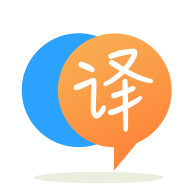
[英]Check if List<T> element contains an item with a Particular Property Value
[英]how to check if List<T> element contains an item with a Particular Property Value
public class PricePublicModel
{
public PricePublicModel() { }
public int PriceGroupID { get; set; }
public double Size { get; set; }
public double Size2 { get; set; }
public int[] PrintType { get; set; }
public double[] Price { get; set; }
}
List<PricePublicModel> pricePublicList = new List<PricePublicModel>();
如何檢查pricePublicList
元素pricePublicList
包含特定值。 更准確地說,我想檢查是否存在pricePublicModel.Size == 200
? 另外,如果這個元素存在,如何知道它是哪一個?
編輯如果字典更適合這個,那么我可以使用字典,但我需要知道如何:)
如果您有一個列表,並且想知道列表中的哪個位置存在與給定條件匹配的元素,則可以使用FindIndex
實例方法。 比如
int index = list.FindIndex(f => f.Bar == 17);
其中f => f.Bar == 17
是具有匹配條件的謂詞。
在你的情況下,你可能會寫
int index = pricePublicList.FindIndex(item => item.Size == 200);
if (index >= 0)
{
// element exists, do what you need
}
bool contains = pricePublicList.Any(p => p.Size == 200);
您可以使用現有的
if (pricePublicList.Exists(x => x.Size == 200))
{
//code
}
使用 LINQ 很容易做到這一點:
var match = pricePublicList.FirstOrDefault(p => p.Size == 200);
if (match == null)
{
// Element doesn't exist
}
您實際上並不需要 LINQ,因為List<T>
提供了一種完全符合您要求的方法: Find
。
搜索與指定謂詞定義的條件匹配的元素,並返回整個
List<T>
第一次出現的元素。
示例代碼:
PricePublicModel result = pricePublicList.Find(x => x.Size == 200);
var item = pricePublicList.FirstOrDefault(x => x.Size == 200);
if (item != null) {
// There exists one with size 200 and is stored in item now
}
else {
// There is no PricePublicModel with size 200
}
您也可以只使用List.Find()
:
if(pricePublicList.Find(item => item.Size == 200) != null) { // Item exists, do something } else { // Item does not exist, do something else }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.