name.ToString() + " = '" + prop.GetValue(address).ToString() + "'" + ", ";
}
else if (prop.PropertyType.Name == "Int32")
{
update_command_string += prop.Name.ToString() + " = " + ((int)prop.GetValue(address)).ToString() + ", ";
}
}
}
if (not_all_null)
{
update_command_string = update_command_string.Substring(0, update_command_string.Length - 2) + $" WHERE ID = {(int)id}";
SqliteCommand update_command = new SqliteCommand(update_command_string, _db_connection);
update_command.ExecuteNonQuery();
}
}
}
public void DeleteAddress(int id)
{
string delete_command_string = $"DELETE FROM ADDRESSES WHERE ID = {(int)id}";
SqliteCommand delete_command = new SqliteCommand(delete_command_string, _db_connection);
delete_command.ExecuteNonQuery();
}
public bool existAddress(int id)
{
string exists_command_string = $"SELECT * FROM ADDRESSES WHERE ID={id}";
SqliteCommand exists_command = new SqliteCommand(exists_command_string, _db_connection);
SqliteDataReader reader = exists_command.ExecuteReader();
while (reader.Read())
return true;
return false;
}
public Address getSingleAddress(int id)
{
string get_address_string = $"SELECT * FROM ADDRESSES WHERE ID={id}";
SqliteCommand single_address_command = new SqliteCommand(get_address_string, _db_connection);
SqliteDataReader reader = single_address_command.ExecuteReader();
return readerToAddresses(reader)[0].Item2;
}
}
}
這也是我的 appsettings.json 因為我看到它提到了很多:
{
"ConnectionStrings": {
"DefaultConnection": "Addresses.sqlite"
},
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*"
}
我只是不知道如何進行。 我會喜歡一些關於如何處理這個問題的建議。
您需要告訴框架AddressRepository
和SqliteConnection
是應該注入的服務。
像這樣的東西,在你的Program.cs
中:
builder.Services.AddScoped<IAddressRepository, AddressRepository>();
builder.Services.AddScoped<SqliteConnection>(() => new SqliteConnection(builder.Configuration.GetConnectionString("DefaultConnection")));
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.