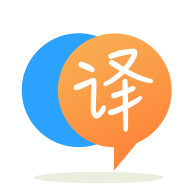
[英]"SocketException: Could not resolve host" when connecting to SFTP server with SSH.NET
[英]SocketException when connecting to server
我在同一台計算機上同時運行客戶端和服務器。 有1個人知道上述錯誤嗎?
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Threading;
using System.Net.Sockets;
using System.IO;
using System.Net;
namespace Server
{
public partial class Server : Form
{
private Socket connection;
private Thread readThread;
private NetworkStream socketStream;
private BinaryWriter writer;
private BinaryReader reader;
//default constructor
public Server()
{
InitializeComponent();
//create a new thread from server
readThread = new Thread(new ThreadStart(RunServer));
readThread.Start();
}
protected void Server_Closing(object sender, CancelEventArgs e)
{
System.Environment.Exit(System.Environment.ExitCode);
}
//sends the text typed at the server to the client
protected void inputText_KeyDown(object sender, KeyEventArgs e)
{
// send the text to client
try
{
if (e.KeyCode == Keys.Enter && connection != null)
{
writer.Write("Server>>> " + inputText.Text);
displayText.Text +=
"\r\nSERVER>>> " + inputText.Text;
//if user at server enter terminate
//disconnect the connection to the client
if (inputText.Text == "TERMINATE")
connection.Close();
inputText.Clear();
}
}
catch (SocketException)
{
displayText.Text += "\nError writing object";
}
}//inputTextBox_KeyDown
// allow client to connect & display the text it sends
public void RunServer()
{
TcpListener listener;
int counter = 1;
//wait for a client connection & display the text client sends
try
{
//step 1: create TcpListener
IPAddress ipAddress = Dns.Resolve("localhost").AddressList[0];
TcpListener tcplistener = new TcpListener(ipAddress, 9000);
//step 2: TcpListener waits for connection request
tcplistener.Start();
//step 3: establish connection upon client request
while (true)
{
displayText.Text = "waiting for connection\r\n";
// accept incoming connection
connection = tcplistener.AcceptSocket();
//create NetworkStream object associated with socket
socketStream = new NetworkStream(connection);
//create objects for transferring data across stream
writer = new BinaryWriter(socketStream);
reader = new BinaryReader(socketStream);
displayText.Text += "Connection " + counter + " received.\r\n ";
//inform client connection was successful
writer.Write("SERVER>>> Connection successful");
inputText.ReadOnly = false;
string theReply = "";
// step 4: read string data sent from client
do
{
try
{
//read the string sent to the server
theReply = reader.ReadString();
// display the message
displayText.Text += "\r\n" + theReply;
}
// handle the exception if error reading data
catch (Exception)
{
break;
}
} while (theReply != "CLIENT>>> TERMINATE" && connection.Connected);
displayText.Text +=
"\r\nUser terminated connection";
// step 5: close connection
inputText.ReadOnly = true;
writer.Close();
reader.Close();
socketStream.Close();
connection.Close();
++counter;
}
} //end try
catch (Exception error)
{
MessageBox.Show(error.ToString());
}
}
}// end method runserver
}// end class server
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.Threading;
using System.Net.Sockets;
using System.IO;
using System.Net;
namespace Client
{
public partial class Client : Form
{
private NetworkStream output;
private BinaryWriter writer;
private BinaryReader reader;
private string message = "";
private Thread readThread;
//default constructor
public Client()
{
InitializeComponent();
readThread = new Thread(new ThreadStart(RunClient));
readThread.Start();
}
protected void Client_Closing(
object sender, CancelEventArgs e)
{
System.Environment.Exit(System.Environment.ExitCode);
}
//sends the text user typed to server
protected void inputText_KeyDown(
object sender, KeyEventArgs e)
{
try
{
if (e.KeyCode == Keys.Enter)
{
writer.Write("CLIENT>>> " + inputText.Text);
displayText.Text +=
"\r\nCLIENT>>> " + inputText.Text;
inputText.Clear();
}
}
catch (SocketException ioe)
{
displayText.Text += "\nError writing object";
}
}//end method inputText_KeyDown
//connect to server & display server-generated text
public void RunClient()
{
TcpClient client;
//instantiate TcpClient for sending data to server
try
{
displayText.Text += "Attempting connection\r\n";
//step1: create TcpClient for sending data to server
client = new TcpClient();
client.Connect("localhost", 9000);
//step2: get NetworkStream associated with TcpClient
output = client.GetStream();
//create objects for writing & reading across stream
writer = new BinaryWriter(output);
reader = new BinaryReader(output);
displayText.Text += "\r\nGot I/O streams\r\n";
inputText.ReadOnly = false;
//loop until server terminate
do
{
//step3: processing phase
try
{
//read from server
message = reader.ReadString();
displayText.Text += "\r\n" + message;
}
//handle exception if error in reading server data
catch (Exception)
{
System.Environment.Exit(System.Environment.ExitCode);
}
} while (message != "SERVER>>> TERMINATE");
displayText.Text += "\r\nClosing connection.\r\n";
//step4: close connection
writer.Close();
reader.Close();
output.Close();
client.Close();
Application.Exit();
}
catch (Exception error)
{
MessageBox.Show(error.ToString());
}
}
}
}
這可能是您的防火牆正在起作用。 嘗試連接到TCP 80上的www.google.com之類的東西,以查看是否可以實際連接。
您是否正在使用Windows的較新版本? 您可能只在偵聽IPv4,但“本地主機”正在解析為IPv6地址,但找不到它。 嘗試連接到“ 127.0.0.1”而不是localhost,然后查看結果是否更改。
mk,我將tcplistener / tcpclient用於簡單的應用程序。 。 。 翡翠變色龍
如果您將該構造函數與TCPListener一起使用,則它將使基礎服務提供商選擇一個網絡地址,該地址可能不是“ localhost”。 您可能正在偵聽LAN / WLAN卡而不是本地主機。
看一下TCPListener的MSDN頁面,那里的示例展示了如何使用其他構造函數,其他示例則查看其他構造函數。
這是一種方法:
IPAddress ipAddress = Dns.Resolve("localhost").AddressList[0];
TcpListener tcpListener = new TcpListener(ipAddress, 9000);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.