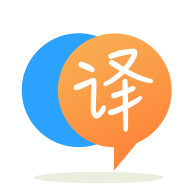
[英]The process cannot access the file .exe because it is being used by another process
[英]The process cannot access the file because it is being used by another process
當我執行以下代碼時,我得到一個常見的異常: The process cannot access the file *filePath* because it is being used by another process
。
允許此線程等待直到可以安全訪問此文件的最有效方法是什么?
假設:
:
using (var fs = File.Open(filePath, FileMode.Append)) //Exception here
{
using (var sw = new StreamWriter(fs))
{
sw.WriteLine(text);
}
}
到目前為止,我想出的最好的方法是以下方法。 這樣做有不利之處嗎?
private static void WriteToFile(string filePath, string text, int retries)
{
const int maxRetries = 10;
try
{
using (var fs = File.Open(filePath, FileMode.Append))
{
using (var sw = new StreamWriter(fs))
{
sw.WriteLine(text);
}
}
}
catch (IOException)
{
if (retries < maxRetries)
{
Thread.Sleep(1);
WriteToFile(filePath, text, retries + 1);
}
else
{
throw new Exception("Max retries reached.");
}
}
}
如果有多個線程嘗試訪問同一文件,請考慮使用鎖定機制。 最簡單的形式可能是:
lock(someSharedObject)
{
using (var fs = File.Open(filePath, FileMode.Append)) //Exception here
{
using (var sw = new StreamWriter(fs))
{
sw.WriteLine(text);
}
}
}
作為替代方案,請考慮:
File.AppendText(text);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.