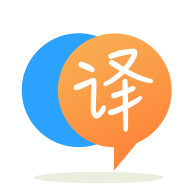
[英]Continuous Communication between C# and C++ application using Named Pipe
[英]How to send messages between c++ .dll and C# app using named pipe?
我正在用C ++編寫注入的.dll,我想使用命名管道與C#app進行通信。
現在,我在C#app中使用內置的System.IO.Pipe .net類,我正在使用C ++中的常規函數。
我沒有太多的C ++經驗(閱讀:這是我的第一個C ++代碼..),我在C#中經驗豐富。
似乎與服務器和客戶端的連接正常,唯一的問題是messaged沒有被發送。 我嘗試將.dll作為服務器,將C#app作為服務器,使管道方向為InOut(雙工),但似乎沒有工作。
當我嘗試將.dll發送到C#應用程序的服務器時,我使用的代碼是這樣的:
DWORD ServerCreate() // function to create the server and wait till it successfully creates it to return.
{
hPipe = CreateNamedPipe(pipename,//The unique pipe name. This string must have the following form: \\.\pipe\pipename
PIPE_ACCESS_DUPLEX,
PIPE_TYPE_MESSAGE | PIPE_READMODE_MESSAGE | PIPE_NOWAIT, //write and read and return right away
PIPE_UNLIMITED_INSTANCES,//The maximum number of instances that can be created for this pipe
4096 , // output time-out
4096 , // input time-out
0,//client time-out
NULL);
if(hPipe== INVALID_HANDLE_VALUE)
{
return 1;//failed
}
else
return 0;//success
}
void SendMsg(string msg)
{
DWORD cbWritten;
WriteFile(hPipe,msg.c_str(), msg.length()+1, &cbWritten,NULL);
}
void ProccesingPipeInstance()
{
while(ServerCreate() == 1)//if failed
{
Sleep(1000);
}
//if created success, wait to connect
ConnectNamedPipe(hPipe, NULL);
for(;;)
{
SendMsg("HI!");
if( ConnectNamedPipe(hPipe, NULL)==0)
if(GetLastError()==ERROR_NO_DATA)
{
DebugPrintA("previous closed,ERROR_NO_DATA");
DisconnectNamedPipe(hPipe);
ConnectNamedPipe(hPipe, NULL);
}
Sleep(1000);
}
和C#cliend像這樣:
static void Main(string[] args)
{
Console.WriteLine("Hello!");
using (var pipe = new NamedPipeClientStream(".", "HyprPipe", PipeDirection.In))
{
Console.WriteLine("Created Client!");
Console.Write("Connecting to pipe server in the .dll ...");
pipe.Connect();
Console.WriteLine("DONE!");
using (var pr = new StreamReader(pipe))
{
string t;
while ((t = pr.ReadLine()) != null)
{
Console.WriteLine("Message: {0}",t);
}
}
}
}
我看到C#客戶端連接到.dll,但它不會收到任何消息..我試着反過來,正如我之前所說,並試圖讓C#發送消息到.dll,會在消息框中顯示它們。 注入了.dll並將其連接到C#服務器,但當它收到一條消息時,它剛剛崩潰了它被注入的應用程序。
請幫助我,或指導我如何在C ++和C#app之間使用命名管道
一些東西。
1-您是否使用相同的管道名稱
C ++:“\\\\。\\ pipe \\ HyperPipe”
C#:“HyperPipe”
2-我認為在C#方面使用ReadToEnd()可能更好,我只在C ++中使用了命名管道,但我認為ReadToEnd()會讀取一條消息,因為你使用的是基於消息的管道。
3-在C ++方面,您正在嘗試使用非阻塞管道並輪詢管道以獲取連接和數據等。我建議使用以下三種方法之一。
a - Use a blocking pipe on a separate thread or b - Use non blocking pipes using Overlapped IO c - Use non blocking pipes using IOCompletion ports
第一個選項是最簡單的,而且聽起來你正在做的事情,它會很好地擴展。 這是一個簡單示例的鏈接到(a) http://msdn.microsoft.com/en-us/library/aa365588 ( VS.85 ) .aspx
4-確保您的編碼在兩側都匹配。 例如,如果您的C ++代碼是為Unicode編譯的,則必須使用Unicode編碼在C#端讀取管道流。 有些事情如下。
using (StreamReader rdr = new StreamReader(pipe, System.Text.Encoding.Unicode))
{
System.Console.WriteLine(rdr.ReadToEnd());
}
更新 :由於我沒有在C#中使用它,我想我會寫一個小測試。 只是使用阻塞管道沒有線程或任何東西,只是為了確認基礎工作,這是非常粗略的測試代碼。
C ++服務器
#include <tchar.h>
#include <windows.h>
int _tmain(int argc, _TCHAR* argv[])
{
HANDLE hPipe = ::CreateNamedPipe(_T("\\\\.\\pipe\\HyperPipe"),
PIPE_ACCESS_DUPLEX,
PIPE_TYPE_MESSAGE | PIPE_READMODE_MESSAGE,
PIPE_UNLIMITED_INSTANCES,
4096,
4096,
0,
NULL);
ConnectNamedPipe(hPipe, NULL);
LPTSTR data = _T("Hello");
DWORD bytesWritten = 0;
WriteFile(hPipe, data, _tcslen(data) * sizeof(TCHAR), &bytesWritten, NULL);
CloseHandle(hPipe);
return 0;
}
C#客戶端
using System;
using System.Text;
using System.IO;
using System.IO.Pipes;
namespace CSPipe
{
class Program
{
static void Main(string[] args)
{
NamedPipeClientStream pipe = new NamedPipeClientStream(".", "HyperPipe", PipeDirection.InOut);
pipe.Connect();
using (StreamReader rdr = new StreamReader(pipe, Encoding.Unicode))
{
System.Console.WriteLine(rdr.ReadToEnd());
}
Console.ReadKey();
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.