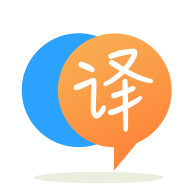
[英]Regular expression to check if password is "8 characters including 1 uppercase letter, 1 special character, alphanumeric characters"
[英]Check Password contains alphanumeric and special character
如何檢查字符串passwordText是否至少包含
嘗試這個:
bool result =
passwordText.Any(c => char.IsLetter(c)) &&
passwordText.Any(c => char.IsDigit(c)) &&
passwordText.Any(c => char.IsSymbol(c));
雖然您可能希望通過“字母字符”,“數字”和“符號”更具體地說明您的意思,因為這些術語對不同的人意味着不同的東西,並且您不確定這些術語的定義是否符合定義框架使用。
我猜你用字母表示“az”或“A-Z”,用數字表示“0-9”,用符號表示任何其他可打印的ASCII字符。 如果是這樣,試試這個:
static bool IsLetter(char c)
{
return (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z');
}
static bool IsDigit(char c)
{
return c >= '0' && c <= '9';
}
static bool IsSymbol(char c)
{
return c > 32 && c < 127 && !IsDigit(c) && !IsLetter(c);
}
static bool IsValidPassword(string password)
{
return
password.Any(c => IsLetter(c)) &&
password.Any(c => IsDigit(c)) &&
password.Any(c => IsSymbol(c));
}
如果實際上你的意思是其他東西,那么相應地調整上述方法。
使用RegEx將是最靈活的。 您始終可以將其存儲在資源文件中,並在不進行代碼更改的情況下進行更改。
有關密碼示例,請參閱以下內容;
您可以使用String.IndexOfAny()
來確定字符串中是否存在指定字符數組中的至少一個字符。
strPassword.IndexOfAny(new char['!','@','#','$','%','^','&','*','(',')']);
您可以使用您正在尋找的字符/符號數組來運行它。 如果它返回大於-1的任何值,那么你就得到了一個匹配。 數字也可以這樣做。
這將允許您准確定義您要查找的內容,並且如果您願意,可以輕松地排除某些字符。
這很簡單;
Regex sampleRegex = new Regex(@"(?!^[0-9]*$)(?!^[a-zA-Z]*$)^([a-zA-Z0-9]{2,})$");
boolean isStrongPassword= sampleRegex.IsMatch(givenPassword);
對於非常簡單的事情,我從之前使用的程序中獲取了這個。
//password rules
int minUpper = 3;
int minLower = 3;
int minLength = 8;
int maxLength = 12;
string allowedSpecials = "@#/.!')";
//entered password
string enteredPassword = "TEStpass123@";
//get individual characters
char[] characters = enteredPassword.ToCharArray();
//checking variables
int upper = 0;
int lower = 0;
int character = 0;
int number = 0;
int length = enteredPassword.Length;
int illegalCharacters = 0;
//check the entered password
foreach (char enteredCharacters in characters)
{
if (char.IsUpper(enteredCharacters))
{
upper = upper + 1;
}
else if (char.IsLower(enteredCharacters))
{
lower = lower + 1;
}
else if (char.IsNumber(enteredCharacters))
{
number = number + 1;
}
else if (allowedSpecials.Contains(enteredCharacters.ToString()))
{
character = character + 1;
}
else
{
illegalCharacters = illegalCharacters + 1;
}
// MessageBox.Show(chars.ToString());
}
if (upper < minUpper || lower < minLower || length < minLength || length > maxLength || illegalCharacters >=1)
{
MessageBox.Show("Something's not right, your password does not meet the minimum security criteria");
}
else
{
//code to proceed this step
}
這應該符合您的所有要求。 它不使用正則表達式。
private bool TestPassword(string passwordText, int minimumLength=5, int maximumLength=12,int minimumNumbers=1, int minimumSpecialCharacters=1) {
//Assumes that special characters are anything except upper and lower case letters and digits
//Assumes that ASCII is being used (not suitable for many languages)
int letters = 0;
int digits = 0;
int specialCharacters = 0;
//Make sure there are enough total characters
if (passwordText.Length < minimumLength)
{
ShowError("You must have at least " + minimumLength + " characters in your password.");
return false;
}
//Make sure there are enough total characters
if (passwordText.Length > maximumLength)
{
ShowError("You must have no more than " + maximumLength + " characters in your password.");
return false;
}
foreach (var ch in passwordText)
{
if (char.IsLetter(ch)) letters++; //increment letters
if (char.IsDigit(ch)) digits++; //increment digits
//Test for only letters and numbers...
if (!((ch > 47 && ch < 58) || (ch > 64 && ch < 91) || (ch > 96 && ch < 123)))
{
specialCharacters++;
}
}
//Make sure there are enough digits
if (digits < minimumNumbers)
{
ShowError("You must have at least " + minimumNumbers + " numbers in your password.");
return false;
}
//Make sure there are enough special characters -- !(a-zA-Z0-9)
if (specialCharacters < minimumSpecialCharacters)
{
ShowError("You must have at least " + minimumSpecialCharacters + " special characters (like @,$,%,#) in your password.");
return false;
}
return true;
}
private void ShowError(string errorMessage) {
Console.WriteLine(errorMessage);
}
這是如何調用它的示例:
private void txtPassword_TextChanged(object sender, EventArgs e)
{
bool temp = TestPassword(txtPassword.Text, 6, 10, 2, 1);
}
這不測試大寫和小寫字母的混合,這可能是優選的。 為此,只需將char條件中斷(ch > 96 && ch < 123)
為小寫字母組(ch > 64 && ch < 91)
為大寫。
正則表達式更短更簡單(適合那些使用它的人)並且在線有很多例子,但這種方法更適合為用戶定制反饋。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.