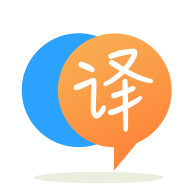
[英]Auto highlight the text in all textbox control in a winform upon enter(gained focus)
[英]Auto highlight text in a textbox control
當控件獲得焦點時,如何自動突出顯示文本框控件中的文本。
在 Windows 窗體和 WPF 中:
textbox.SelectionStart = 0;
textbox.SelectionLength = textbox.Text.Length;
如果您想為整個 WPF 應用程序執行此操作,您可以執行以下操作: - 在文件 App.xaml.cs 中
protected override void OnStartup(StartupEventArgs e)
{
//works for tab into textbox
EventManager.RegisterClassHandler(typeof(TextBox),
TextBox.GotFocusEvent,
new RoutedEventHandler(TextBox_GotFocus));
//works for click textbox
EventManager.RegisterClassHandler(typeof(Window),
Window.GotMouseCaptureEvent,
new RoutedEventHandler(Window_MouseCapture));
base.OnStartup(e);
}
private void TextBox_GotFocus(object sender, RoutedEventArgs e)
{
(sender as TextBox).SelectAll();
}
private void Window_MouseCapture(object sender, RoutedEventArgs e)
{
var textBox = e.OriginalSource as TextBox;
if (textBox != null)
textBox.SelectAll();
}
在 ASP.NET 中:
textbox.Attributes.Add("onfocus","this.select();");
使用內置方法SelectAll
很容易實現
簡單的你可以這樣寫:
txtTextBox.Focus();
txtTextBox.SelectAll();
文本框中的所有內容都將被選中:)
如果您的目的是在單擊鼠標時突出顯示文本框中的文本,您可以通過添加以下內容使其變得簡單:
this.textBox1.Click += new System.EventHandler(textBox1_Click);
在:
partial class Form1
{
private void InitializeComponent()
{
}
}
其中 textBox1 是位於 Form1 中的相關文本框的名稱
然后創建方法定義:
void textBox1_Click(object sender, System.EventArgs e)
{
textBox1.SelectAll();
}
在:
public partial class Form1 : Form
{
}
我認為最簡單的方法是在 Enter 事件中使用TextBox.SelectAll
:
private void TextBox_Enter(object sender, EventArgs e)
{
((TextBox)sender).SelectAll();
}
這是我一直在使用的代碼。 它需要將附加屬性添加到您希望自動選擇的每個文本框。 鑒於我不希望我的應用程序中的每個文本框都這樣做,這對我來說是最好的解決方案。
public class AutoSelectAll
{
public static bool GetIsEnabled(DependencyObject obj)
{
return (bool)obj.GetValue(IsEnabledProperty);
}
public static void SetIsEnabled(DependencyObject obj, bool value)
{
obj.SetValue(IsEnabledProperty, value);
}
static void ue_Loaded(object sender, RoutedEventArgs e)
{
var ue = sender as FrameworkElement;
if (ue == null)
return;
ue.GotFocus += ue_GotFocus;
ue.GotMouseCapture += ue_GotMouseCapture;
}
private static void ue_Unloaded(object sender, RoutedEventArgs e)
{
var ue = sender as FrameworkElement;
if (ue == null)
return;
//ue.Unloaded -= ue_Unloaded;
ue.GotFocus -= ue_GotFocus;
ue.GotMouseCapture -= ue_GotMouseCapture;
}
static void ue_GotFocus(object sender, RoutedEventArgs e)
{
if (sender is TextBox)
{
(sender as TextBox).SelectAll();
}
e.Handled = true;
}
static void ue_GotMouseCapture(object sender, MouseEventArgs e)
{
if (sender is TextBox)
{
(sender as TextBox).SelectAll();
}
e.Handled = true;
}
public static readonly DependencyProperty IsEnabledProperty = DependencyProperty.RegisterAttached("IsEnabled", typeof(bool),
typeof(AutoSelectAll), new UIPropertyMetadata(false, IsEnabledChanged));
static void IsEnabledChanged(DependencyObject d, DependencyPropertyChangedEventArgs e)
{
var ue = d as FrameworkElement;
if (ue == null)
return;
if ((bool)e.NewValue)
{
ue.Unloaded += ue_Unloaded;
ue.Loaded += ue_Loaded;
}
}
}
我在這里所做的主要更改是向我看到的許多示例添加了一個加載事件。 這允許代碼在卸載后繼續工作(即更改選項卡)。 此外,我還包含了代碼,以確保在您用鼠標單擊文本框時選中文本,而不僅僅是鍵盤聚焦它。 注意:如果您實際單擊文本框中的文本,光標會按原樣插入字母之間。
您可以通過在 xaml 中包含以下標記來使用它。
<TextBox
Text="{Binding Property}"
Library:AutoSelectAll.IsEnabled="True" />
如果您需要對大量文本框(在 Silverlight 或 WPF 中)執行此操作,則可以使用博客文章中使用的技術: http : //dnchannel.blogspot.com/2010/01/silverlight-3-auto -select-text-in.html 。 它使用附加屬性和路由事件。
你可以用這個,精辟。 :D
TextBox1.Focus();
TextBox1.Select(0, TextBox1.Text.Length);
如果您只想在用戶第一次單擊框時選擇所有文本,然后讓他們根據需要單擊文本中間,這就是我最終使用的代碼。
僅處理FocusEnter
事件是行不通的,因為Click
事件隨后發生,並且如果您在 Focus 事件中使用SelectAll()
會覆蓋選擇。
private bool isFirstTimeEntering;
private void textBox_Enter(object sender, EventArgs e)
{
isFirstTimeEntering = true;
}
private void textBox_Click(object sender, EventArgs e)
{
switch (isFirstTimeEntering)
{
case true:
isFirstTimeEntering = false;
break;
case false:
return;
}
textBox.SelectAll();
textBox.SelectionStart = 0;
textBox.SelectionLength = textBox.Text.Length;
}
如果您想在“ On_Enter 事件”上全選,這將無助於您實現目標。 嘗試使用“On_Click 事件”
private void textBox_Click(object sender, EventArgs e)
{
textBox.Focus();
textBox.SelectAll();
}
在事件“Enter”(例如:按 Tab 鍵)或“第一次單擊”時,所有文本都將被選中。 網絡 4.0
public static class TbHelper
{
// Method for use
public static void SelectAllTextOnEnter(TextBox Tb)
{
Tb.Enter += new EventHandler(Tb_Enter);
Tb.Click += new EventHandler(Tb_Click);
}
private static TextBox LastTb;
private static void Tb_Enter(object sender, EventArgs e)
{
var Tb = (TextBox)sender;
Tb.SelectAll();
LastTb = Tb;
}
private static void Tb_Click(object sender, EventArgs e)
{
var Tb = (TextBox)sender;
if (LastTb == Tb)
{
Tb.SelectAll();
LastTb = null;
}
}
}
我不知道為什么沒有人提到,但你也可以這樣做,它對我有用
textbox.Select(0, textbox.Text.Length)
textBoxX1.Focus();
this.ActiveControl = textBoxX1;
textBoxX1.SelectAll();
在窗口形式 c# 中。 如果您使用 Enter 事件,它將不起作用。 嘗試使用 MouseUp 事件
bool FlagEntered;
private void textBox1_MouseUp(object sender, MouseEventArgs e)
{
if ((sender as TextBox).SelectedText == "" && !FlagEntered)
{
(sender as TextBox).SelectAll();
FlagEntered = true;
}
}
private void textBox1_Leave(object sender, EventArgs e)
{
FlagEntered = false;
}
textbox.Focus();
textbox.SelectionStart = 0;
textbox.SelectionLength = textbox.Text.Length;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.