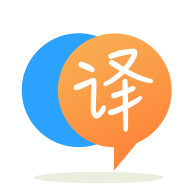
[英]HorizontalAlignment on image not working in PdfPCell in itextsharp
[英]Image auto resizes in PdfPCell with iTextSharp
我對iTextSharp庫中的圖像有一個奇怪的問題。 我正在將圖像添加到PdfPCell中,並且由於某種原因它會被放大。 我如何保持原始尺寸?
我雖然在打印時圖像會相同,但圖片上的差異在打印版本上是相同的。 必須使用ScaleXXX手動縮放圖像以使其向右移動似乎有點不合邏輯並且不會給出好的結果。
那么如何將圖像放在原始大小的表格的PdfPCell中而不必縮放呢?
這是我的代碼:
private PdfPTable CreateTestPDF()
{
PdfPTable table = new PdfPTable(1);
table.WidthPercentage = 100;
Phrase phrase = new Phrase("MY TITLE", _font24Bold);
table.AddCell(phrase);
PdfPTable nestedTable = new PdfPTable(5);
table.WidthPercentage = 100;
Phrase cellText = new Phrase("cell 1", _font9BoldBlack);
nestedTable.AddCell(cellText);
cellText = new Phrase("cell 2", _font9BoldBlack);
nestedTable.AddCell(cellText);
cellText = new Phrase("cell 3", _font9BoldBlack);
nestedTable.AddCell(cellText);
iTextSharp.text.Image image = iTextSharp.text.Image.GetInstance(@"d:\MyPic.jpg");
image.Alignment = iTextSharp.text.Image.ALIGN_CENTER;
PdfPCell cell = new PdfPCell(image);
cell.HorizontalAlignment = PdfPCell.ALIGN_MIDDLE;
nestedTable.AddCell(cell);
cellText = new Phrase("cell 5", _font9BoldBlack);
nestedTable.AddCell(cellText);
nestedTable.AddCell("");
string articleInfo = "Test Text";
cellText = new Phrase(articleInfo, _font8Black);
nestedTable.AddCell(cellText);
nestedTable.AddCell("");
nestedTable.AddCell("");
nestedTable.AddCell("");
table.AddCell(nestedTable);
SetBorderSizeForAllCells(table, iTextSharp.text.Rectangle.NO_BORDER);
return table;
}
static BaseColor _textColor = new BaseColor(154, 154, 154);
iTextSharp.text.Font _font8 = new iTextSharp.text.Font(iTextSharp.text.Font.FontFamily.HELVETICA, 8, iTextSharp.text.Font.NORMAL, _textColor);
iTextSharp.text.Font _font8Black = new iTextSharp.text.Font(iTextSharp.text.Font.FontFamily.HELVETICA, 8, iTextSharp.text.Font.NORMAL, BaseColor.BLACK);
iTextSharp.text.Font _font9 = new iTextSharp.text.Font(iTextSharp.text.Font.FontFamily.HELVETICA, 9, iTextSharp.text.Font.NORMAL, _textColor);
iTextSharp.text.Font _font9BoldBlack = new iTextSharp.text.Font(iTextSharp.text.Font.FontFamily.HELVETICA, 9, iTextSharp.text.Font.BOLD, BaseColor.BLACK);
iTextSharp.text.Font _font10 = new iTextSharp.text.Font(iTextSharp.text.Font.FontFamily.HELVETICA, 10, iTextSharp.text.Font.NORMAL, _textColor);
iTextSharp.text.Font _font10Black = new iTextSharp.text.Font(iTextSharp.text.Font.FontFamily.HELVETICA, 10, iTextSharp.text.Font.NORMAL, BaseColor.BLACK);
iTextSharp.text.Font _font10BoldBlack = new iTextSharp.text.Font(iTextSharp.text.Font.FontFamily.HELVETICA, 10, iTextSharp.text.Font.BOLD, BaseColor.BLACK);
iTextSharp.text.Font _font24Bold = new iTextSharp.text.Font(iTextSharp.text.Font.FontFamily.HELVETICA, 24, iTextSharp.text.Font.BOLD, _textColor);
我正在使用iTextSharp v4.1.2,我得到以下行為:
使用此代碼,通過AddCell方法將圖像直接添加到表中,圖像將按比例放大以適合單元格:
nestedTable.AddCell(image);
使用此代碼,將圖像添加到單元格,然后將單元格添加到表格中,圖像將以其原始大小顯示:
PdfPCell cell = new PdfPCell(image);
cell.HorizontalAlignment = PdfPCell.ALIGN_CENTER;
nestedTable.AddCell(cell);
您是否已將圖像直接添加到pdf文檔(表格外)以比較/仔細檢查圖像大小?
document.add(image);
我假設您希望圖像在單元格中居中,周圍有一些空間。 作為最后的手段,您可以更改圖像。 使其成為具有透明背景的png,並確保圖像的所有邊緣周圍都有一些透明的“邊距”。
編輯
我剛下載了v5.0.2,得到了與上面提到的相同的結果。 我試過它的圖像既小又大於細胞的大小,行為是一樣的; 第一種方法縮放圖像,第二種方法不縮放。
編輯
好吧,顯然我多年來一直錯誤地談到整個DPI的事情。 我似乎無法看到它對圖像的DPI有什么影響。
我以三種不同的分辨率(72dpi,96 dpi和110 dpi)創建了一個600x400px的圖像。 然后我將每個這些圖像添加到一個正好是600x400的新文檔中。
Dim pSize As Rectangle = New Rectangle(600, 1000)
Dim document As Document = New Document(pSize, 0, 0, 0, 0)
對於三個圖像文件中的每一個,當添加到文檔中時
document.add(image)
它們完美地適合文檔,不同的DPI設置沒有區別。
@ Stewbob的答案確實有效,但它只與表格的方法有關。
iTextSharp的問題在於它的行為會有所不同,具體取決於您使用的構造函數。 這將(惱人地)放大圖像以填充單元格:
PdfPCell c = new PdfPCell();
c.Add(image);
c.setHorizontalAlignment(Element.ALIGN_CENTER); // this will be ignored
但這會使圖像保持您設置的大小(並允許對齊):
PdfPCell c = new PdfPCell(image);
c.setHorizontalAlignment(Element.ALIGN_CENTER);
我不確切地知道為什么會這樣,如果你在構造函數中添加圖像而不是'復合模式',如果你稍后添加它(這種情況下每個對象都是假設的話)照顧它自己的對齊方式)。
更多信息(在Java中,但仍然適用) http://tutorials.jenkov.com/java-itext/table.html#cell-modes
因此,如果您必須保留PdfPCell中圖像的大小,您可以使用以下代碼:
iTextSharp.text.Image image = iTextSharp.text.Image.GetInstance(imageFilePath);
// Save the image width
float width = image.Width;
PdfPCell cell = new PdfPCell();
cell.AddElement(image);
// Now find the Image element in the cell and resize it
foreach (IElement element in cell.CompositeElements)
{
// The inserted image is stored in a PdfPTable, so when you find
// the table element just set the table width with the image width, and lock it.
PdfPTable tblImg = element as PdfPTable;
if (tblImg != null)
{
tblImg.TotalWidth = width;
tblImg.LockedWidth = true;
}
}
該函數具有適合圖像的屬性。 只添加一個真實的
cell.AddElement(image,true);
對於那些要求過載的人,請使用:
var imageCell = new PdfPCell(image, true);
代替 :
cell.AddElement(image,true);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.