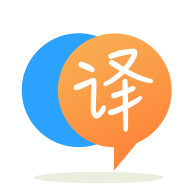
[英]How to generate .NET 4.0 classes from xsd with more than 1 element to class?
[英]How to generate .NET 4.0 classes from xsd?
使用 Visual Studio 2010 從 xsd 文件生成 .NET 4.0 c# 類(實體)的選項有哪些?
足夠簡單; 只需運行(在 vs 命令提示符下)
xsd your.xsd /classes
(這將創建your.cs
)。 但是請注意,這里的大多數內在選項自 2.0 以來沒有太大變化
對於選項,使用xsd /?
或查看 MSDN ; 例如/enableDataBinding
可能很有用。
我在這里向您展示使用 Vs2017 和 Vs2019 的最簡單方法 使用 Visual Studio 打開您的 xsd 並生成一個示例 xml 文件,如建議的url 中所示。
2. 在“XML Schema Explorer”中一直向下滾動以找到根/數據節點。 右鍵單擊根/數據節點,它將顯示“生成示例 XML”。 如果未顯示,則表示您不在數據元素節點上,而是在任何數據定義節點上。
當您有循環引用(即一個類型可以直接或間接擁有它自己類型的元素)時,xsd.exe 不能很好地工作。
當存在循環引用時,我使用 Xsd2Code。 Xsd2Code 可以很好地處理循環引用並且可以在 VS IDE 中工作,這是一個很大的優勢。 它還具有許多您可以使用的功能,例如生成序列化/反序列化代碼。 如果要生成序列化,請確保打開 GenerateXMLAttributes(否則,如果未在所有元素上定義,則會出現排序異常)。
兩者都不適用於選擇功能。 你最終會得到對象的列表/集合,而不是你想要的類型。 如果可能,我建議避免在 xsd 中進行選擇,因為這不會很好地序列化/反序列化為強類型類。 不過,如果你不關心這個,那么這不是問題。
xsd2code 中的 any 功能反序列化為 System.Xml.XmlElement,我覺得這非常方便,但如果您想要強類型對象,則可能會出現問題。 在允許自定義配置數據時,我經常使用 any,因此 XmlElement 可以方便地傳遞給另一個在別處自定義定義的 XML 反序列化器。
對於快速和懶惰的解決方案,(並且根本不使用 VS)嘗試這些在線轉換器:
XSD => XML => C# 類
示例 XSD:
<?xml version="1.0" encoding="UTF-8" ?>
<xs:schema xmlns:xs="http://www.w3.org/2001/XMLSchema">
<xs:element name="shiporder">
<xs:complexType>
<xs:sequence>
<xs:element name="orderperson" type="xs:string"/>
<xs:element name="shipto">
<xs:complexType>
<xs:sequence>
<xs:element name="name" type="xs:string"/>
<xs:element name="address" type="xs:string"/>
<xs:element name="city" type="xs:string"/>
<xs:element name="country" type="xs:string"/>
</xs:sequence>
</xs:complexType>
</xs:element>
<xs:element name="item" maxOccurs="unbounded">
<xs:complexType>
<xs:sequence>
<xs:element name="title" type="xs:string"/>
<xs:element name="note" type="xs:string" minOccurs="0"/>
<xs:element name="quantity" type="xs:positiveInteger"/>
<xs:element name="price" type="xs:decimal"/>
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:sequence>
<xs:attribute name="orderid" type="xs:string" use="required"/>
</xs:complexType>
</xs:element>
</xs:schema>
轉換為 XML:
<?xml version="1.0" encoding="utf-8"?>
<!-- Created with Liquid Technologies Online Tools 1.0 (https://www.liquid-technologies.com) -->
<shiporder xsi:noNamespaceSchemaLocation="schema.xsd" orderid="string" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<orderperson>string</orderperson>
<shipto>
<name>string</name>
<address>string</address>
<city>string</city>
<country>string</country>
</shipto>
<item>
<title>string</title>
<note>string</note>
<quantity>3229484693</quantity>
<price>-6894.465094196054907</price>
</item>
<item>
<title>string</title>
<note>string</note>
<quantity>2181272155</quantity>
<price>-2645.585094196054907</price>
</item>
<item>
<title>string</title>
<note>string</note>
<quantity>2485046602</quantity>
<price>4023.034905803945093</price>
</item>
<item>
<title>string</title>
<note>string</note>
<quantity>1342091380</quantity>
<price>-810.825094196054907</price>
</item>
</shiporder>
轉換為此類結構:
/*
Licensed under the Apache License, Version 2.0
http://www.apache.org/licenses/LICENSE-2.0
*/
using System;
using System.Xml.Serialization;
using System.Collections.Generic;
namespace Xml2CSharp
{
[XmlRoot(ElementName="shipto")]
public class Shipto {
[XmlElement(ElementName="name")]
public string Name { get; set; }
[XmlElement(ElementName="address")]
public string Address { get; set; }
[XmlElement(ElementName="city")]
public string City { get; set; }
[XmlElement(ElementName="country")]
public string Country { get; set; }
}
[XmlRoot(ElementName="item")]
public class Item {
[XmlElement(ElementName="title")]
public string Title { get; set; }
[XmlElement(ElementName="note")]
public string Note { get; set; }
[XmlElement(ElementName="quantity")]
public string Quantity { get; set; }
[XmlElement(ElementName="price")]
public string Price { get; set; }
}
[XmlRoot(ElementName="shiporder")]
public class Shiporder {
[XmlElement(ElementName="orderperson")]
public string Orderperson { get; set; }
[XmlElement(ElementName="shipto")]
public Shipto Shipto { get; set; }
[XmlElement(ElementName="item")]
public List<Item> Item { get; set; }
[XmlAttribute(AttributeName="noNamespaceSchemaLocation", Namespace="http://www.w3.org/2001/XMLSchema-instance")]
public string NoNamespaceSchemaLocation { get; set; }
[XmlAttribute(AttributeName="orderid")]
public string Orderid { get; set; }
[XmlAttribute(AttributeName="xsi", Namespace="http://www.w3.org/2000/xmlns/")]
public string Xsi { get; set; }
}
}
注意力! 考慮到這只是入門,結果顯然需要細化!
我在批處理腳本中使用XSD
直接從XML
生成.xsd
文件和類:
set XmlFilename=Your__Xml__Here
set WorkingFolder=Your__Xml__Path_Here
set XmlExtension=.xml
set XsdExtension=.xsd
set XSD="C:\Program Files (x86)\Microsoft SDKs\Windows\v8.1A\bin\NETFX 4.5.1\Tools\xsd.exe"
set XmlFilePath=%WorkingFolder%%XmlFilename%%XmlExtension%
set XsdFilePath=%WorkingFolder%%XmlFilename%%XsdExtension%
%XSD% %XmlFilePath% /out:%WorkingFolder%
%XSD% %XsdFilePath% /c /out:%WorkingFolder%
在我的情況下工作的命令是:
xsd /c your.xsd
Marc Gravells 的回答適合我,但我的 xsd 是 .xml 的擴展名。 當我使用 xsd 程序時,它給出了:
- The table (Amt) cannot be the child table to itself in nested relations.
根據此KB325695,我將擴展名從 .xml 重命名為 .xsd 並且運行良好。
我在 Windows 命令提示符下使用了xsd.exe
。
但是,由於我的 xml 引用了幾個在線 xml(在我的情況下http://www.w3.org/1999/xlink.xsd
引用了http://www.w3.org/2001/xml.xsd
)我還必須下載這些原理圖,將它們放在與我的 xsd 相同的目錄中,然后在命令中列出這些文件:
"C:\\Program Files (x86)\\Microsoft SDKs\\Windows\\v8.1A\\bin\\NETFX 4.5.1 Tools\\xsd.exe" /classes /language:CS your.xsd xlink.xsd xml.xsd
除了 WSDL,我還有 xsd 文件。 以上在我的情況下不起作用給出了錯誤。 它的工作方式如下
wsdl /l:C# /out:D:\FileName.cs D:\NameApi\wsdl_1_1\RESAdapterService.wsdl
D:\CXTypes.xsd D:\CTypes.xsd
D:\Preferences.xsd
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.