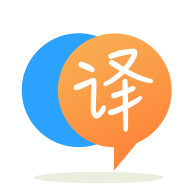
[英]com.mysql.jdbc.exceptions.jdbc4.CommunicationsException:
[英]com.mysql.jdbc.exceptions.jdbc4.CommunicationsException when using JDBC to access remote MySQL database
/**
*
*/
package ORM;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
/**
* @author Gwilym
* @version 0.0
*/
public class DatabaseConnection {
private String userName="";
private String password="";
private String host="";
Connection conn;
/**
* @param userName
* @param password
* @param host
*/
public DatabaseConnection(String userName, String password, String host) {
this.userName = userName;
this.password = password;
this.host = host;
}
public DatabaseConnection(String userName, String password, String host,boolean autoConnect) {
this.userName = userName;
this.password = password;
this.host = host;
if (autoConnect)
{
try {
Connect();
} catch (DatabaseConnectionException e) {
e.printStackTrace();
}
}
}
/**
* @return the connection
*/
public Connection getConn() {
return conn;
}
/**
* @param userName the userName to set
*/
public void setUserName(String userName) {
this.userName = userName;
}
/**
* @param password the password to set
*/
public void setPassword(String password) {
this.password = password;
}
/**
* @param host the host to set
*/
public void setHost(String host) {
this.host = host;
}
/**
* Connect, attempts to connect to the MySQL database
* with sun JDBC
* & MySQL driver
* @param none
* @return True iff connected;
* @return False for all else;
* @throws DatabaseConnectionException
*/
public boolean Connect() throws DatabaseConnectionException
{
// Attempt to load database driver
try
{
String url = "jdbc:mysql:"+host;
System.out.println(url);
//Load driver
Class.forName ("com.mysql.jdbc.Driver").newInstance ();
conn = DriverManager.getConnection (url, userName, password);
}
catch (ClassNotFoundException cnfe) // driver not found
{
conn=null;
System.err.println ("Unable to load database driver");
throw new DatabaseConnectionException(cnfe);
}
catch(InstantiationException ie)
{
conn=null;
System.err.println ("Unable to Create database driver");
throw new DatabaseConnectionException(ie);
}
catch (IllegalAccessException iae)
{
conn=null;
System.err.println ("Unable to Create database driver");
throw new DatabaseConnectionException(iae);
} catch (SQLException sqle) {
conn=null;
System.err.println ("SQL error");
throw new DatabaseConnectionException(sqle);
}
if (conn!=null)
{
System.out.println ("Database connection established");
return true;
}
else
{
System.out.println ("Database connection Failed");
return false;
}
}
/**
* Disconnects the System from the mySQL database
*
* @param none
* @return true, if successful
* @return false if not connection in existance
*/
public boolean Disconnect()
{
if (conn != null)
{
try
{
conn.close ();
conn=null;
System.out.println ("Database connection terminated normally");
return true;
}
catch (Exception e) {
//Ignore these errors as they all result in conn.close anyway
}
finally
{
conn=null;
System.gc();
// my removing the refrance to conncetion all calling the Garbage collecter we insure it is destoryed.
}
System.out.println ("Database connection terminated with errors");
return true;
}
else
{
System.out.println ("No Database connection present");
return true;
}
}
}
上面的代碼叫做
DatabaseConnection db =new DatabaseConnection("USERNAME","PASSWORD","//tel2.dur.ac.uk:3306/dcs8s07_SEG",true);
由於顯而易見的原因,我刪除了用戶名和密碼,但它們可以被認為是正確的。
直到問題它自己我得到一個com.mysql.jdbc.exceptions.jdbc4.CommunicationsException什么時候這個代碼運行的詳細信息“成功發送到服務器的最后一個數據包是0毫秒前。驅動程序沒有收到任何來自服務器的數據包。“
我目前的主要問題是試圖發現究竟出了什么問題。
到目前為止,我可以告訴驅動程序正確加載,因為我的代碼不會拋出ClassNotFoundException,而是拋出某種類型的SQLException。
所以問題幾乎肯定是某種方式的聯系。 我可以通過位於同一服務器上的phpMyadmin連接和查詢這個數據庫,所以我可以假設
1)服務器在線2)mySQL正在工作3)用戶名和密碼是正確的4)數據庫存在,我的名字是正確的
由此和“驅動程序沒有從服務器收到任何數據包。” 我想知道網址是否格式錯誤?
URL = jdbc:mysql://tel2.dur.ac.uk:3306 / dcs8s07_SEG
或者有一個簡單的設置在服務器上是不正確的,而不允許我連接?
我一直在思考這個問題並嘗試了幾個谷歌無濟於事,所以任何想法都會有很大的幫助
提前謝謝!
這是一個包裝異常。 這個例外的根本原因是什么? 在堆棧跟蹤中進一步查看。
一個非常常見的根本原因是java.net.ConnectException: Connection refused
。 我在近99%的案例中都看到了這一點。 如果在您的情況下也是如此,那么所有可能的原因是:
要解決其中一個問題,請遵循以下建議:
ping
驗證並測試它們。 my.cnf
進行驗證。 --skip-networking
選項的情況下啟動mysqld。 用戶名和密碼與此問題無關。 此時甚至無法訪問DB。 否則您將獲得“登錄失敗”或“未授權” SQLException
。
除了上一篇文章,您還應該進行低級別的telnet測試,這是驗證連接性的最佳方法。 此測試將告訴您是否存在阻止訪問該端口的防火牆或其他軟件。
telnet tel2.dur.ac.uk 3306
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.