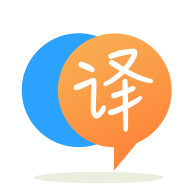
[英]Difference between Convert.ToString(str) and str?.ToString() ?? “”
[英]Difference between Convert.ToString() and .ToString()
Convert.ToString()
和.ToString()
有什么區別?
我在網上發現了很多不同之處,但主要區別是什么?
Convert.ToString()
處理null
,而ToString()
沒有。
在 object 上調用ToString()
假定 object 不是 null(因為 ZA8CFDE63311 上的一個實例方法需要調用它)。 Convert.ToString(obj)
doesn't need to presume the object is not null (as it is a static method on the Convert class), but instead will return String.Empty
if it is null.
除了有關處理null
值的其他答案之外, Convert.ToString
在調用基本Object.ToString
之前嘗試使用IFormattable
和IConvertible
接口。
例子:
class FormattableType : IFormattable
{
private double value = 0.42;
public string ToString(string format, IFormatProvider formatProvider)
{
if (formatProvider == null)
{
// ... using some IOC-containers
// ... or using CultureInfo.CurrentCulture / Thread.CurrentThread.CurrentCulture
formatProvider = CultureInfo.InvariantCulture;
}
// ... doing things with format
return value.ToString(formatProvider);
}
public override string ToString()
{
return value.ToString();
}
}
結果:
Convert.ToString(new FormattableType()); // 0.42
new FormattableType().ToString(); // 0,42
讓我們通過這個例子來理解區別:
int i= 0;
MessageBox.Show(i.ToString());
MessageBox.Show(Convert.ToString(i));
我們可以使用i.ToString ()
或Convert.ToString
轉換 integer i
。 那么有什么區別呢?
它們之間的基本區別是Convert
function 處理 NULLS 而i.ToString ()
不處理; 它將拋出 NULL 引用異常錯誤。 因此,使用convert
的良好編碼習慣始終是安全的。
您可以創建一個 class 並覆蓋toString
方法來做任何你想做的事情。
例如,您可以創建 class “MyMail”並覆蓋toString
方法以發送 email 或執行其他操作,而不是寫入當前的 object。
Convert.toString
將指定的值轉換為其等效的字符串表示形式。
除了處理null之外,這些方法“基本”相同。
Pen pen = null;
Convert.ToString(pen); // No exception thrown
pen.ToString(); // Throws NullReferenceException
來自 MSDN:
Convert.ToString 方法
將指定的值轉換為其等效的字符串表示形式。
返回表示當前 object 的字符串。
object o=null;
string s;
s=o.toString();
//returns a null reference exception for string s.
string str=convert.tostring(o);
//returns an empty string for string str and does not throw an exception.,it's
//better to use convert.tostring() for good coding
我同意@Ryan的回答。 順便說一句,從 C#6.0 開始,您可以使用:
someString?.ToString() ?? string.Empty;
或者
$"{someString}"; // I do not recommend this approach, although this is the most concise option.
代替
Convert.ToString(someString);
對於代碼愛好者來說,這是最好的答案。
.............. Un Safe code ...................................
Try
' In this code we will get "Object reference not set to an instance of an object." exception
Dim a As Object
a = Nothing
a.ToString()
Catch ex As NullReferenceException
Response.Write(ex.Message)
End Try
'............... it is a safe code..............................
Dim b As Object
b = Nothing
Convert.ToString(b)
Convert.ToString(strName)
將處理可為空的值,而strName.Tostring()
將不處理 null 值並引發異常。
所以最好使用Convert.ToString()
然后.ToString();
在Convert.ToString()
中,Convert 處理NULL
值或不處理,但在.ToString()
中,它不處理NULL
值和NULL
引用異常。 因此,使用Convert.ToString()
是一種很好的做法。
ToString()
無法處理 null 值,而convert.ToString()
可以處理 null 的值,因此當您希望系統處理 null 值時,請使用convert.ToString()
ToString() Vs Convert.ToString()
相似之處:-
兩者都用於將特定類型轉換為字符串,即 int 到 string、float 到 string 或 object 到 string。
區別:-
ToString()
無法處理 null 而如果使用Convert.ToString()
將處理 null 值。
例子:
namespace Marcus
{
class Employee
{
public int Id { get; set; }
public string Name { get; set; }
}
class Startup
{
public static void Main()
{
Employee e = new Employee();
e = null;
string s = e.ToString(); // This will throw an null exception
s = Convert.ToString(e); // This will throw null exception but it will be automatically handled by Convert.ToString() and exception will not be shown on command window.
}
}
}
這里兩種方法都用於轉換字符串,但它們之間的基本區別是: Convert
function 處理NULL
,而i.ToString()
沒有它會拋出NULL reference exception error.
因此,使用convert
的良好編碼習慣始終是安全的。
讓我們看一個例子:
string s;
object o = null;
s = o.ToString();
//returns a null reference exception for s.
string s;
object o = null;
s = Convert.ToString(o);
//returns an empty string for s and does not throw an exception.
Convert.ToString(value)
首先嘗試將 obj 轉換為IConvertible ,然后IFormattable調用相應的ToString(...)
方法。 如果參數值為null
則返回string.Empty
。 作為最后的手段,如果沒有其他方法,請返回obj.ToString()
。
值得注意的是, Convert.ToString(value)
可以返回null
,例如value.ToString()
返回 null。
請參閱.Net 參考源
我寫了這段代碼並編譯它。
class Program
{
static void Main(string[] args)
{
int a = 1;
Console.WriteLine(a.ToString());
Console.WriteLine(Convert.ToString(a));
}
}
通過使用“逆向工程”( ilspy ),我發現“object.ToString()”和“Convert.ToString(obj)”只做一件事。 事實上 'Convert.ToString(obj)' 調用 'object.ToString()' 所以'object.ToString()' 更快。
class System.Object
{
public string ToString(IFormatProvider provider)
{
return Number.FormatInt32(this, null, NumberFormatInfo.GetInstance(provider));
}
}
class System.Convert
{
public static string ToString(object value)
{
return value.ToString(CultureInfo.CurrentCulture);
}
}
Convert.Tostring() function 處理 NULL 而 .ToString() 方法不處理。 訪問這里。
在 C# 中,如果您聲明一個字符串變量並且您沒有為該變量分配任何值,則默認情況下該變量采用 null 值。 在這種情況下,如果您使用 ToString() 方法,那么您的程序將拋出 null 引用異常。 另一方面,如果您使用 Convert.ToString() 方法,那么您的程序將不會引發異常。
Convert.Tostring()
基本上只是調用以下value == null? String.Empty: value.ToString()
value == null? String.Empty: value.ToString()
(string)variable
僅在您要轉換的內容上有隱式或顯式運算符時才會轉換
ToString()
可以被類型覆蓋(它可以控制它的作用),如果不是,它會導致類型的名稱
顯然如果一個 object 是null ,你不能訪問實例成員ToString()
,會導致異常
對於可為空類型ToString()
實際上處理null
值:
int? i = null;
var s1 = i.ToString(); // returns ""
var s2 = Convert.ToString(i); // returns ""
只是為了補救現有的答案。
來源:
https://docs.microsoft.com/en-us/dotnet/api/system.nullable-1.tostring?view=net-5.0
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.