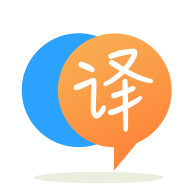
[英]Importing Excel files with a large number of columns header into mysql with c#
[英]Importing excel files in c#
誰能建議我如何在斷開模式下使用ado.net讀取c#中的excel文件。我的excel文件很大,無法保存在內存中。請提出一種將數據加載到數據集中的方法。 現在我正在通過使用Excel = Microsoft.Office.Interop.Excel並添加excel reference(com)來閱讀它們,然后使用諸如range等對象。
可能這就是您正在尋找的http://exceldatareader.codeplex.com/
id說用ADO連接到它並像對待數據庫一樣對待它: http : //www.connectionstrings.com/excel
這是我正在使用的示例,它對於其他想要從Excel電子表格中獲取數據的人來說可能很方便。
DataSet
的DataTable
中。 using System; using System.Data; using System.IO; using System.Runtime.InteropServices; using Excel = Microsoft.Office.Interop.Excel; public class clsExcelWriter : IDisposable { private Excel.Application oExcel; private Excel._Workbook oBook; private Excel._Worksheet oSheet; // Used to store the name of the current file public string FileName { get; private set; } public clsExcelWriter(string filename) { // Initialize Excel oExcel = new Excel.Application(); if (!File.Exists(filename)) { // Create a new one? } else { oBook = (Excel._Workbook)oExcel.Workbooks.Open(filename); oSheet = (Excel._Worksheet)oBook.ActiveSheet; } this.FileName = filename; // Supress any alerts oExcel.DisplayAlerts = false; } private string GetExcelColumnName(int columnNumber) { int dividend = columnNumber; string columnName = String.Empty; int modulo; while (dividend > 0) { modulo = (dividend - 1) % 26; columnName = Convert.ToChar(65 + modulo).ToString() + columnName; dividend = (int)((dividend - modulo) / 26); } return columnName; } public void Dispose() { // Lets make sure we release those COM objects! if (oExcel != null) { Marshal.FinalReleaseComObject(oSheet); oBook.Close(); Marshal.FinalReleaseComObject(oBook); oExcel.Quit(); Marshal.FinalReleaseComObject(oExcel); GC.Collect(); GC.WaitForPendingFinalizers(); GC.Collect(); GC.WaitForPendingFinalizers(); } } public static DataSet OpenFile(string filename) { DataSet ds = new DataSet(); using (clsExcelWriter xl = new clsExcelWriter(filename)) { // Iterate through each worksheet foreach (Excel._Worksheet sheet in xl.oBook.Worksheets) { // Create a new table using the sheets name DataTable dt = new DataTable(sheet.Name); // Get the first row (where the headers should be located) object[,] xlValues = (object[,])sheet.get_Range("A1", xl.GetExcelColumnName(sheet.UsedRange.Columns.Count) + 1).Value; // Iterate through the values to add new DataColumns to the DataTable for (int i = 0; i < xlValues.GetLength(1); i++) { dt.Columns.Add(new DataColumn(xlValues[1, i + 1].ToString())); } // Now get the rest of the rows xlValues = (object[,])sheet.get_Range("A2", xl.GetExcelColumnName(sheet.UsedRange.Columns.Count) + sheet.UsedRange.Rows.Count).Value; for (int row = 0; row < xlValues.GetLength(0); row++) { DataRow dr = dt.NewRow(); for (int col = 0; col < xlValues.GetLength(1); col++) { // xlValues array starts from 1, NOT 0 (just to confuse yee) dr[dt.Columns[col].ColumnName] = xlValues[row + 1, col + 1]; } dt.Rows.Add(dr); } ds.Tables.Add(dt); } } // Your DataSet should now be filled! :) return ds; } }
}
using System; using System.Data; using System.IO; using System.Runtime.InteropServices; using Excel = Microsoft.Office.Interop.Excel; public class clsExcelWriter : IDisposable { private Excel.Application oExcel; private Excel._Workbook oBook; private Excel._Worksheet oSheet; // Used to store the name of the current file public string FileName { get; private set; } public clsExcelWriter(string filename) { // Initialize Excel oExcel = new Excel.Application(); if (!File.Exists(filename)) { // Create a new one? } else { oBook = (Excel._Workbook)oExcel.Workbooks.Open(filename); oSheet = (Excel._Worksheet)oBook.ActiveSheet; } this.FileName = filename; // Supress any alerts oExcel.DisplayAlerts = false; } private string GetExcelColumnName(int columnNumber) { int dividend = columnNumber; string columnName = String.Empty; int modulo; while (dividend > 0) { modulo = (dividend - 1) % 26; columnName = Convert.ToChar(65 + modulo).ToString() + columnName; dividend = (int)((dividend - modulo) / 26); } return columnName; } public void Dispose() { // Lets make sure we release those COM objects! if (oExcel != null) { Marshal.FinalReleaseComObject(oSheet); oBook.Close(); Marshal.FinalReleaseComObject(oBook); oExcel.Quit(); Marshal.FinalReleaseComObject(oExcel); GC.Collect(); GC.WaitForPendingFinalizers(); GC.Collect(); GC.WaitForPendingFinalizers(); } } public static DataSet OpenFile(string filename) { DataSet ds = new DataSet(); using (clsExcelWriter xl = new clsExcelWriter(filename)) { // Iterate through each worksheet foreach (Excel._Worksheet sheet in xl.oBook.Worksheets) { // Create a new table using the sheets name DataTable dt = new DataTable(sheet.Name); // Get the first row (where the headers should be located) object[,] xlValues = (object[,])sheet.get_Range("A1", xl.GetExcelColumnName(sheet.UsedRange.Columns.Count) + 1).Value; // Iterate through the values to add new DataColumns to the DataTable for (int i = 0; i < xlValues.GetLength(1); i++) { dt.Columns.Add(new DataColumn(xlValues[1, i + 1].ToString())); } // Now get the rest of the rows xlValues = (object[,])sheet.get_Range("A2", xl.GetExcelColumnName(sheet.UsedRange.Columns.Count) + sheet.UsedRange.Rows.Count).Value; for (int row = 0; row < xlValues.GetLength(0); row++) { DataRow dr = dt.NewRow(); for (int col = 0; col < xlValues.GetLength(1); col++) { // xlValues array starts from 1, NOT 0 (just to confuse yee) dr[dt.Columns[col].ColumnName] = xlValues[row + 1, col + 1]; } dt.Rows.Add(dr); } ds.Tables.Add(dt); } } // Your DataSet should now be filled! :) return ds; } }
}
using System.Data; using ExcelWriter; namespace Test { class Program { static void Main(string[] args) { DataSet ds = clsExcelWriter.OpenFile(@"C:\\Results.xls"); // Do some fancy stuff with the DataSet! xD while (true) ; } } }
這里是我用來從Excel工作表讀取數據的方法:
private DbDataReader ReadExcelSheet(string file, string sheet)
{
string connStr = "Provider=Microsoft.Jet.OLEDB.4.0;Data Source=" + file + ";Extended Properties=Excel 8.0;";
DbProviderFactory factory = DbProviderFactories.GetFactory("System.Data.OleDb");
DbConnection connection = factory.CreateConnection();
connection.ConnectionString = connStr;
DbCommand command = connection.CreateCommand();
string query = BuildSelectQuery(sheet, names_mapping);//you need column names here
command.CommandText = query;
connection.Open();
DbDataReader dr = command.ExecuteReader();
return dr;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.