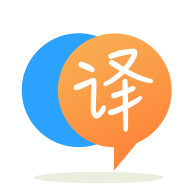
[英]C# - OracleCommandBuilder to generate insert command from a stored proc returning refcursor
[英]Database Programming in C#, returning output from Stored Proc
我正在努力了解如何將存儲過程與應用程序接口。 我的示例很簡單,但是它不在命令提示符下顯示我的列和行,而是顯示System.Data.SqlClient.SqlDataReader。 如何顯示存儲過程中的行?
----Stored Proc--
ALTER PROCEDURE dbo.SelectID
AS
SELECT * FROM tb_User;
-----
下面是代碼:
using System;
using System.Data.SqlClient;
using System.IO;
namespace ExecuteStoredProc
{
class Program
{
static void Main(string[] args)
{
SqlConnection cnnUserMan;
SqlCommand cmmUser;
//SqlDataReader drdUser;
//Instantiate and open the connection
cnnUserMan = new SqlConnection("Data Source=.\\SQLEXPRESS;AttachDbFilename=c:\\Program Files\\Microsoft SQL Server\\MSSQL10.SQLEXPRESS\\MSSQL\\DATA\\UserDB.mdf; Integrated Security=True;Connect Timeout=30;User Instance=True");
cnnUserMan.Open();
//Instantiate and initialize command
cmmUser = new SqlCommand("SelectID", cnnUserMan);
cmmUser.CommandType = System.Data.CommandType.StoredProcedure;
//drdUser = cmmUser.ExecuteReader();
Console.WriteLine(cmmUser.ExecuteReader());
Console.ReadLine();
}
}
}
謝謝。
cmmUser。 ExecuteReader()執行存儲過程並返回SqlDataReader對象。 因此,您需要像這樣使用注釋掉的SqlDataReader:
SqlDataReader drdUser;
drdUser = cmmUser.ExecuteReader();
while(drdUser.Read()){
//You can get at each column in the row by indexing the reader using either the column number
// like drdUser[0] or the column name drdUser["COlumnName"]. Since I don't know the names of your
// columns I will use numbers
Console.WriteLine(String.Format("{0} {1} {2}", drdUser[0], drdUser[1], drdUser[2]);
}
drdUser.Close():
另外,我建議將SqlConnection的實例化包裝到using塊中,以便在完成如下操作后將其處置:
namespace ExecuteStoredProc
{
class Program
{
static void Main(string[] args)
{
//Instantiate and open the connection
using(SqlConnection cnnUserMan = new SqlConnection("Your connection string"))
{
cnnUserMan.Open();
//Instantiate and initialize command
using(SqlCommand cmmUser = new SqlCommand("SelectID", cnnUserMan))
{
cmmUser.CommandType = System.Data.CommandType.StoredProcedure;
using(SqlDataReader drdUser = cmmUser.ExecuteReader())
{
while(drdUser.Read())
{
Console.WriteLine(String.Format("{0} {1} {2}", drdUser[0], drdUser[1], drdUser[2]);
}
}
}
Console.ReadLine();
}
}
}
}
UPDATE
根據marc_s的評論,將所有一次性組件,SqlConnection,SqlCommand和SqlDataReader封裝在using塊中以確保它們被正確處理是有意義的,而不是我原來的解決方案,該解決方案僅使用use包裹SqlConnection。
更新2
根據Thorarin的評論,將變量聲明為using塊的一部分看起來更干凈,尤其是在這種情況下,您不需要每個using塊之外的變量。
此代碼將顯示存儲過程返回的所有行:
static void Main(string[] args)
{
// Instantiate the connection
using (SqlConnection cnnUserMan = new SqlConnection("Data Source=.\\SQLEXPRESS;AttachDbFilename=c:\\Program Files\\Microsoft SQL Server\\MSSQL10.SQLEXPRESS\\MSSQL\\DATA\\UserDB.mdf; Integrated Security=True;Connect Timeout=30;User Instance=True"))
using (SqlCommand cmmUser = cnnUserMan.CreateCommand())
{
// Initialize command
cmmUser.CommandText = "SelectID";
cmmUser.CommandType = CommandType.StoredProcedure;
cnnUserMan.Open();
using (SqlDataReader dr = cmmUser.ExecuteReader())
{
// Loop through returned rows
while (dr.Read())
{
// Loop through all the returned columns
// Printing column name and value
for (int col = 0; col < dr.FieldCount; col++)
{
Console.WriteLine(dr.GetName(col) + " = " + dr.GetValue(col));
}
Console.WriteLine();
}
}
}
Console.ReadLine();
}
我做了一些更改。 您可能會注意到using
語句的使用,該語句可確保正確處理SqlConnection
和SqlDataReader
對象。 完成后,這將釋放與數據庫的連接。
閱讀信息有多種方法,因此請查看MSDN上的SqlDataReader文檔 。 或者,您可能想使用更通用的IDataReader
接口。
更新 :
還為SqlCommand
添加了using
語句,現在使用CreateCommand
實例化該語句。 現在在打開連接之前創建命令。
您不能使用Console.WriteLine來顯示DataTable,因為您必須使用Foreach / for循環遍歷表的每一行並逐一打印。
您將需要通過迭代ExecuteReader方法返回的DataReader並逐列和逐行構建輸出來手動生成輸出字符串。 您可以創建一個通用類來為任何數據讀取器輸出執行此操作。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.