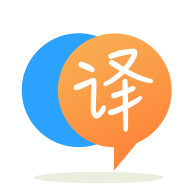
[英]How do I display my datagrid view items to textboxes in a another form?
[英]Foreach Control in form, how can I do something to all the TextBoxes in my Form?
如何使用 Foreach 語句對我的文本框執行某些操作?
foreach (Control X in this.Controls)
{
Check if the controls is a TextBox, if it is delete it's .Text letters.
}
如果您使用的是 C# 3.0 或更高版本,您可以執行以下操作
foreach ( TextBox tb in this.Controls.OfType<TextBox>()) {
..
}
如果沒有 C# 3.0,您可以執行以下操作
foreach ( Control c in this.Controls ) {
TextBox tb = c as TextBox;
if ( null != tb ) {
...
}
}
或者甚至更好,用 C# 2.0 編寫 OfType。
public static IEnumerable<T> OfType<T>(IEnumerable e) where T : class {
foreach ( object cur in e ) {
T val = cur as T;
if ( val != null ) {
yield return val;
}
}
}
foreach ( TextBox tb in OfType<TextBox>(this.Controls)) {
..
}
您正在尋找
foreach (Control x in this.Controls)
{
if (x is TextBox)
{
((TextBox)x).Text = String.Empty;
}
}
這里的技巧是Controls
不是List<>
或IEnumerable
而是ControlCollection
。
我建議使用 Control 的擴展,它會返回更多..可查詢的內容;)
public static IEnumerable<Control> All(this ControlCollection controls)
{
foreach (Control control in controls)
{
foreach (Control grandChild in control.Controls.All())
yield return grandChild;
yield return control;
}
}
然后你可以這樣做:
foreach(var textbox in this.Controls.All().OfType<TextBox>)
{
// Apply logic to the textbox here
}
foreach (Control X in this.Controls)
{
if (X is TextBox)
{
(X as TextBox).Text = string.Empty;
}
}
您也可以使用 LINQ。 例如,對於清除Textbox
文本,請執行以下操作:
this.Controls.OfType<TextBox>().ToList().ForEach(t => t.Text = string.Empty);
您可以執行以下操作:
foreach (Control X in this.Controls)
{
TextBox tb = X as TextBox;
if (tb != null)
{
string text = tb.Text;
// Do something to text...
tb.Text = string.Empty; // Clears it out...
}
}
很多上面的工作。 只是為了補充。 如果您的文本框不直接位於窗體上,而是位於其他容器對象(如 GroupBox)上,則必須獲取 GroupBox 對象,然后遍歷 GroupBox 以訪問其中包含的文本框。
foreach(Control t in this.Controls.OfType<GroupBox>())
{
foreach (Control tt in t.Controls.OfType<TextBox>())
{
// do stuff
}
}
foreach (Control x in this.Controls)
{
if (x is TextBox)
{
((TextBox)x).Text = String.Empty;
//instead of above line we can use
*** x.resetText();
}
}
檢查這個:
foreach (Control x in this.Controls)
{
if (x is TextBox)
{
x.Text = "";
}
}
簡單使用 linq,根據您認為適合您處理的任何控件進行更改。
private void DisableButtons()
{
foreach (var ctl in Controls.OfType<Button>())
{
ctl.Enabled = false;
}
}
private void EnableButtons()
{
foreach (var ctl in Controls.OfType<Button>())
{
ctl.Enabled = true;
}
}
只需添加其他控件類型:
public static void ClearControls(Control c)
{
foreach (Control Ctrl in c.Controls)
{
//Console.WriteLine(Ctrl.GetType().ToString());
//MessageBox.Show ( (Ctrl.GetType().ToString())) ;
switch (Ctrl.GetType().ToString())
{
case "System.Windows.Forms.CheckBox":
((CheckBox)Ctrl).Checked = false;
break;
case "System.Windows.Forms.TextBox":
((TextBox)Ctrl).Text = "";
break;
case "System.Windows.Forms.RichTextBox":
((RichTextBox)Ctrl).Text = "";
break;
case "System.Windows.Forms.ComboBox":
((ComboBox)Ctrl).SelectedIndex = -1;
((ComboBox)Ctrl).SelectedIndex = -1;
break;
case "System.Windows.Forms.MaskedTextBox":
((MaskedTextBox)Ctrl).Text = "";
break;
case "Infragistics.Win.UltraWinMaskedEdit.UltraMaskedEdit":
((UltraMaskedEdit)Ctrl).Text = "";
break;
case "Infragistics.Win.UltraWinEditors.UltraDateTimeEditor":
DateTime dt = DateTime.Now;
string shortDate = dt.ToShortDateString();
((UltraDateTimeEditor)Ctrl).Text = shortDate;
break;
case "System.Windows.Forms.RichTextBox":
((RichTextBox)Ctrl).Text = "";
break;
case " Infragistics.Win.UltraWinGrid.UltraCombo":
((UltraCombo)Ctrl).Text = "";
break;
case "Infragistics.Win.UltraWinEditors.UltraCurrencyEditor":
((UltraCurrencyEditor)Ctrl).Value = 0.0m;
break;
default:
if (Ctrl.Controls.Count > 0)
ClearControls(Ctrl);
break;
}
}
}
更好的是,您可以封裝它以在一種方法中清除您想要的任何類型的控件,如下所示:
public static void EstadoControles<T>(object control, bool estado, bool limpiar = false) where T : Control
{
foreach (var textEdits in ((T)control).Controls.OfType<TextEdit>()) textEdits.Enabled = estado;
foreach (var textLookUpEdits in ((T)control).Controls.OfType<LookUpEdit>()) textLookUpEdits.Enabled = estado;
if (!limpiar) return;
{
foreach (var textEdits in ((T)control).Controls.OfType<TextEdit>()) textEdits.Text = string.Empty;
foreach (var textLookUpEdits in ((T)control).Controls.OfType<LookUpEdit>()) textLookUpEdits.EditValue = @"-1";
}
}
private IEnumerable<TextBox> GetTextBoxes(Control control)
{
if (control is TextBox textBox)
{
yield return textBox;
}
if (control.HasChildren)
{
foreach (Control ctr in control.Controls)
{
foreach (var textbox in GetTextBoxes(ctr))
{
yield return textbox;
}
}
}
}
我發現這工作得很好,但最初我在面板上有我的文本框,所以我的文本框都沒有按預期清除,所以我添加了
this.panel1.Controls.....
這讓我想到,你有很多不同功能的文本框,只有一些需要清除,也許使用多個面板層次結構,你可以只針對少數而不是全部。
foreach ( TextBox tb in this.Controls.OfType<TextBox>()) {
..
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.