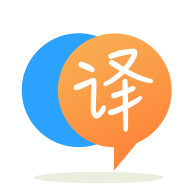
[英]c# how to get list of derived class properties by reflection, ordered by base class properties first, and then derived class props
[英]C# Reflection: How to get the properties of the derived class from the base class
基本上,我只想從基類中使用[Test]屬性獲取派生類的屬性。
這是我的示例代碼:
namespace TestConsole
{
public class BaseClass
{
private System.Int64 _id;
public BaseClass()
{ }
[Test]
public System.Int64 ID
{
get { return _id;}
set { _id = value;}
}
public System.Xml.XmlNode ToXML()
{
System.Xml.XmlNode xml = null;
//Process XML here
return xml;
}
}
public class DerivedClass : BaseClass
{
System.String _name;
public DerivedClass()
{ }
[Test]
public System.String Name
{
get { return _name; }
set { _name = value; }
}
}
public class TestConsole
{
public static void main()
{
DerivedClass derivedClass = new DerivedClass();
System.Xml.XmlNode xmlNode = derivedClass.ToXML();
}
}
}
我希望xmlNode是這樣的:
<root>
<class name="DerivedClass">
<Field name="Id">
<Field name="Name">
</class>
</root>
謝謝
您可以調用this.GetType().GetProperties()
並在每個PropertyInfo
上調用GetCustomAttributes()
來查找具有該屬性的屬性。
您將需要遞歸運行循環以掃描基本類型的屬性。
我認為XmlNode
不適合用於此目的。 首先, 創建XmlNode要求您為其傳遞XmlDocument,這不是一個很好的解決方案 。
其次,可以使用LINQ很好地提取屬性和屬性值,而且我認為這可以與LINQ to XML(特別是XElement
類)很好地集成在一起。
我寫了一些做到這一點的代碼:
using System;
using System.Linq;
using System.Xml.Linq;
[AttributeUsage(AttributeTargets.Property)]
class PropertyAttribute : Attribute { }
class BaseClass
{
[Property]
public int Id { get; set; }
IEnumerable<XElement> PropertyValues {
get {
return from prop in GetType().GetProperties(BindingFlags.Instance | BindingFlags.Public)
where prop.GetGetMethod () != null
let attr = prop.GetCustomAttributes(typeof(PropertyAttribute), false)
.OfType<PropertyAttribute>()
.SingleOrDefault()
where attr != null
let value = Convert.ToString (prop.GetValue(this, null))
select new XElement ("field",
new XAttribute ("name", prop.Name),
new XAttribute ("value", value ?? "null")
);
}
}
public XElement ToXml ()
{
return new XElement ("class",
new XAttribute ("name", GetType ().Name),
PropertyValues
);
}
}
class DerivedClass : BaseClass
{
[Property]
public string Name { get; set; }
}
public static class Program
{
public static void Main()
{
var instance = new DerivedClass { Id = 42, Name = "Johnny" };
Console.WriteLine (instance.ToXml ());
}
}
這會將以下XML輸出到控制台:
<class name="DerivedClass">
<field name="Name" value="Johnny" />
<field name="Id" value="42" />
</class>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.